Tips and Tricks for Faster ReactJS Application Performance Optimization
ReactJS is a popular JavaScript library for building dynamic user interfaces. However, as applications become more complex, they can sometimes become slow and unresponsive.
Table of Contents
Performance optimization is a critical aspect of building high-quality ReactJS applications, and there are several tips and tricks that developers can use to improve the performance of their applications.
In this blog post, we’ll explore some best practices for ReactJS performance optimization, including how to use React.memo, avoiding unnecessary renders, code splitting, and more.
1. Using React.memo
React.memo is a higher-order component that can be used to memoize functional components. Memoization is a technique used in computer science to cache the results of expensive function calls, so they don’t need to be recomputed every time they are called.
In ReactJS, memoization can be used to improve performance by reducing the number of re-renders that occur when a component’s props or state changes. By wrapping a component with React.memo, the component will only re-render when its props have changed.
It’s important to note that React.memo should only be used on components that are expensive to render. Using it on every component can actually hurt performance, as memoization adds some overhead to the rendering process.
2. Avoiding Unnecessary Renders
Another way to improve the performance of your ReactJS applications is to avoid unnecessary renders. Unnecessary renders occur when a component is re-rendered, but its output has not changed.
To avoid unnecessary renders, you can use the shouldComponentUpdate lifecycle method or the PureComponent base class. Both of these methods allow you to compare the current props and state of a component to its previous props and state and determine if a re-render is necessary.
Here’s an example of how to use shouldComponentUpdate:
javascript
import React from 'react'; class MyComponent extends React.Component { shouldComponentUpdate(nextProps, nextState) { return nextProps.name !== this.props.name; } render() { return ( <div> {this.props.name} </div> ); } } export default MyComponent;
In this example, the shouldComponentUpdate method is used to compare the current name prop to the previous name prop. If the name has not changed, the component will not re-render.
3. Code Splitting
Code splitting is a technique used to improve the performance of your ReactJS applications by reducing the amount of JavaScript that needs to be loaded when a user visits your site. By breaking your code into smaller chunks and only loading the code that is necessary for a particular page or feature, you can reduce the amount of time it takes for your site to load.
In ReactJS, code splitting can be achieved using tools like Webpack or Rollup. These tools allow you to define entry points for your application and split your code into separate chunks based on those entry points.
Here’s an example of how to use code splitting with Webpack:
javascript
import React, { lazy, Suspense } from 'react'; const LazyComponent = lazy(() => import('./LazyComponent')); function MyComponent() { return ( <div> <Suspense fallback={<div>Loading...</div>}> <LazyComponent /> </Suspense> </div> ); } export default MyComponent;
In this example, the LazyComponent is only loaded when it is needed, reducing the amount of JavaScript that needs to be loaded initially.
4. Using shouldComponentUpdate vs PureComponent
When it comes to avoiding unnecessary renders, there are two approaches that you can take: using shouldComponentUpdate or using the PureComponent base class.
shouldComponentUpdate allows you to define your own logic for determining whether a component should re-render. You can compare the current props and state to the previous props and state and return true if a re-render is necessary.
Pure Component, on the other hand, automatically implements shouldComponentUpdate with a shallow prop and state comparison. This means that if the props and state of a PureComponent have not changed, it will not re-render.
Using PureComponent can be a good way to avoid unnecessary renders, but it’s important to note that it only performs a shallow comparison of props and state. If your component has complex data structures or nested objects, you may need to use shouldComponentUpdate instead.
Here’s an example of how to use PureComponent:
javascript
import React from 'react'; class MyComponent extends React.PureComponent { render() { return ( <div> {this.props.name} </div> ); } } export default MyComponent;
In this example, MyComponent extends React.PureComponent, which automatically implements shouldComponentUpdate with a shallow comparison of props and state.
5. Using React Profiler
React Profiler is a tool that allows you to analyze the performance of your ReactJS applications. It provides detailed information about the rendering time of each component in your application, including the time spent in the render method and the time spent updating the component’s state.
To use React Profiler, you’ll need to add the <React.Profiler> component to your application and provide a callback function that will be called when the component is finished rendering.
Here’s an example of how to use React Profiler:
javascript
import React, { Profiler } from 'react'; function MyComponent() { return ( <Profiler id="MyComponent" onRender={(id, phase, actualDuration) => { console.log(`Component ${id} took ${actualDuration}ms to render`); }}> <div> {/* your component code */} </div> </Profiler> ); } export default MyComponent;
In this example, the <Profiler> component is used to measure the rendering time of MyComponent. When the component is finished rendering, the onRender callback function is called, which logs the actual duration of the rendering process to the console.
6. Conclusion
ReactJS is a powerful library for building dynamic user interfaces, but as applications become more complex, they can sometimes become slow and unresponsive. Performance optimization is a critical aspect of building high-quality ReactJS applications, and there are several tips and tricks that developers can use to improve the performance of their applications.
In this blog post, we’ve explored some best practices for ReactJS performance optimization, including using React.memo, avoiding unnecessary renders, code splitting, using shouldComponentUpdate vs PureComponent, and using React Profiler. By following these tips and tricks, you can ensure that your ReactJS applications are fast, responsive, and efficient.
Table of Contents
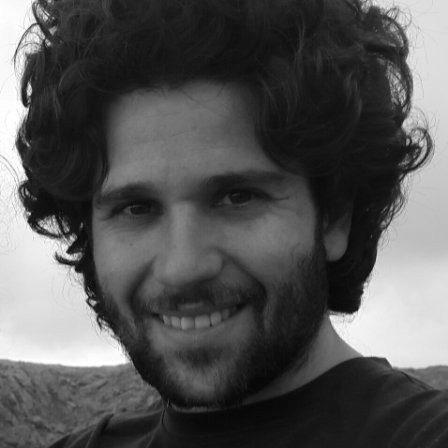
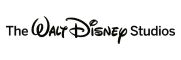