Ensuring Application Security with ReactJS: Best Practices to Prevent Common Vulnerabilities
Securing your web application is a critical aspect of development. ReactJS, a popular JavaScript library for building user interfaces, provides some built-in security features, but developers must also implement best practices to protect their applications from common vulnerabilities.
Table of Contents
In this blog post, we’ll discuss several ReactJS security best practices to help you build robust and secure applications.
1. Prevent Cross-Site Scripting (XSS) Attacks
Cross-Site Scripting (XSS) attacks occur when an attacker injects malicious code into your application, potentially compromising user data and application integrity. ReactJS automatically escapes HTML and JavaScript content rendered in JSX, which helps prevent most XSS attacks. However, you should still be cautious when rendering user-generated content or using React’s dangerouslySetInnerHTML property.
To mitigate XSS risks:
- Avoid using dangerouslySetInnerHTML whenever possible.
- Sanitize user-generated content before rendering it. Use a trusted library like DOMPurify to cleanse the content of malicious scripts.
2. Use Content Security Policy (CSP)
Content Security Policy (CSP) is a security feature that helps prevent cross-site scripting and other code injection attacks. By specifying which sources of content are allowed to be loaded by the browser, CSP can reduce the risk of an attacker injecting malicious code into your application.
To implement CSP in your React application:
- Configure your server to return the Content-Security-Policy HTTP header with a policy tailored to your application’s requirements.
- Test your policy with a tool like the Google CSP Evaluator to ensure it doesn’t inadvertently block legitimate content.
3. Protect against Cross-Site Request Forgery (CSRF)
Cross-Site Request Forgery (CSRF) is an attack that forces an end user to execute unwanted actions on a web application they’re currently authenticated to. To protect your React application from CSRF attacks, use anti-CSRF tokens, which are unique and unpredictable values generated on the server-side and included in forms or URLs.
To implement anti-CSRF tokens in your React application:
- Generate a unique CSRF token for each user session on the server-side.
- Include the CSRF token in your forms and verify it on the server-side when processing form submissions.
4. Validate and Sanitize Input Data
Validating and sanitizing user input is crucial to prevent security vulnerabilities and maintain data integrity. Input validation ensures that user input meets specific criteria, while input sanitization cleanses input data by removing or modifying potentially harmful characters.
To validate and sanitize input data in your React application:
- Use built-in form validation features, like setting the required attribute on form elements or using the pattern attribute for input validation.
- Leverage third-party libraries like Yup or Joi for more complex validation rules.
- Sanitize input data on the server-side using a trusted library like Validator.js.
5. Secure Data Transmission
Securing data transmission between the client and server is essential for protecting sensitive user information.
To ensure secure data transmission:
- Use HTTPS (HTTP Secure) to encrypt data transmitted between the client and server.
- Implement secure authentication mechanisms, such as OAuth or JSON Web Tokens (JWT), to protect user credentials.
- Store sensitive data securely, either by encrypting it or using secure storage solutions like environment variables or dedicated secret managers.
6. Keep Dependencies Updated
Outdated dependencies can introduce security vulnerabilities into your application. Regularly updating your dependencies can help mitigate these risks.
To keep your React application’s dependencies up to date:
- Regularly audit your dependencies using tools like npm audit or yarn audit.
- Keep track of security advisories for your dependencies and apply patches promptly.
- Use tools like Dependabot or Renovate to automate the process of updating dependencies and submitting pull requests for review.
7. Perform Regular Security Audits
Regularly auditing your application for security vulnerabilities is essential for maintaining a secure and robust application.
To perform security audits for your React application:
- Use automated tools like OWASP ZAP, Burp Suite, or Snyk to scan your application for common vulnerabilities.
- Conduct regular code reviews with a focus on security best practices.
- Consider hiring a security expert or engaging a third-party security firm to perform a thorough security audit.
8. Follow the Principle of Least Privilege
The Principle of Least Privilege (POLP) states that users, systems, and applications should only be granted the minimum necessary privileges to perform their tasks. By limiting access to resources and data, you can reduce the potential attack surface and minimize the impact of a security breach.
To implement the Principle of Least Privilege in your React application:
- Use role-based access control (RBAC) to restrict user access to application features based on their roles.
- Limit the permissions and privileges of service accounts, API keys, and tokens.
- Regularly review and update access controls to ensure users and systems have the appropriate level of access.
9. Conclusion
Securing your ReactJS application requires a combination of built-in features, best practices, and regular security audits. By implementing the security best practices discussed in this blog post, you can build a more robust, secure, and resilient application that protects your users and your data from common vulnerabilities. Stay vigilant and proactive in your approach to security, and your application will be better equipped to withstand potential threats.
Table of Contents
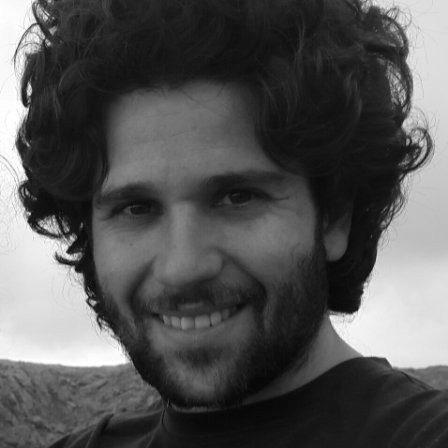
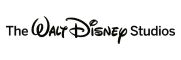