Enhancing ReactJS Functional Components with usePromise Hook
ReactJS, with its powerful composition model and functional programming concepts, has established itself as a leading library for user interface development. These strengths make it a go-to choice for developers and a compelling reason to hire ReactJS developers for your projects. In React version 16.8.0, hooks were introduced, allowing functional components to access features traditionally confined to class components. One such feature is the ability to handle promises.
This article dives into `usePromise`, a custom React hook designed to seamlessly handle promises within functional components. Although a built-in `usePromise` hook doesn’t exist in React’s current offerings (as of my knowledge cutoff in September 2021), the emphasis of this article is to explore how to create and make the most of such a hook. This way, you and your team of ReactJS developers can manage promises more efficiently in your React applications.
Understanding Promises
In JavaScript, a Promise is an object representing the eventual completion or failure of an asynchronous operation. Promises are typically in one of these three states:
- Pending: The Promise’s outcome hasn’t yet been determined because the asynchronous operation is still being processed.
- Fulfilled: The asynchronous operation completed successfully.
- Rejected: The asynchronous operation failed.
Promises significantly simplify asynchronous JavaScript, eliminating the need for nesting callbacks, thereby preventing ‘callback hell’. They are frequently used with AJAX requests, timers, and other asynchronous operations.
The Need for usePromise
Dealing with promises in React, especially within the render method, can be tricky. Rendering is synchronous, but promises are asynchronous. React render method cannot be paused until a promise is resolved or rejected. This dichotomy can lead to unexpected behavior, such as flickering UI or stale data.
This is where a `usePromise` custom hook comes into play. It allows us to handle the promise’s lifecycle – from initiating the promise, handling its pending state, and finally managing its resolution or rejection.
Creating the usePromise Hook
Let’s create a `usePromise` hook. This hook takes a function that returns a promise and returns an array with three items: data, error, and isPending. It uses the `useState` and `useEffect` hooks to manage the state and lifecycle of the promise.
Here is what the basic structure of the `usePromise` hook might look like:
```jsx import { useState, useEffect } from 'react'; const usePromise = (promiseCreator, deps) => { const [isPending, setIsPending] = useState(false); const [data, setData] = useState(null); const [error, setError] = useState(null); useEffect(() => { setIsPending(true); promiseCreator() .then(response => { setData(response); setIsPending(false); }) .catch(err => { setError(err); setIsPending(false); }); }, deps); return [data, error, isPending]; }; export default usePromise; ```
In this hook, `promiseCreator` is a function that returns a promise, and `deps` is an array of dependencies for the `useEffect` hook.
Using the usePromise Hook
Now, let’s see how we can use our custom hook. Suppose we have an API function `fetchUsers` that returns a promise.
```jsx import React from 'react'; import usePromise from './usePromise'; const fetchUsers = () => { return new Promise((resolve, reject) => { // Simulate an API call setTimeout(() => { resolve(['User1', 'User2', 'User3']); }, 2000); }); }; const UserList = () => { const [users, error, isPending] = usePromise(fetchUsers, []); return ( <div> {isPending ? ( 'Loading...' ) : error ? ( `Error: ${error.message}` ) : ( <ul> {users.map(user => ( <li key={user}>{user}</li> ))} </ul> )} </div> ); }; export default UserList; ```
In the `UserList` component, we call the `usePromise` hook with `fetchUsers` as the `promiseCreator`. The `usePromise` hook manages the state and life cycle of the promise, and we use its return values to render the appropriate UI.
Error Handling with usePromise
One of the significant advantages of the `usePromise` hook is error handling. Since the hook catches any errors thrown by the promise, these errors can be handled and displayed within the component.
Consider the following example where the `fetchUsers` function might reject the promise with an error:
```jsx const fetchUsers = () => { return new Promise((resolve, reject) => { // Simulate an API call setTimeout(() => { reject(new Error('Failed to fetch users')); }, 2000); }); }; ```
In this case, the `UserList` component would display the error message ‘Failed to fetch users’ if the promise is rejected.
Conclusion
Leveraging promises in JavaScript is integral to handling asynchronous operations effectively. This is where the `usePromise` custom hook shines, enabling efficient promise management within functional React components, thus simplifying state management and lifecycle methods. If you’re looking to adopt this efficient pattern, consider hiring ReactJS developers skilled in creating and using custom hooks like `usePromise`. They can tailor these abstractions to your specific applications, leading to cleaner, more maintainable codebases.
The `usePromise` hook is a robust tool in the React toolkit that harmonizes the power of promises with React’s component-based model. It is adept at managing asynchronous operations, upholding a responsive UI, and robustly handling errors.
But remember, using the `usePromise` hook judiciously is key. It’s a neat abstraction, but it’s not always the perfect solution for every situation. Hence, hiring ReactJS developers who can accurately identify the specific needs and context of your project to adopt the right tools and patterns is invaluable.
Table of Contents
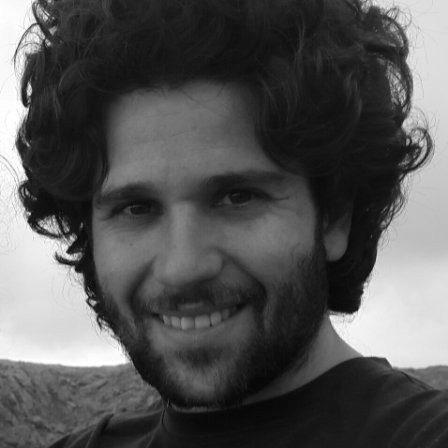
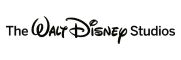