Taking Charge of Component Updates: Using ReactJS’s useForceUpdate Hook for Precise Control in Functional Components
This introduction provides an overview of the blog post on `useForceUpdate` in ReactJS, a function that allows developers to manually trigger a component re-render, circumventing React’s standard state or prop changes based model. The blog will cover the fundamentals of `useForceUpdate`, its applications in the ReactJS framework, and circumstances where its usage becomes necessary. In addition, readers will be guided through the implementation process, informed about best practices, and introduced to potential alternatives. This post is geared towards both beginner and experienced React developers aiming to deepen their understanding of ReactJS component updates.
Understanding React Component Updates
This section explains how React handles component updates. React uses a virtual DOM to manage changes in an application’s interface, comparing the new virtual DOM to the old one when a component’s state or props change. This comparison process, known as “diffing,” informs React whether to update the actual DOM. Therefore, changes in state and props usually trigger component updates. However, in certain scenarios, it may be necessary to force an update even without changes in state or props. This is where `useForceUpdate` comes in. React is also designed to minimize unnecessary renders, which enhances application performance but can cause issues if the render depends on mutable values outside of state or props.
The Need for Force Update
This segment explains why `useForceUpdate` might be needed in React applications. Scenarios include external data changes, bypassing the `shouldComponentUpdate` lifecycle method, and non-reactable state. External data refers to global variables or data from a shared state management system like Redux. The `shouldComponentUpdate` method, if it returns false, prevents a re-render even if state or props change. Non-reactable state involves variables in the component that change but don’t trigger a re-render as they are not part of state or props.
Although these cases may necessitate forced updates, they should be used sparingly as they can cause unpredictable behavior and make debugging harder. React’s philosophy encourages managing components via state and props, hence it’s generally better to encapsulate changing data within state or props to allow natural re-renders.
ReactJS and useForceUpdate Hook
In React, a Hook is a special function that lets you “hook into” React features. For instance, `useState` and `useEffect` are hooks that allow you to add React state and side effects to functional components. The `useForceUpdate` is not a built-in React Hook, but you can implement it using the existing built-in Hooks.
So, what is `useForceUpdate`? It’s a custom hook that forces a re-render of a functional component, even when neither state nor props have changed. While class components have a `forceUpdate()` method that can be used to force a re-render, functional components don’t have a built-in way to do this.
Here’s a basic implementation of `useForceUpdate`:
import { useState } from 'react'; function useForceUpdate(){ const [value, setValue] = useState(0); // integer state return () => setValue(value => value + 1); // update the state to force render }
In the above code, `useForceUpdate` is a function that uses the `useState` Hook to create a piece of state. It utilizes the `useState` hook to create a state that doesn’t carry any significant meaning in the component. Its purpose is to trigger a re-render when updated.
The function returned by `useForceUpdate` is responsible for updating this state, thus forcing the component to re-render. While it’s a powerful tool, `useForceUpdate` should be used sparingly, reserved for specific cases where a re-render is necessary without a state or prop change. In most cases, it’s preferable to allow React to handle updates based on state and prop changes.
Implementing useForceUpdate
Implementing `useForceUpdate` in your functional component is straightforward. Here’s a step-by-step guide:
Step 1: Create a new React functional component.
import React from 'react'; function MyComponent() { // component body }
Step 2: Inside your functional component, define the `useForceUpdate` function.
import React, { useState } from 'react'; function MyComponent() { const useForceUpdate = () => { const [value, setValue] = useState(0); return () => setValue(value => value + 1); } }
Step 3: Call `useForceUpdate` to get the force update function.
import React, { useState } from 'react'; function MyComponent() { const useForceUpdate = () => { const [value, setValue] = useState(0); return () => setValue(value => value + 1); } const forceUpdate = useForceUpdate(); }
Step 4: Now you can call `forceUpdate` anywhere inside your component to force a re-render.
import React, { useState } from 'react'; function MyComponent() { const useForceUpdate = () => { const [value, setValue] = useState(0); return () => setValue(value => value + 1); } const forceUpdate = useForceUpdate(); const handleClick = () => { // Perform actions… forceUpdate(); }; return ( <button onClick={handleClick}> Click me </button> ); } export default MyComponent;
In this example, clicking the button will force `MyComponent` to re-render, even if no state or prop changes have occurred.
