Revamp React: A Guide to Gracefully Handling Errors with useErrorBoundary in Components
Error handling is an essential aspect of building robust and reliable applications. In ReactJS, errors that occur during rendering or in lifecycle methods can lead to component crashes, breaking the user experience. However, React provides a powerful tool called error boundaries to gracefully handle such errors and prevent the entire application from crashing. In this blog post, we will explore the useErrorBoundary hook and learn how to handle errors gracefully in React components.
2
-
Implementing Error Boundaries in React
1.1 Creating the ErrorBoundary Component:
To implement error boundaries in React, we first need to create an ErrorBoundary component. This component acts as a wrapper around other components and captures any errors that occur within its children. Let’s create a basic ErrorBoundary component:
import React, { Component } from 'react'; class ErrorBoundary extends Component { constructor(props) { super(props); this.state = { hasError: false, error: null, errorInfo: null, }; } componentDidCatch(error, errorInfo) { this.setState({ hasError: true, error, errorInfo, }); } render() { if (this.state.hasError) { return ( <div> <h2>Something went wrong.</h2> <p>{this.state.error && this.state.error.toString()}</p> <details style={{ whiteSpace: 'pre-wrap' }}> {this.state.errorInfo.componentStack} </details> </div> ); } return this.props.children; } } export default ErrorBoundary;
1.2. Rendering the Error Boundary:
Once we have our ErrorBoundary component, we can wrap it around other components to create a boundary for handling errors. For example, let’s assume we have a component called Profile that may potentially throw errors. We can wrap it with our ErrorBoundary component as follows:
import React from 'react'; import ErrorBoundary from './ErrorBoundary'; import Profile from './Profile'; function App() { return ( <div> <h1>User Profile</h1> <ErrorBoundary> <Profile /> </ErrorBoundary> </div> ); } export default App;
-
Handling Errors with useErrorBoundary Hook
Introducing useErrorBoundary Hook:
In addition to the traditional class-based approach, React also provides a hook-based solution for error boundaries called useErrorBoundary. This hook allows us to handle errors in functional components using the power of React hooks.
2.1. Installing the required package:
Before we can use the useErrorBoundary hook, we need to install the react-error-boundary package. Open your terminal and run the following command:
npm install react-error-boundary
2.2. Creating a Custom Error Boundary Hook:
Let’s create a custom hook called useErrorBoundary that encapsulates the error boundary logic:
import { useState } from 'react'; import { useErrorHandler } from 'react-error-boundary'; const useErrorBoundary = () => { const [error, setError] = useState(null); const handleError = useErrorHandler(); const handleCatchError = (error) => { setError(error); handleError(error); }; return { error, handleCatchError }; }; export default useErrorBoundary;
Using useErrorBoundary Hook:
2.3. Implementing useErrorBoundary in a Functional Component:
Now that we have our custom useErrorBoundary hook, let’s see how we can implement it in a functional component. Suppose we have a component called Profile that may potentially throw errors. We can use the useErrorBoundary hook to handle errors gracefully:
import React from 'react'; import useErrorBoundary from './useErrorBoundary'; const Profile = () => { const { error, handleCatchError } = useErrorBoundary(); if (error) { return ( <div> <h2>Something went wrong.</h2> <p>{error.toString()}</p> </div> ); } // Rest of the component code return ( <div> {/* Component rendering */} </div> ); }; export default Profile;
2.4. Error Handling with useErrorBoundary:
The useErrorBoundary hook provides us with an error variable and a handleCatchError function. In the example above, if an error occurs within the Profile component, the error will be captured in the error variable, and the component can render an appropriate error message.
2.4. Customizing Error Messages:
With the useErrorBoundary hook, you have the flexibility to customize error messages based on your requirements. You can include additional details or UI components to enhance the user experience when an error occurs.
-
Error Logging in Error Boundaries
3.1 Logging Errors to a Server:
In addition to gracefully handling errors in your application, it’s often crucial to log those errors for debugging and monitoring purposes. You can enhance your error boundary component to log errors to a server using an error logging service or your own server-side logging solution. Here’s an example of logging errors to a server using the fetch API:
componentDidCatch(error, errorInfo) { // Log error to the server fetch('/api/error-logs', { method: 'POST', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify({ error, errorInfo }), }); // Rest of the error handling logic }
3.2. Logging Errors to a Third-Party Service:
Alternatively, you can use third-party error logging services like Sentry or LogRocket to handle error logging. These services provide easy integrations with React applications and offer additional features such as error aggregation and real-time monitoring. Refer to the documentation of your chosen service for specific implementation details.
-
Best Practices for Error Boundaries
4.1. Wrapping Key Components:
It’s generally recommended to wrap key components in your application with error boundaries. By doing so, you ensure that critical parts of your application are protected against unexpected errors, preventing the entire application from crashing. Identify components that are prone to errors or play critical roles in your app and wrap them with error boundaries.
