How to Build a Custom React Hook for Data Fetching
React hooks are a powerful feature introduced in React 16.8 that allows developers to use state and other React features without using class components. One popular use case for React hooks is data fetching. With custom hooks, you can create reusable logic that can be used across your application to fetch data from APIs.
Table of Contents
In this article, we’ll learn how to build a custom React hook for data fetching. We’ll start by looking at the basics of React hooks and data fetching, and then we’ll walk through the steps involved in building a custom hook. We’ll also provide a code example to demonstrate how to use the hook in a React application.
1. Basics of React Hooks
React hooks are functions that let you use React features, such as state and lifecycle methods, in functional components. Hooks allow you to use state and other React features without using class components, which can make your code cleaner and easier to understand.
There are several built-in React hooks, such as useState and useEffect, which are used to manage state and perform side effects respectively. These hooks are easy to use and can be used in any functional component.
React hooks were introduced in React 16.8 as a way to manage stateful logic in functional components. Prior to hooks, stateful logic could only be handled in class components using the setState() method.
The useState hook allows you to add state to functional components. It takes an initial value as an argument and returns an array containing the current state value and a function to update the state.
const [count, setCount] = useState(0);
The useEffect hook allows you to perform side effects, such as fetching data or updating the DOM, in functional components. It takes a function as an argument and is called after every render.
useEffect(() => { // Perform side effect here }, [dependency]);
The useReducer hook is used to manage complex state and is similar to Redux. It takes a reducer function and an initial state as arguments and returns an array containing the current state and a dispatch function to update the state.
const [state, dispatch] = useReducer(reducer, initialState);
There are several other built-in hooks in React, such as useCallback, useMemo, and useRef. These hooks allow you to optimize performance and manage other aspects of stateful logic.
2. Basics of Custom React Hooks
Custom React Hooks are JavaScript functions that allow you to reuse stateful logic across multiple components. They are essentially a way to extract common logic from components into reusable functions. One of the benefits of using custom React Hooks is that it simplifies the code and makes it easier to read and maintain.
One of the most commonly used hooks for data fetching is the useEffect Hook. The useEffect Hook allows you to perform side effects, such as data fetching after a component has been rendered. It takes two parameters: a function that contains the side effect, and an array of dependencies that determine when the effect should run.
3. Why Use Custom Hooks?
React hooks provide a way to reuse stateful logic across components. Custom hooks take this a step further by allowing you to create reusable hooks that encapsulate complex logic and can be shared across multiple components or even projects.
Custom hooks can be used to abstract away complex logic such as data fetching, managing local storage, or handling form submissions. This makes your code more modular, easier to maintain, and less prone to bugs.
4. Basics of Data Fetching
Data fetching is a common operation in modern web applications. When building a web application, you often need to retrieve data from an API to display it to the user. This data can be in various formats such as JSON, XML, or plain text.
In React, you can use the built-in fetch API to retrieve data from an API. Fetch is a JavaScript API for making HTTP requests. It returns a Promise that resolves to the response from the server.
Here’s an example of using fetch to retrieve data from an API:
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { // Do something with the data }) .catch(error => { // Handle the error });
In this example, we’re using fetch to retrieve data from the URL ‘https://api.example.com/data‘. We’re then using the .json() method to convert the response to a JavaScript object. Finally, we’re using the .then() method to handle the data and the .catch() method to handle any errors that might occur.
5. Building a Custom React Hook for Data Fetching
Now that we’ve covered the basics of custom React Hooks, let’s dive into how to build a custom React Hook for data fetching.
5.1 Identify the API endpoint
Before you start building the custom hook, you need to identify the API endpoint you want to fetch data from. In this example, we’ll use the JSONPlaceholder API, which provides fake data for testing and prototyping.
5.2 Define the Custom Hook
To define a custom React Hook for data fetching, you need to create a function that takes in the API endpoint as a parameter and returns an array with two values: the data and a boolean value that indicates whether the data is loading.
import { useState, useEffect } from 'react'; const useFetchData = (url) => { const [data, setData] = useState(null); const [isLoading, setIsLoading] = useState(false); useEffect(() => { const fetchData = async () => { setIsLoading(true); try { const response = await fetch(url); const json = await response.json(); setData(json); } catch (error) { console.log(error); } finally { setIsLoading(false); } }; fetchData(); }, [url]); return [data, isLoading]; }; export default useFetchData;
5.3 Fetching data using the Custom Hook
Now that we’ve defined the custom hook, we can use it in our components. To fetch data using the custom hook, we need to pass in the API endpoint as a parameter.
import useFetchData from './useFetchData'; const App = () => { const [data, isLoading] = useFetchData( 'https://jsonplaceholder.typicode.com/todos' ); return ( <div> {isLoading ? ( <p>Loading...</p> ) : ( <ul> {data && data.map((item) => ( <li key={item.id}>{item.title}</li> ))} </ul> )} </div> ); }; export default App;
5.4 Handling errors
To handle errors when fetching data, we can add a try-catch block in the fetchData function in the custom hook.
useEffect(() => { const fetchData = async () => { setIsLoading(true); try { const response = await fetch(url); const json = await response.json(); setData(json); } catch (error) { console.log(error); setIsError(true); } finally { setIsLoading(false); } }; fetchData(); }, [url]);
Next, we’ll define state to store the data and any errors that might occur during the data fetching process.
function useFetch(url, options) { const [data, setData] = useState(null); const [error, setError] = useState(null); // Custom hook code goes here }
We’ve defined two state variables, data, and error, using the useState hook. We’ve initialized them to null since we don’t know what the data or error might be at this point.
