A Practical Guide to Integrating APIs with ReactJS
ReactJS is a popular JavaScript library for building user interfaces, and integrating APIs with ReactJS can be a powerful way to build dynamic and engaging web applications.
Table of Contents
In this blog post, we’ll explore how to integrate APIs with ReactJS, providing a comprehensive guide to building powerful and dynamic applications.
1. Understanding APIs
APIs (Application Programming Interfaces) are a set of protocols, routines, and tools for building software applications. APIs allow developers to access the functionality of external applications, databases, and services, providing a way to share data and functionality across multiple platforms and systems.
APIs can be accessed using HTTP requests, which are typically sent using the GET, POST, PUT, and DELETE methods. These requests are used to retrieve data, submit data, update data, and delete data from the API.
To use an API with ReactJS, you’ll need to use a library that can handle HTTP requests. Two popular options are the built-in Fetch API and the third-party Axios library.
2. Using the Fetch API
The Fetch API is built into modern web browsers and provides a simple and intuitive way to make HTTP requests from within a ReactJS component.
Here’s an example of a component that uses the Fetch API to retrieve data from an external API:
javascript
import React, { useState, useEffect } from 'react'; function UserList() { const [users, setUsers] = useState([]); useEffect(() => { fetch('https://jsonplaceholder.typicode.com/users') .then(response => response.json()) .then(data => setUsers(data)) .catch(error => console.log(error)); }, []); return ( <div> <h1>User List</h1> <ul> {users.map(user => <li key={user.id}>{user.name}</li>)} </ul> </div> ); }
This component uses the useEffect hook to call the Fetch API after the component is mounted. The API returns an array of user objects, which are stored in the component’s state using the useState hook. The component then renders the user data to the screen using JSX.
3. Using the Axios Library
The Axios library is a third-party library that provides a powerful and flexible way to make HTTP requests from within a ReactJS component. Axios provides many features, including automatic request cancellation, progress tracking, and support for authentication.
To use the Axios library with ReactJS, you’ll need to install it using npm and import it into your component. Here’s an example of a component that uses the Axios library to retrieve data from an external API:
javascript
import React, { useState, useEffect } from 'react'; import axios from 'axios'; function UserList() { const [users, setUsers] = useState([]); useEffect(() => { axios.get('https://jsonplaceholder.typicode.com/users') .then(response => setUsers(response.data)) .catch(error => console.log(error)); }, []); return ( <div> <h1>User List</h1> <ul> {users.map(user => <li key={user.id}>{user.name}</li>)} </ul> </div> ); }
This component uses the useEffect hook to call the Axios library after the component is mounted. The library returns an array of user objects, which are stored in the component’s state using the useState hook. The component then renders the user data to the screen using JSX.
4. Handling API Errors
When working with external APIs, it’s important to handle errors effectively. API errors can occur for a variety of reasons, including network issues, server problems, and invalid data.
To handle API errors in ReactJS, you can use a combination of try/catch blocks and error-handling functions.
Here’s an example of a component that handles API errors using the Fetch API:
javascript
import React, { useState, useEffect } from 'react'; function UserList() { const [users, setUsers] = useState([]); const [error, setError] = useState(null); useEffect(() => { fetch('https://jsonplaceholder.typicode.com/users') .then(response => { if (!response.ok) { throw new Error('Network response was not ok'); } return response.json(); }) .then(data => setUsers(data)) .catch(error => setError(error)); }, []); if (error) { return <div>Error: {error.message}</div>; } else { return ( <div> <h1>User List</h1> <ul> {users.map(user => <li key={user.id}>{user.name}</li>)} </ul> </div> ); } }
This component uses a try/catch block to catch any errors that occur during the Fetch API call. If an error occurs, the component updates its state to reflect the error message. The component then conditionally renders the user data or the error message, depending on whether an error occurred.
5. Best Practices for API Integration
When integrating APIs with ReactJS, it’s important to follow best practices for handling asynchronous data and building scalable applications.
Here are some best practices to keep in mind:
- Use state management libraries like Redux or MobX to manage complex state and data flows.
- Use caching mechanisms to avoid making unnecessary API calls and to improve performance.
- Implement error handling to catch and report errors effectively.
- Use pagination to limit the amount of data retrieved from the API and improve performance.
- Implement authentication and security measures to protect user data and prevent unauthorized access.
- Optimize performance by minimizing the amount of data retrieved from the API and reducing unnecessary processing.
6. Conclusion
Integrating APIs with ReactJS can be a powerful way to build dynamic and engaging web applications. By using the Fetch API or the Axios library, you can make HTTP requests from within your ReactJS components and retrieve data from external APIs. When working with APIs, it’s important to handle errors effectively and use best practices for handling asynchronous data. With these skills, you can create amazing ReactJS applications that provide an unparalleled user experience.
Table of Contents
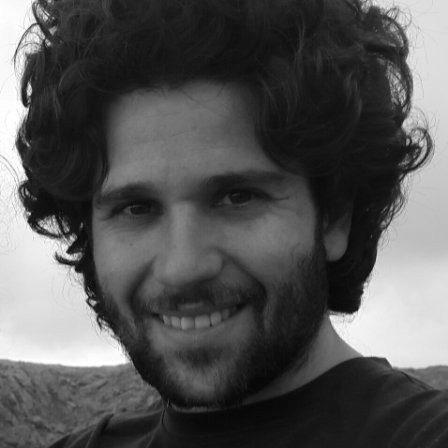
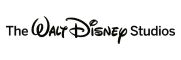