Implementing Dark Mode in Your ReactJS application using useDarkMode
ReactJS, a prominent JavaScript library, has transformed web development since its introduction by Facebook in 2013. It simplifies the creation of interactive UIs through its component-based architecture.
A crucial aspect of modern user experience is Dark Mode, a feature that offers visual appeal and several user benefits, such as reduced eye strain and energy conservation. Dark Mode, once a luxury, is now a necessity for web applications.
To accommodate Dark Mode in React applications, ‘useDarkMode,’ a custom hook, has been introduced. It simplifies the implementation of Dark Mode, managing user theme preferences and toggling between themes. In this guide, we’ll explore the useDarkMode hook and its application in your ReactJS project, helping you effortlessly introduce Dark Mode into your applications.
What is Dark Mode and Why is it Important?
Dark Mode, as the name implies, is a user interface (UI) option that alters an application’s color scheme to display light text on a dark background. Traditional applications are designed with a ‘light mode,’ employing a light background with dark text. Dark Mode essentially inverts this scheme.
Originally introduced by operating systems and applications to reduce eye strain in low-light conditions, Dark Mode has now evolved into a standard feature on many platforms, apps, and websites, offering a visually relaxing alternative to the typical bright, white backgrounds.
But why is Dark Mode so important? Here are some key reasons:
- Enhanced User Experience
The foremost reason is user preference and comfort. Some users find Dark Mode less straining on the eyes, especially in low-light or nighttime environments. It can also help minimize screen glare, providing a comfortable reading experience.
- Energy Efficiency
Dark Mode can contribute to battery saving on OLED and AMOLED screens. These screens emit light from each pixel individually. Therefore, when the background is black (as it is in Dark Mode), the pixels are effectively off, thus using less power.
- Reduced Eye Strain
Prolonged exposure to light backgrounds can cause digital eye strain, which includes symptoms like dry eyes, blurred vision, and headaches. Dark Mode, by reducing light emission, can alleviate these symptoms.
- Aesthetics
Dark Mode imparts a sleek, modern look to applications. For many users, the appeal is purely visual. Dark Mode brings certain design elements to the fore, improving visual ergonomics by reducing eye strain, and making it easier to see the details on the screen.
The adoption of Dark Mode, therefore, not only caters to user preference but also provides tangible benefits such as energy conservation and improved user comfort. Given its increasing popularity and demand, integrating Dark Mode into your application could be a significant enhancement to your application’s user experience.
Introduction to useDarkMode
In the realm of ReactJS, hooks have revolutionized the way we write and manage state in functional components, fostering clean and readable code. One such hook that has gained traction for its straightforward yet powerful functionality is `useDarkMode`.
`useDarkMode` is a custom hook specifically designed to handle the integration of Dark Mode in your ReactJS applications. It does the heavy lifting for you, automatically managing the theme state in your application, switching between light and dark themes, and persisting the user’s theme preference across sessions.
When invoked, `useDarkMode` provides you with a `darkMode` state variable and a `toggle` function. The `darkMode` variable is a boolean indicating whether Dark Mode is currently activated (true if Dark Mode, false for Light Mode). The `toggle` function, as the name suggests, is used to switch between Dark Mode and Light Mode.
But what makes `useDarkMode` truly impressive is its ability to remember the user’s preferred theme. If a user selects Dark Mode, this preference is stored in the browser’s localStorage. The next time the user visits your application, `useDarkMode` checks this stored value and automatically applies the user’s preferred theme, ensuring a consistent user experience.
`useDarkMode` thus simplifies the implementation of Dark Mode, allowing you to focus on building robust and engaging applications while it takes care of the intricacies of theme management.
Implementing useDarkMode in a ReactJS Application
Before implementing Dark Mode, ensure that you have a functioning ReactJS application. If you are starting from scratch, you can create a new application using Create React App, Next.js, or your preferred React setup.
After setting up your project, you need to install the use-dark-mode package. It can be installed via npm (Node Package Manager) or Yarn.
With npm, use this command:
npm install use-dark-mode
With Yarn, use:
yarn add use-dark-mode
Once the package is installed, you can start using `useDarkMode` in your React components.
Here is a simple example of how to integrate `useDarkMode` into a functional component:
import React from 'react'; import useDarkMode from 'use-dark-mode'; const DarkModeToggle = () => { const darkMode = useDarkMode(false); return ( <div> <button type="button" onClick={darkMode.disable}> ? </button> <button type="button" onClick={darkMode.enable}> ? </button> </div> ); }; export default DarkModeToggle;
In the example above, the `useDarkMode` hook is imported and used in the `DarkModeToggle` component. It’s called with an initial value of `false`, which means that Dark Mode is off by default.
The `darkMode` object returned by the hook contains several properties and methods. The `disable` and `enable` methods are used to switch off and on the Dark Mode, respectively.
These methods are attached to two buttons in the component. When the ‘sun‘ button is clicked, the `disable` method is triggered, turning off Dark Mode (i.e., switching to Light Mode). Conversely, when the ‘moon‘ button is clicked, the `enable` method is triggered, turning on Dark Mode.