4.2. Handling Async Errors:
Error boundaries primarily handle errors that occur during rendering or in lifecycle methods. For handling errors in async operations like data fetching or API calls, consider using try-catch blocks or promises’ .catch() method. Combine error boundaries with appropriate error handling techniques to cover all types of errors in your application.
4.3. Avoiding Infinite Error Loops:
Be cautious when implementing error boundaries to avoid infinite error loops. If an error occurs within an error boundary while rendering the error boundary itself, it can lead to an infinite loop. Ensure that your error boundary has a proper fallback mechanism or handles such scenarios gracefully to prevent an endless cycle of errors.
-
Error Logging in Error Boundaries
5.1. Logging Errors to a Server:
In addition to gracefully handling errors in your application, it’s often crucial to log those errors for debugging and monitoring purposes. You can enhance your error boundary component to log errors to a server using an error logging service or your own server-side logging solution. Here’s an example of logging errors to a server using the axios library:
import axios from 'axios'; componentDidCatch(error, errorInfo) { // Log error to the server axios.post('/api/error-logs', { error, errorInfo }) .then(response => { // Handle success response }) .catch(err => { // Handle error response }); // Rest of the error handling logic }
5.2. Logging Errors to a Third-Party Service:
Alternatively, you can use third-party error logging services like Sentry or LogRocket to handle error logging. These services provide easy integrations with React applications and offer additional features such as error aggregation and real-time monitoring. Here’s an example of logging errors to Sentry using the @sentry/react package:
import * as Sentry from '@sentry/react'; componentDidCatch(error, errorInfo) { // Log error to Sentry Sentry.captureException(error, { extra: errorInfo }); // Rest of the error handling logic }
-
Best Practices for Error Boundaries
6.1. Wrapping Key Components:
It’s generally recommended to wrap key components in your application with error boundaries. By doing so, you ensure that critical parts of your application are protected against unexpected errors, preventing the entire application from crashing. Identify components that are prone to errors or play critical roles in your app and wrap them with error boundaries.
<ErrorBoundary> <CriticalComponent /> </ErrorBoundary>
6.2. Handling Async Errors:
Error boundaries primarily handle errors that occur during rendering or in lifecycle methods. For handling errors in async operations like data fetching or API calls, consider using try-catch blocks or promises’ .catch() method. Combine error boundaries with appropriate error handling techniques to cover all types of errors in your application.
async function fetchData() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); // Process data } catch (error) { // Handle error } }
6.3. Avoiding Infinite Error Loops:
Be cautious when implementing error boundaries to avoid infinite error loops. If an error occurs within an error boundary while rendering the error boundary itself, it can lead to an infinite loop. Ensure that your error boundary has a proper fallback mechanism or handles such scenarios gracefully to prevent an endless cycle of errors.
class ErrorBoundary extends React.Component { constructor(props) { super(props); this.state = { hasError: false }; } static getDerivedStateFromError(error) { // Update state to render fallback UI return { hasError: true }; } componentDidCatch(error, errorInfo) { // Log error and errorInfo console.log(error, errorInfo); } render() { if (this.state.hasError) { // Fallback UI return <h1>Something went wrong.</h1>; } return this.props.children; } }
-
Conclusion
7.1. Recap of Error Boundary Concepts:
In this blog post, we explored the concept of error boundaries in ReactJS. We discussed two approaches: using the traditional class-based error boundary component and the useErrorBoundary hook. Error boundaries allow us to handle errors gracefully, preventing the entire application from crashing and providing a better user experience.
7.2. Benefits of Graceful Error Handling:
Graceful error handling using error boundaries helps improve the stability and robustness of your React applications. It allows you to catch and handle errors at a component level, isolate error boundaries, and provide fallback UIs or error messages. With error logging, you can monitor and debug errors effectively, ensuring a smoother user experience.
7.3. Final Thoughts:
By leveraging error boundaries in ReactJS, you can enhance the resilience of your applications and create a more user-friendly environment. Whether you choose the class-based approach or the useErrorBoundary hook, make sure to implement error boundaries strategically around critical components and follow best practices for effective error handling.
Table of Contents
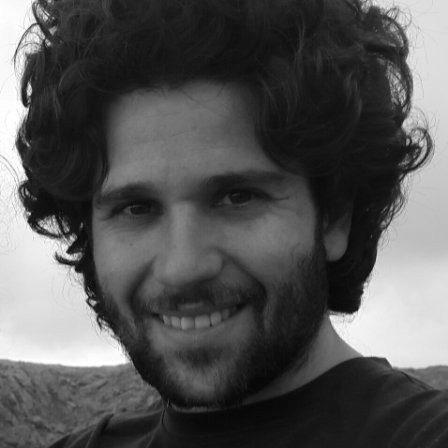
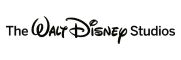