A Comprehensive Guide to Getting Started with ReactJS
ReactJS is a popular JavaScript library for building user interfaces. Whether you’re a seasoned developer or just starting out, ReactJS can be a powerful tool for building dynamic and engaging web applications. Table of Contents
In this comprehensive guide, we’ll cover everything you need to know to get started with ReactJS. We’ll cover the basics of ReactJS, including its component-based architecture and JSX syntax, and we’ll explore some of the key features of the library, such as state management and lifecycle methods.
Getting Started with ReactJS
1. Setting Up Your Development Environment
Before you can start building ReactJS applications, you’ll need to set up your development environment. To get started, you’ll need to install Node.js, which includes the npm package manager. You can download Node.js from the official website.
Once you’ve installed Node.js, you can use npm to install the create-react-app command line tool. This tool provides a quick and easy way to create a new ReactJS project.
To create a new ReactJS project, simply open a terminal window and type the following command:
lua
npx create-react-app my-app
This will create a new ReactJS project in a directory called “my-app”. You can then navigate to this directory and start the development server by running the following commands:
bash
cd my-app npm start
This will start the development server and open your new ReactJS application in your default web browser.
2. Understanding ReactJS Components
One of the key features of ReactJS is its component-based architecture. ReactJS components are self-contained units of functionality that can be composed together to create a complete user interface.
ReactJS components can be defined using either JavaScript classes or functions. Class components are more powerful and can include state and lifecycle methods, while function components are simpler and more lightweight.
Here’s an example of a simple function component:
javascript
function HelloWorld() { return <div>Hello, World!</div>; }
This component simply renders a “Hello, World!” message to the screen. You can include this component in other components by importing it and rendering it as JSX.
3. Using JSX Syntax
JSX is a syntax extension for JavaScript that allows you to write HTML-like code in your ReactJS components. JSX is not required to use ReactJS, but it is highly recommended because it makes your components more readable and easier to understand.
Here’s an example of a JSX component:
javascript
function Greeting(props) { return <div>Hello, {props.name}!</div>; }
This component takes a “name” prop and uses it to render a personalized greeting to the screen. Notice how the “name” prop is included within curly braces in the JSX.
4. Managing Component State
In ReactJS, component state is used to manage data that changes over time. State is typically used to manage user input, application settings, and other dynamic data.
To manage state in ReactJS, you can use the useState hook. This hook allows you to add state to your function components without having to convert them to class components.
Here’s an example of a component that uses the useState hook to manage a counter:
javascript
function Counter() { const [count, setCount] = useState(0); function handleClick() { setCount(count + 1); } return ( <div> <p>You clicked {count} times.</p> <button onClick={handleClick}>Click me</button> </div> ); }
This component uses the useState hook to add a “count” state variable to the component. The component also includes a button that, when clicked, updates the count state variable.
5. Working with Lifecycle Methods
Lifecycle methods are a key feature of ReactJS that allow you to perform actions at different stages of a component’s lifecycle. Lifecycle methods can be used to manage component state, fetch data from external APIs, and perform other actions.
There are several lifecycle methods available in ReactJS, including componentDidMount, componentDidUpdate, and componentWillUnmount. These methods are called in a specific order during the component’s lifecycle.
Here’s an example of a component that uses the componentDidMount lifecycle method to fetch data from an external API:
javascript
class UserList extends React.Component { constructor(props) { super(props); this.state = { users: [] }; } async componentDidMount() { const response = await fetch('https://jsonplaceholder.typicode.com/users'); const data = await response.json(); this.setState({ users: data }); } render() { return ( <div> <h1>User List</h1> <ul> {this.state.users.map(user => <li key={user.id}>{user.name}</li>)} </ul> </div> ); } }
This class component uses the componentDidMount lifecycle method to call the fetchUsers function after the component is mounted. The component also includes a users state variable that is updated with the data fetched from the external API.
ReactJS Best Practices
As you continue to develop your ReactJS skills, it’s important to follow best practices to ensure that your code is clean, efficient, and maintainable. Here are some best practices to keep in mind:
1. Use Functional Components When Possible
Functional components are simpler and more lightweight than class components. Whenever possible, you should use functional components to reduce the amount of boilerplate code in your application.
2. Use the React Developer Tools
The React Developer Tools is a browser extension that allows you to inspect and debug React components in your application. This tool can be invaluable when you’re trying to understand how your components are working.
3. Avoid Mutating State Directly
When updating state in your ReactJS components, you should avoid mutating state directly. Instead, you should use the setState function to update state in a way that is safe and predictable.
4. Keep Components Small and Focused
To make your code more maintainable, you should try to keep your components small and focused. Each component should be responsible for a specific task or set of tasks.
5. Use React Router for Navigation
React Router is a powerful library for handling navigation in your ReactJS applications. By using React Router, you can create dynamic, single-page applications that allow users to navigate between different views without refreshing the page.
Conclusion
ReactJS is a powerful tool for building dynamic and engaging web applications. By following best practices and continuing to learn and grow as a developer, you can become a ReactJS expert and create amazing applications that will delight your users.
In this comprehensive guide, we’ve covered everything you need to know to get started with ReactJS. We’ve covered setting up your development environment, understanding ReactJS components, using JSX syntax, managing component state, working with lifecycle methods, and following best practices. With this knowledge, you can start building powerful and efficient ReactJS applications that meet your users’ needs.
Table of Contents
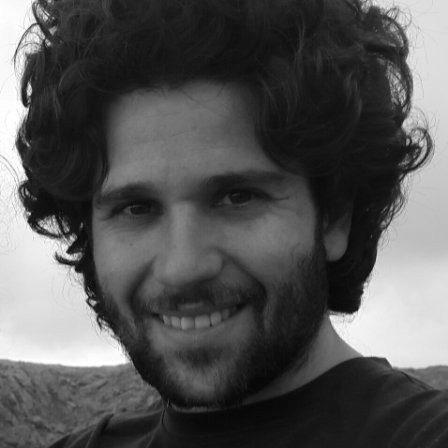
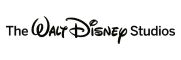