And that’s it! With just a few lines of code, you’ve integrated Dark Mode into your application. The user’s preference will be remembered between sessions thanks to the useDarkMode hook’s built-in localStorage functionality. Now, you can start adding Dark Mode styles to your application and give your users the choice between Light and Dark Mode.
Customizing Dark Mode
After successfully integrating the `useDarkMode` hook into your application, you can start customizing the Dark Mode appearance to match your branding and design principles.
- Adjusting Dark Mode Color Schemes:
The first step in customizing Dark Mode is adjusting the color scheme. CSS custom properties (also known as CSS variables) can be an effective way to manage color schemes for different modes.
Here’s an example of how you might define color variables in your CSS for light and dark themes:
:root { --color-background: white; --color-text: black; } body.dark-mode { --color-background: black; --color-text: white; }
In your components, you can use these variables like so:
body { background-color: var(--color-background); color: var(--color-text); }
When you toggle Dark Mode, simply add or remove the `dark-mode` class to/from the `body` element, and the colors will adjust automatically.
- Creating a Toggle Button
While the previous example used two separate buttons for enabling and disabling Dark Mode, you may prefer to use a single toggle button. The `toggle` function returned by the `useDarkMode` hook allows for this:
import React from 'react'; import useDarkMode from 'use-dark-mode'; const DarkModeToggle = () => { const darkMode = useDarkMode(false); return ( <div> <button type="button" onClick={darkMode.toggle}> {darkMode.value ? '?' : '?'} </button> </div> ); }; export default DarkModeToggle;
This button will display a moon symbol when in Light Mode, and a sun symbol when in Dark Mode. Clicking the button will toggle between the two modes.
- Retaining the User’s Mode Preference
A key feature of the `useDarkMode` hook is its ability to remember the user’s preferred mode across sessions using the browser’s localStorage. This is handled automatically by the hook, providing a consistent user experience with no extra code required on your part.
These are some basic ways to customize Dark Mode in your application. Depending on your requirements, you might add transitions for smooth mode switching, apply different styling for images or other media, or provide additional theme options for users. With the groundwork laid by the `useDarkMode` hook, you’re free to get creative with your Dark Mode implementation!
Real-world Examples and Applications
Understanding theoretical aspects and implementation details is crucial, but examining real-world examples and applications can provide practical insights into how Dark Mode is employed across various platforms.
- Operating Systems (macOS, Windows, Android, iOS)
Dark Mode has become an integral part of modern operating systems. It’s not only used within the system interfaces but is also applied to native applications and features, such as calendars, mail apps, and more.
- Social Media (Twitter, Instagram, Facebook)
Major social media platforms have embraced Dark Mode, recognizing its popularity among users. Twitter was one of the first platforms to offer this feature. Instagram and Facebook followed suit, integrating Dark Mode into their user interfaces to enhance user comfort during late-night browsing sessions.
- Productivity Apps (Slack, Notion)
Many productivity apps have introduced Dark Mode to reduce eye strain and make prolonged usage more comfortable. Slack, for instance, offers a Dark Mode to make reading and writing messages easier on the eyes. Notion, a note-taking and project management app, also includes Dark Mode, making late-night project planning or note-taking sessions less strenuous.
- Web Browsers (Chrome, Firefox)
Even web browsers have caught on to the Dark Mode trend. Both Chrome and Firefox offer Dark Mode settings, allowing users to browse the internet with reduced eye strain.
- IDEs and Text Editors (VS Code, Atom)
Integrated Development Environments (IDEs) and text editors are where developers spend most of their time. Dark themes have been a staple in these tools for years due to their comfort and reduced eye strain. VS Code, for example, comes with a built-in dark theme and allows for numerous dark theme extensions.
All these applications and platforms implement Dark Mode in slightly different ways, but they all use it to achieve the same goal: enhancing the user experience by providing a visually comfortable and appealing interface. Incorporating Dark Mode into your own application can therefore not only meet user expectations but also give your application a modern, user-friendly edge.
Conclusion
Incorporating Dark Mode in applications is a key trend in modern UI design, offering significant user experience benefits. With ReactJS and its `useDarkMode` hook, implementing Dark Mode has been simplified, making it an accessible feature for any application. We’ve explored its importance, how to use `useDarkMode`, customizing this feature, and its real-world applications. As a developer, embracing Dark Mode is an opportunity to enhance user satisfaction, engagement, and align your application with contemporary, user-focused design standards.
Table of Contents
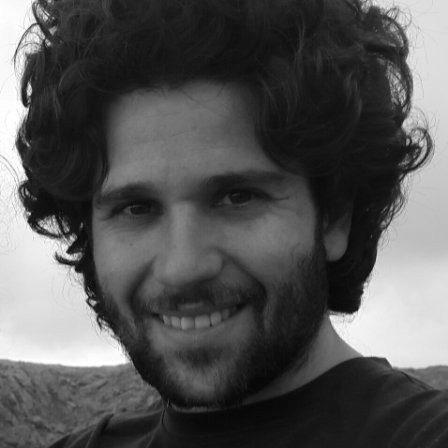
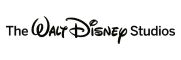