How to clone a component with its props?
In React, while components themselves are designed to be reusable, there are instances where you might want to generate a copy of a component but with slight alterations to its properties (props). This could be to inject additional props, override existing ones, or encapsulate behavior.
React offers a built-in utility called `React.cloneElement` to achieve this. The `React.cloneElement` function allows you to create a copy of a specific React element (an instance of a component) while preserving its props and adding or overwriting them as required.
The syntax for `React.cloneElement` is quite straightforward. The first argument is the element you want to clone. The second argument allows you to specify new props or override existing ones. Subsequent arguments can be used to pass children explicitly.
For example, if you have a component instance `<MyComponent prop1=”value1″ />` and you want to clone it while adding a new prop (`prop2=”value2″`) and overriding an existing one (`prop1=”newValue1″`), you would do:
```javascript const clonedComponent = React.cloneElement( <MyComponent prop1="value1" />, { prop1: "newValue1", prop2: "value2" } ); ```
This `clonedComponent` would now represent an instance of `MyComponent` with `prop1` set to `”newValue1″` and an additional `prop2` set to `”value2″`.
It’s important to note that `React.cloneElement` does a shallow copy of the element. While the new element will have new props, the original element’s references (like event handlers) remain unchanged. This ensures that the behavior of the component remains consistent even after cloning.
`React.cloneElement` provides a robust and clean way to replicate and modify React components dynamically. It offers developers flexibility in component composition, making it easier to adapt components to diverse contexts without altering the original definition.
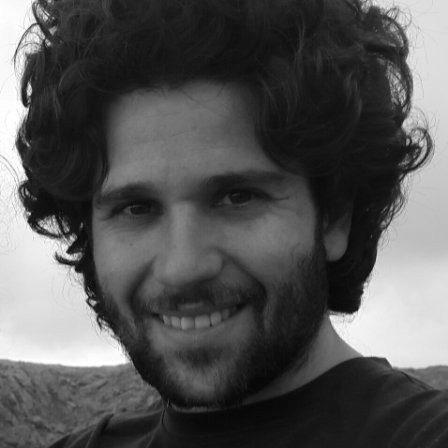
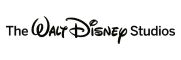