Accelerate Development with Reusable Components in ReactJS
ReactJS, a popular JavaScript library for building user interfaces, has become an essential tool for front-end developers. TypeScript, a powerful superset of JavaScript, provides static typing, enabling developers to catch errors early, improve code quality, and make the development process more efficient. Combining ReactJS and TypeScript creates a powerful synergy that can take your projects to new heights.
Table of Contents
In this blog post, we’ll explore the benefits of using TypeScript with ReactJS, walk you through setting up a React and TypeScript project, and provide tips for maximizing the advantages of this match made in heaven.
1. Why Use TypeScript with ReactJS?
TypeScript offers several benefits when used in conjunction with ReactJS:
- Improved Code Quality: TypeScript’s static typing helps you catch errors early in the development process, preventing runtime issues and improving code quality.
- Better Developer Experience: TypeScript’s advanced IntelliSense features, such as autocompletion and type inference, make it easier to understand and navigate codebases, enhancing the development experience.
- Easier Refactoring: TypeScript’s type system allows for more precise and less error-prone refactoring, making it simpler to maintain and update your code.
- Better Collaboration: TypeScript’s self-documenting nature makes it easier for teams to collaborate on projects, as the type annotations provide clarity and context.
2. Setting Up a React and TypeScript Project
To create a new React project with TypeScript, follow these steps:
2.1 Install the Create React App CLI tool globally (if you haven’t already)
bash
npm install -g create-react-app
2.2 Create a new project using the –template typescript flag.
bash
create-react-app my-react-typescript-app --template typescript
2.3 Change into the project directory and start the development server.
bash
cd my-react-typescript-app npm start
3. Using TypeScript with React Components
To use TypeScript in your React components, create your component files with the .tsx extension instead of .js. TypeScript enables you to type your component props and state for better error-checking and improved developer experience.
Here’s an example of a typed React component:
tsx
import React, { useState } from "react"; interface CounterProps { initialCount: number; } const Counter: React.FC<CounterProps> = ({ initialCount }) => { const [count, setCount] = useState<number>(initialCount); return ( <div> <h2>Count: {count}</h2> <button onClick={() => setCount(count + 1)}>Increment</button> <button onClick={() => setCount(count - 1)}>Decrement</button> </div> ); }; export default Counter;
In the example above, we define an interface CounterProps to type the component’s props and use the React.FC type for the functional component. We also type the state using TypeScript’s built-in types.
4. Tips for Maximizing the Benefits of React and TypeScript
4.1 Use Type Aliases and Interfaces:
Leverage TypeScript’s type aliases and interfaces to create reusable and self-documenting types for your components and data structures.
4.2 Type Event Handlers:
Improve code quality and avoid runtime issues by typing event handlers and their corresponding event objects.
tsx
const handleInputChange = (event: React.ChangeEvent<HTMLInputElement>) => { setInputValue(event.target.value); };
4.3 Type Higher-Order Components and Context:
Use TypeScript’s generic types to create strongly-typed higher-order components and React context providers and consumers.
4.4 Utilize Utility Types:
TypeScript offers utility types like Partial, Readonly, and Pick that can help you manipulate and transform existing types for greater flexibility and code reusability.
tsx
interface User { id: number; name: string; email: string; } type UserWithoutEmail = Pick<User, "id" | "name">;
4.5 Leverage Third-Party Type Definitions:
Many popular libraries and packages provide TypeScript type definitions either built-in or through the DefinitelyTyped project. Use these type definitions to ensure type safety when working with third-party libraries in your React and TypeScript projects.
bash
npm install --save-dev @types/react-router-dom
4.6 Configure TypeScript Strict Mode:
Enable strict mode in your tsconfig.json file to enforce stricter type-checking and catch more potential issues during development.
json
{ "compilerOptions": { // ... "strict": true // ... } }
4.7. Use Type Guards and Type Assertions:
Utilize TypeScript’s type guards and type assertions to provide more precise type information to the compiler, especially when working with conditional rendering or complex data structures.
tsx
const renderContent = (data: string | null) => { if (typeof data === "string") { return <p>{data}</p>; } return <p>No data available.</p>; };
5. Conclusion
ReactJS and TypeScript together form a powerful combination that can improve code quality, enhance the development experience, and make it easier to maintain and update your projects. By following the tips and best practices outlined in this blog post, you’ll be well on your way to maximizing the benefits of this match made in heaven. Embrace the synergy of ReactJS and TypeScript, and unlock your full potential as a front-end developer.
Table of Contents
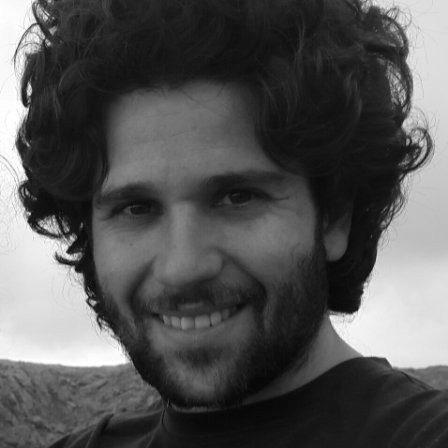
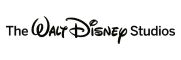