Mastering Binary State Management in ReactJS with a Custom useToggle Hook
In React development, a platform mastered by expert ReactJS developers, we often encounter scenarios where we need to switch between two states. Examples of this include toggling a checkbox, expanding and collapsing a dropdown menu, or showing and hiding a modal. These situations require us to manage binary state data. While React provides a built-in hook, `useState`, for managing state, the frequent pattern of toggling between two states necessitates a more streamlined approach.
Enter the custom toggle hook. A custom hook is a JavaScript function, often crafted by seasoned ReactJS developers, whose name begins with “use” that may call other hooks. This allows us to create a custom hook for a repetitive or complex state logic to keep our components clean, reusable, and maintainable. The development of such solutions often leads businesses to hire ReactJS developers for their projects.
In this blog post, we’ll delve into how to create a custom hook `useToggle` that simplifies binary state management in our React applications. This is a great illustration of the added value that comes when you hire ReactJS developers – expertise in creating streamlined, efficient solutions for common coding scenarios.
Defining our useToggle Hook
Let’s start by defining our `useToggle` hook. We’ll use the `useState` hook to manage our binary state data and a function to toggle this state.
```jsx import { useState } from 'react'; function useToggle(initialValue = false) { const [value, setValue] = useState(initialValue); const toggleValue = () => setValue(!value); return [value, toggleValue]; } ```
In this hook, we first use `useState` to create a state variable `value` and a setter `setValue`. `value` is a boolean that indicates the current state. `initialValue` is an optional parameter that sets the initial state.
The `toggleValue` function toggles the `value` state when called, without needing to know the current state. When we call `useToggle`, it returns an array containing the current state and a function to toggle this state. This design is similar to the `useState` hook, offering familiarity and predictability.
Using our useToggle Hook
Toggle a Text Display
Here’s an example of how we can use our `useToggle` hook in a component to toggle the display of some text.
```jsx import React from 'react'; import useToggle from './useToggle'; function TextToggle() { const [isTextVisible, toggleTextVisibility] = useToggle(false); return ( <div> <button onClick={toggleTextVisibility}> Toggle Text </button> {isTextVisible && <p>Here is some toggleable text!</p>} </div> ); } export default TextToggle; ```
In this `TextToggle` component, we use our `useToggle` hook to create a state `isTextVisible` and a toggle function `toggleTextVisibility`. Initially, `isTextVisible` is set to false, so the text is hidden. When the button is clicked, `toggleTextVisibility` is called to toggle `isTextVisible`, showing or hiding the text accordingly.
Toggle a Modal
Here’s another example where we use our `useToggle` hook to toggle a modal.
```jsx import React from 'react'; import useToggle from './useToggle'; import Modal from './Modal'; function App() { const [isModalOpen, toggleModal] = useToggle(false); return ( <div> <button onClick={toggleModal}> {isModalOpen ? 'Close Modal' : 'Open Modal'} </button> {isModalOpen && <Modal />} </div> ); } export default App; ```
In this `App` component, we use our `useToggle` hook to manage the modal’s open state. The button’s text reflects the current state, and clicking the button toggles the modal. Our `useToggle` hook makes the code clean and easy to understand.
Enhancing our useToggle Hook
Our `useToggle` hook is already functional, but we can enhance it further. Let’s make our `toggleValue` function more flexible by allowing it to toggle to a specific state, not just the opposite state.
```jsx import { useState } from 'react'; function useToggle(initialValue = false) { const [value, setValue] = useState(initialValue); const toggleValue = (value) => setValue(currentValue => typeof value === "boolean" ? value : !currentValue ); return [value, toggleValue]; } ```
With this enhancement, we can still call `toggleValue()` without parameters to switch to the opposite state. Alternatively, we can pass a boolean value to `toggleValue(value)` to set a specific state.
Conclusion
By leveraging custom hooks in React, we can create reusable logic like our `useToggle` hook to handle common binary state toggling situations. This not only helps us write cleaner and more maintainable code, but also encapsulates complex logic, enhancing the readability of our components. It’s this level of detailed knowledge and skill that you get when you hire ReactJS developers.
Whether you’re just beginning your journey with React or already have substantial experience, understanding and creating custom hooks like `useToggle` will undoubtedly boost your efficiency in developing React applications. If you’re looking to scale your project quickly, hiring expert ReactJS developers can be a game-changing decision.
Remember, hooks are not just about `useState` or `useEffect`; they are a powerful way to abstract component logic, making your React code more reusable and your components more self-contained. Take advantage of these practices to supercharge your development process, or consider hiring seasoned ReactJS developers to help navigate this efficiently.
Table of Contents
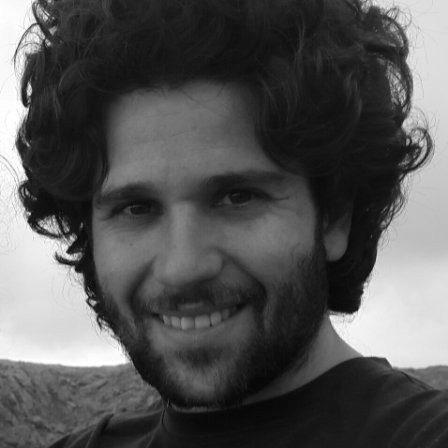
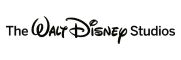