Remember that while `useForceUpdate` can be helpful in some situations, you should avoid overusing it. Most of the time, you can and should rely on React’s built-in re-rendering mechanism, which is based on changes to state and props.
Case Study: useForceUpdate in Action
To better understand the `useForceUpdate` hook, let’s explore a practical case study.
Imagine you’re creating a stopwatch application in React. When the stopwatch is running, the application needs to re-render every second to update the displayed time. However, the running time isn’t part of the React component’s state or props—it’s an external value, which makes this a perfect scenario for `useForceUpdate`.
Here is how you might implement this:
import React, { useState, useEffect } from 'react'; function Stopwatch() { const useForceUpdate = () => { const [value, setValue] = useState(0); return () => setValue(value => value + 1); } const forceUpdate = useForceUpdate(); let seconds = 0; let intervalId = null; const startStopwatch = () => { intervalId = setInterval(() => { seconds += 1; forceUpdate(); }, 1000); }; const stopStopwatch = () => { clearInterval(intervalId); }; useEffect(() => { return () => { stopStopwatch(); }; }, []); return ( <div> <p>Elapsed time: {seconds} seconds</p> <button onClick={startStopwatch}>Start</button> <button onClick={stopStopwatch}>Stop</button> </div> ); } export default Stopwatch;
In this example, the `setInterval` function updates the `seconds` variable every second, and `forceUpdate` is called to force a re-render of the Stopwatch component, updating the displayed time.
We use the `useEffect` hook to clean up the interval when the component is unmounted. This is important to prevent memory leaks, as intervals can continue to run even after the component is unmounted, if they’re not properly cleared.
This case study demonstrates a valid use case for `useForceUpdate`. Although the stopwatch scenario could be handled using state, using `forceUpdate` here helps to illustrate how the hook can be used.
Best Practices and Precautions
While `useForceUpdate` can be a useful tool in some situations, it’s important to understand its potential pitfalls and to use it responsibly.
Firstly, it’s important to avoid unnecessary re-renders, as they can negatively impact performance. Secondly, embrace React’s philosophy of reactivity; if frequent force updates are needed, consider restructuring state and props. Thirdly, before resorting to useForceUpdate, explore other solutions like state management libraries (Redux, MobX), the context API, or the useEffect hook. Fourthly, ensure that useForceUpdate usage is compatible with React’s Concurrent Mode to prevent infinite loops.
Lastly, avoid complex dependencies in components which may lead to the need for force updating; encapsulating as much as possible within state and props is advised. While useForceUpdate can be powerful, it should be used responsibly to avoid complications or performance issues.
Conclusion
`useForceUpdate` in ReactJS allows developers to manually trigger updates in functional components, even without changes in state or props. However, it should be used responsibly to avoid performance issues and complex debugging. Adhering to React’s philosophy of managing updates via state and props typically leads to more maintainable and efficient code, but `useForceUpdate` can be useful in certain scenarios like external changes or mutable variables. The goal of this blog post was to deepen understanding of `useForceUpdate` and provide guidelines on its effective use. The hope is that it has been educational and beneficial for all developers, helping to ensure smooth updates in their React applications.
Table of Contents
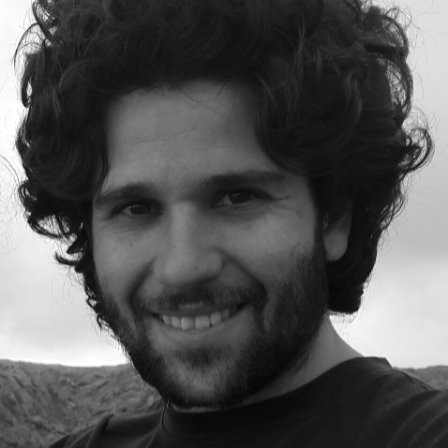
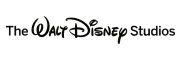