How to conditionally render components?
In React, components represent parts of the user interface. However, not all parts need to be visible all the time. Depending on the application’s state or props passed to a component, you might want to display or hide certain elements. This is where conditional rendering comes into play.
- Using JavaScript Ternary Operator: This is one of the most common ways to conditionally render components. The syntax is concise and integrates seamlessly within JSX:
```javascript {condition ? <ComponentA /> : <ComponentB />} ```
If the condition is true, `ComponentA` will be rendered; otherwise, `ComponentB` will be displayed.
- Logical && Operator: When you only want to render a component when a condition is true (and not render anything otherwise), the `&&` operator becomes handy:
```javascript {condition && <ComponentA />} ```
Here, `ComponentA` will only be rendered if the condition is true.
- Using Variables: You can use JavaScript variables to store elements. This allows for more complex logic using standard `if-else` statements:
```javascript let element; if (condition) { element = <ComponentA />; } else { element = <ComponentB />; } return <div>{element}</div>; ```
- Short-circuit Evaluation: Be cautious when using this approach, especially with values that can be falsy in JavaScript (e.g., `0` or an empty string). Using them directly might not yield the expected results due to type coercion.
- Higher Order Components (HOCs) or Render Props: For more advanced scenarios, especially when conditional rendering logic needs to be reused across multiple components, HOCs or render props might be used. These patterns allow for greater abstraction and reuse of conditional logic.
React’s integration with JavaScript offers various methods to conditionally render components, making it straightforward to create dynamic user interfaces that respond to varying conditions. By understanding and applying these patterns effectively, developers can ensure that users are presented with the right content at the right time.
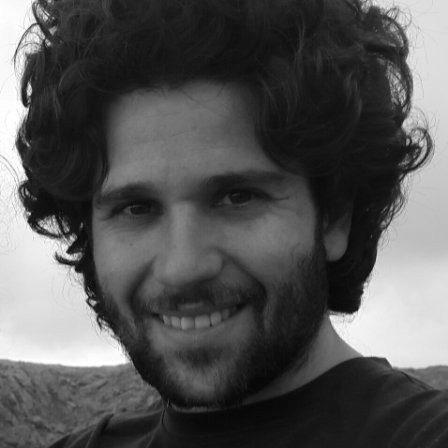
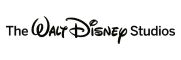