A Guide to Controlled and Uncontrolled Components in ReactJS Forms
Forms are an essential part of any web application that requires user input. ReactJS provides two ways to handle form inputs: controlled and uncontrolled components. Both approaches have their benefits and drawbacks, and choosing the right one depends on the specific requirements of your project.
Table of Contents
1. Controlled Components
A controlled component is a form input element whose value is controlled by React. In other words, the state of the component is managed by React, and any changes to the input value are reflected in the component’s state. To create a controlled component, you need to define a state variable to hold the input value and an event handler to update the state variable whenever the user interacts with the input.
1.1 Example of a controlled component that accepts a user’s name:
javascript
import React, { useState } from 'react'; function ControlledComponentExample() { const [name, setName] = useState(''); const handleNameChange = (event) => { setName(event.target.value); }; return ( <div> <label htmlFor="name">Name:</label> <input type="text" id="name" value={name} onChange={handleNameChange} /> <p>Hello, {name}!</p> </div> ); }
In this example, we use the useState hook to create a state variable called name, which is initially set to an empty string. We define a function called handleNameChange that updates the name state variable whenever the user types a character into the input field. Finally, we render the input field with its value prop set to the name state variable and its onChange prop set to the handleNameChange function.
The main advantage of controlled components is that they provide a consistent way to manage the state of form inputs. By keeping the input value in sync with the component’s state, you can easily implement features like input validation and dynamic input fields.
1.2 Example of a controlled component that uses input validation:
This validation is to ensure that the user’s name is at least two characters long.
javascript
import React, { useState } from 'react'; function ControlledComponentWithValidation() { const [name, setName] = useState(''); const [error, setError] = useState(''); const handleNameChange = (event) => { const newName = event.target.value; setName(newName); if (newName.length < 2) { setError('Name must be at least two characters long'); } else { setError(''); } }; return ( <div> <label htmlFor="name">Name:</label> <input type="text" id="name" value={name} onChange={handleNameChange} /> {error && <p>{error}</p>} <p>Hello, {name}!</p> </div> ); }
In this example, we define a state variable called error to hold any validation errors. In the handleNameChange function, we update the name state variable as before, but we also check if the new name is at least two characters long. If it’s not, we set the error state variable to an error message, which we display below the input field.
2. Uncontrolled Components
An uncontrolled component is a form input element whose value is managed by the browser rather than React. Instead of defining a state variable to hold the input value, you use a ref to get the current value of the input field. This means that the value of the input field is not tied to the component’s state, and any changes to the input field are not reflected in the component’s state.
2.1 Example of an uncontrolled component that accepts a user’s name:
javascript
import React, { useRef } from 'react'; function UncontrolledComponentExample() { const inputRef = useRef(null); const handleSubmit = (event) => { event.preventDefault(); alert(`Name: ${inputRef.current.value}`); }; return ( <form onSubmit={handleSubmit}> <label htmlFor="name">Name:</label> <input type="text" id="name" ref={inputRef} /> <button type="submit">Submit</button> </form> ); }
In this example, we use the useRef hook to create a reference to the input field. We define a function called handleSubmit that gets called when the user submits the form. Inside the function, we use the inputRef to get the current value of the input field and display it in an alert box.
The main advantage of uncontrolled components is that they provide a simpler way to handle form inputs when you don’t need to manage the input value in React’s state. They can also be useful when working with third-party libraries that require uncontrolled input fields.
2.2 Example of an uncontrolled component using an HTML form to submit data:
javascript
import React from 'react'; function UncontrolledFormExample() { const handleSubmit = (event) => { event.preventDefault(); const formData = new FormData(event.target); fetch('/api/submit', { method: 'POST', body: formData }) .then((response) => response.json()) .then((data) => { alert(`Submitted: ${JSON.stringify(data)}`); }) .catch((error) => { console.error('Error:', error); }); }; return ( <form onSubmit={handleSubmit}> <label htmlFor="name">Name:</label> <input type="text" id="name" name="name" /> <br /> <label htmlFor="email">Email:</label> <input type="email" id="email" name="email" /> <br /> <button type="submit">Submit</button> </form> ); }
In this example, we define a function called handleSubmit that gets called when the user submits the form. Inside the function, we create a new FormData object from the form, which we can then submit to the server using the fetch API. When the server responds, we display the submitted data in an alert box.
3. Conclusion
In summary, ReactJS provides two ways to handle form inputs: controlled and uncontrolled components. Controlled components provide a consistent way to manage the state of form inputs, making it easier to implement features like input validation and dynamic input fields. Uncontrolled components provide a simpler way to handle form inputs when you don’t need to manage the input value in React’s state. They can also be useful when working with third-party libraries that require uncontrolled input fields.
Choosing the right approach depends on the specific requirements of your project. If you need more control over the form’s state, controlled components are a better choice. If you want a simpler way to handle form inputs, uncontrolled components may be a better choice. By understanding the differences between controlled and uncontrolled components, you can choose the right approach for your project and build better forms in your React applications.
Table of Contents
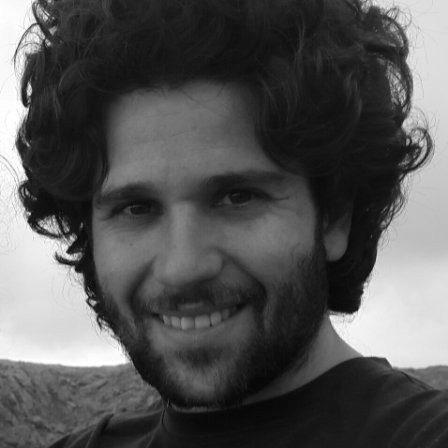
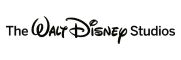