The next step is to define the logic that will be used to fetch the data from the API. We’ll use the useEffect hook to perform the data fetching.
function useFetch(url, options) { const [data, setData] = useState(null); const [error, setError] = useState(null); useEffect(() => { fetch(url, options) .then(response => response.json()) .then(data => { setData(data); }) .catch(error => { setError(error); }); }, [url, options]); return { data, error }; }
In this example, we’ve used the useEffect hook to fetch data from the API specified by the URL parameter. We’ve also passed the options parameter to the fetch method to customize the request.
Once the data is fetched, we use the setData function to update the state with the fetched data. If an error occurs during the data fetching process, we use the setError function to update the state with the error.
Finally, we return an object containing the data and error state variables so that they can be accessed by the component that is using the custom hook.
const MyComponent = () => { const { data, error } = useFetch("https://api.example.com/data", {}); if (error) { return <div>Error: {error.message}</div>; } if (!data) { return <div>Loading...</div>; } return ( <div> {data.map(item => ( <div key={item.id}>{item.name}</div> ))} </div> ); };
In this example, we’ve used the useFetch custom hook to fetch data from the API and store it in the data state variable. We’ve also used the error state variable to handle any errors that might occur during the data fetching process.
The MyComponent function displays the data in the data state variable if it is available. If an error occurs during the data fetching process, the error message is displayed using the error state variable.
6. Customizing the Custom Hook
Our custom hook is now complete, but we can still make some improvements to make it more customizable.
One way to do this is to add a loading state variable to indicate when the data is being fetched. We can use the useState hook to define the isLoading state variable and set it to true before fetching the data.
function useFetch(url, options) { const [data, setData] = useState(null); const [error, setError] = useState(null); const [isLoading, setIsLoading] = useState(true); useEffect(() => { fetch(url, options) .then(response => response.json()) .then(data => { setData(data); setIsLoading(false); }) .catch(error => { setError(error); setIsLoading(false); }); }, [url, options]); return { data, error, isLoading }; }
We’ve added a new isLoading state variable using the useState hook. We’ve also set it to true before fetching the data and updated it to false once the data has been fetched or an error has occurred.
We’ve also updated the return statement to include the isLoading state variable.
const MyComponent = () => { const { data, error, isLoading } = useFetch("https://api.example.com/data", {}); if (isLoading) { return <div>Loading...</div>; } if (error) { return <div>Error: {error.message}</div>; } if (!data) { return <div>No data found</div>; } return ( <div> {data.map(item => ( <div key={item.id}>{item.name}</div> ))} </div> ); };
In this updated example, we’ve added a check for the isLoading state variable before displaying the data. If the isLoading state variable is true, we display a loading message. Once the data has been fetched or an error has occurred, we display the appropriate message using the data and error state variables.
6.1 Using the Custom Hook
Now that we’ve built our custom hook, let’s look at how to use it in a React component.
Here’s an example of a component that uses our custom hook to retrieve data from an API:
import React from 'react'; import useFetch from './useFetch'; function App() { const { data, error } = useFetch('https://api.example.com/data'); if (error) { return <div>Error: {error.message}</div>; } if (!data) { return <div>Loading...</div>; } return ( <div> {data.map(item => ( <div key={item.id}>{item.name}</div> ))} </div> ); } export default App;
In this example, we’re importing our custom hook, useFetch, and using it to retrieve data from the URL ‘https://api.example.com/data‘. We’re using destructuring to extract the data and error variables from the object returned by useFetch.
If there’s an error, we’re rendering an error message. If the data hasn’t been retrieved yet, we’re rendering a loading message. Otherwise, we’re rendering the retrieved data as a list of items.
6.2 Optimizing the Custom Hook
To optimize the custom hook, we can add caching to prevent unnecessary API requests. We can use the useRef Hook to store the data, and only fetch new data if the URL has changed.
import { useState, useEffect, useRef } from 'react'; const useFetchData = (url) => { const [data, setData] = useState(null); const [isLoading, setIsLoading] = useState(false); const [isError, setIsError] = useState(false); const cache = useRef({}); useEffect(() => { if (cache.current[url]) { setData(cache.current[url]); setIsLoading(false); } else { const fetchData = async () => { setIsLoading(true); try { const response = await fetch(url); const json = await response.json(); setData(json); cache.current[url] = json; } catch (error) { console.log(error); setIsError(true); } finally { setIsLoading(false); } }; fetchData(); } }, [url]); return [data, isLoading, isError]; }; export default useFetchData;
7. Conclusion
In this article, we’ve learned how to build a custom hook for data fetching in React. We started by reviewing the basics of React hooks, including `the useState and useEffect hooks. We then created a custom hook using the useEffect hook to fetch data from an API and store it in the data state variable.
We also learned how to handle errors using the setError state variable and how to make the custom hook more customizable by adding a loading state variable.
By building our own custom hook for data fetching, we can reuse this functionality in multiple components throughout our application and reduce code duplication. Custom hooks can also make our code more readable and easier to maintain.
In summary, building a custom hook for data fetching in React involves defining the state variables, using the useEffect hook to fetch data from an API, and returning the data and error state variables. By making the custom hook more customizable, we can add additional functionality such as a loading state variable to improve the user experience.
Table of Contents
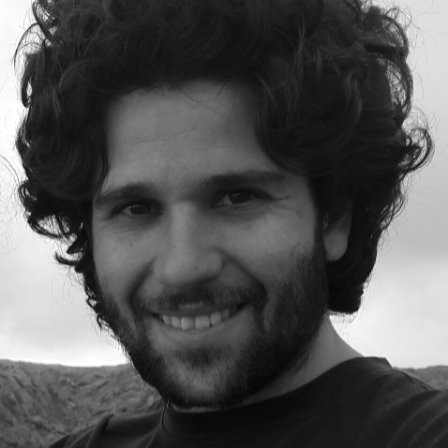
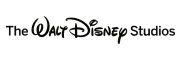