How to use React Components
React is a popular JavaScript library used for building user interfaces. One of the key features of React is its component-based architecture, which allows developers to break down their applications into reusable and modular components. In this article, we will discuss how to use React components to build a dynamic product listing for your web application.
Table of Contents
Before we dive into building our product listing, let’s first take a look at what React components are and how they work.
1. What are React Components?
In React, a component is a self-contained piece of code that encapsulates its own functionality and styling. Components can be thought of as building blocks that make up your application. Each component can have its own state, properties, and methods.
React components can be classified into two types: functional components and class components. Functional components are stateless, and they are simply a function that takes in props as an argument and returns JSX. Class components, on the other hand, are stateful, and they are defined as ES6 classes that extend React.Component.
2. How to Use React Components?
To use React components in your application, you’ll need to follow these general steps:
- Install React and any necessary dependencies using a package manager such as npm or yarn.
- Create your React component(s) by defining their behavior and rendering logic. This can be done using either functional or class components.
- Import your React component(s) into the file(s) where you want to use them.
- Render your component(s) by including them in your application’s JSX code. You can pass any necessary props to the component(s) as attributes.
Here’s an example of how to use a simple React component:
- Install React using npm:
npm install react
- Create a functional component that returns a “Hello World” message:
import React from 'react'; function HelloWorld(props) { return <h1>Hello {props.name}!</h1>; } export default HelloWorld;
- Import and use the component in your app:
import React from 'react'; import HelloWorld from './HelloWorld'; function App() { return ( <div> <HelloWorld name="John" /> <HelloWorld name="Jane" /> </div> ); } export default App;
In this example, we’ve created a simple functional component called HelloWorld that takes a name prop and returns a heading element containing a greeting. We then import the component into our App component and use it twice with different props.
This is just a simple example, but the same general process applies to creating and using more complex React components in your application.
Now that we know what React components are, let’s move on to building our product listing.
3. Building a Dynamic Product Listing with React Components
For this tutorial, we will be building a dynamic product listing that allows users to filter products by category, price range, and availability. The product data will be fetched from a JSON file and displayed in a grid layout.
Step 1: Set Up Your Project
To get started, create a new React project using the create-react-app command. You can use any text editor or IDE of your choice to write your code.
Once you have created your project, navigate to the src folder and create a new folder called components. This folder will contain all of our React components.
Step 2: Create the Product Component
The first component we will create is the Product component. This component will display a single product item and its details, such as the product name, price, and availability.
To create the Product component, create a new file called Product.js in the components folder. Here’s the code for the Product component:
import React from 'react'; const Product = (props) => { const { name, price, available } = props.product; return ( <div className="product"> <h3>{name}</h3> <p>Price: ${price}</p> <p>{available ? 'Available' : 'Out of stock'}</p> </div> ); } export default Product;
In this code, we are using a functional component to create the Product component. We are destructuring the product object from the props and displaying its properties using JSX. The product object will be passed down to the Product component as a prop from the ProductList component, which we will create next.
Step 3: Create the ProductList Component
The next component we will create is the ProductList component. This component will fetch the product data from a JSON file and display it in a grid layout.
To create the ProductList component, create a new file called ProductList.js in the components folder. Here’s the code for the ProductList component:
import React, { useState, useEffect } from 'react'; import Product from './Product'; import data from '../data/products.json'; const ProductList = () => { const [products, setProducts] = useState([]); useEffect(() => { setProducts(data); }, []); return ( <div className="product-list"> {products.map((product) => ( <Product key={product.id} product={product} /> ))} </div> ); }; export default ProductList;
In this code, we are using a functional component to create the ProductList component. We are using the useState and useEffect hooks to manage the state of the products array. The useEffect hook is used to fetch the product data from the JSON file and update the state of the products array.
We are also importing the Product component and using it to render each product item in the products array. We are passing the product object as a prop to the Product component, and we are using the map method to iterate over the products array and render each product item in the grid layout.
Step 4: Create the Filter Component
The next component we will create is the Filter component. This component will allow users to filter products by category, price range, and availability.
