ReactJS Q & A
How to handle forms in React?
To effectively manage forms in React, it’s essential to employ controlled components. Begin by establishing a state variable using the useState hook to store the form’s data. Link input fields to this state variable by defining their value and onChange props. This approach ensures that the form’s UI always reflects the current state of the data, enabling user input changes to seamlessly update the state. When the form is submitted, manage the data as required, such as making API requests or performing client-side processing. Controlled components offer a streamlined approach for synchronizing form data and UI, simplifying data management and input validation.
Here’s an example:
import React, { useState } from 'react'; function MyForm() { const [formData, setFormData] = useState({ username: '', email: '' }); const handleChange = (e) => setFormData({ ...formData, [e.target.name]: e.target.value }); const handleSubmit = (e) => { e.preventDefault(); console.log(formData); // Perform actions with formData here. }; return ( <form onSubmit={handleSubmit}> <input type="text" name="username" value={formData.username} onChange={handleChange} placeholder="Username" /> <input type="email" name="email" value={formData.email} onChange={handleChange} placeholder="Email" /> <button type="submit">Submit</button> </form> ); } export default MyForm;
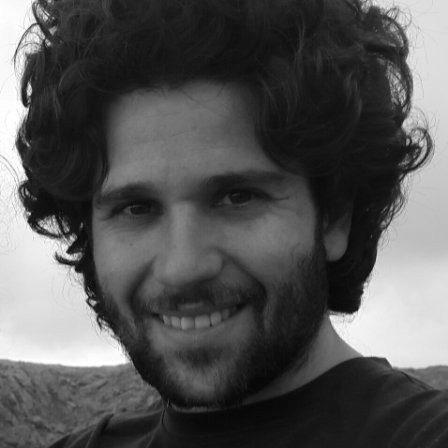
Previously at
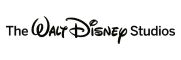
Seasoned Software Engineer specializing in React.js development. Over 5 years of experience crafting dynamic web solutions and collaborating with cross-functional teams.