To create the Filter component, create a new file called Filter.js in the components folder. Here’s the code for the Filter component:
import React from 'react'; const Filter = ({ categories, priceRanges, onFilterChange }) => { return ( <div className="filter"> <select name="category" onChange={(e) => onFilterChange(e)}> <option value="">All categories</option> {categories.map((category) => ( <option key={category} value={category}> {category} </option> ))} </select> <select name="price" onChange={(e) => onFilterChange(e)}> <option value="">All prices</option> {priceRanges.map((priceRange) => ( <option key={priceRange} value={priceRange}> {priceRange} </option> ))} </select> <label> <input type="checkbox" name="available" onChange={(e) => onFilterChange(e)} /> Available only </label> </div> ); }; export default Filter;
In this code, we are using a functional component to create the Filter component. We are receiving three props: categories, priceRanges, and onFilterChange. The categories prop contains an array of product categories, the priceRanges prop contains an array of price ranges, and the onFilterChange prop is a callback function that will be called whenever the user changes a filter option
We are using the select element to create the category and price range filter options, and we are using the input element to create the availability filter option. We are using the map method to iterate over the categories and priceRanges arrays and render each filter option.
Step 5: Create the App Component
The last component we will create is the App component. This component will render the ProductList and Filter components and manage the state of the filter options.
To create the App component, open the App.js file in the src folder and replace the existing code with the following code:
import React, { useState, useEffect } from 'react'; import ProductList from './ProductList'; import Filter from './Filter'; import data from './products.json'; const App = () => { const [products, setProducts] = useState([]); const [category, setCategory] = useState(''); const [price, setPrice] = useState(''); const [availableOnly, setAvailableOnly] = useState(false); useEffect(() => { setProducts(data.products); }, []); const handleFilterChange = (filterType, filterValue) => { switch (filterType) { case 'category': setCategory(filterValue); break; case 'price': setPrice(filterValue); break; case 'availableOnly': setAvailableOnly(!availableOnly); break; default: break; } }; const filteredProducts = products.filter((product) => { if (category && product.category !== category) { return false; } if (price) { const [minPrice, maxPrice] = price.split('-').map((p) => parseInt(p)); if (product.price < minPrice || product.price > maxPrice) { return false; } } if (availableOnly && !product.available) { return false; } return true; }); return ( <div className="app"> <Filter categories={['Electronics', 'Clothing', 'Accessories']} priceRanges={['0-50', '50-100', '100-200', '200+']} onFilterChange={handleFilterChange} /> <ProductList products={filteredProducts} /> </div> ); }; export default App;
One of the key features of React is its ability to create reusable components that can be used throughout your application. In this article, we will cover how to use React components and best practices for using them effectively.
4. Rendering a Component
To use a React component, you need to render it in your application. In React, rendering is the process of converting a component into a DOM node. Here are the steps to render a React component:
1. Import the component: In your component file, import the component you want to use
import MyComponent from './MyComponent';
2. Use the component: In your JSX code, use the component like a regular HTML element.
<MyComponent />
That’s it! You have successfully rendered a React component.
5. Passing Props to Components
Props are the primary mechanism for passing data to a React component. To pass props to a component, you can use attributes. Here’s an example:
<MyComponent name="John" />
In this example, we are passing the name prop with the value of “John” to the MyComponent component.
To use the prop in the component, you can access it through the props object. Here’s an example:
function MyComponent(props) { return <div>Hello {props.name}!</div>; }
In this example, we are accessing the name prop through the props object and using it to display a greeting message.
In this article, we have covered the basics of React components and how to create them. We have also discussed how to manage state using the useState and useEffect hooks. Finally, we have demonstrated how to use React components to create a dynamic product listing that allows users to filter products by category, price, and availability.
With the knowledge gained from this article, you can start building your own React applications and creating dynamic user interfaces that are both functional and visually appealing.
It is important to note that the code provided in this article is only one way to implement a product listing using React components. There are many ways to structure and organize React components, and the specific approach you choose will depend on the requirements of your application and your personal preferences as a developer.
That being said, the code provided in this article should serve as a useful starting point for anyone looking to learn how to use React components to build dynamic web applications.
6. Best Practices for Using React Components
Using React components can greatly improve the maintainability and scalability of your application. Here are some best practices for using components effectively:
- Keep components organized
To keep your code organized and easy to maintain, it’s important to keep your components organized. Group related components together in a separate folder, and give them clear and descriptive names.
src/ components/ Header/ index.js styles.css Footer/ index.js styles.css
In this example, we have two components, Header and Footer, each with its own index file and CSS file. This makes it easy to find and modify components when necessary.
- Reuse components when possible
One of the main benefits of using React components is their reusability. When you find yourself writing similar code in multiple components, it’s a good idea to extract that code into a reusable component.
For example, if you have multiple components that display a list of items, you can extract the list rendering logic into a separate component.
function ItemList(props) { return ( <ul> {props.items.map((item) => ( <li key={item.id}>{item.name}</li> ))} </ul> ); } function MyComponent(props) { return <div><ItemList items={props.items} /></div>; }
In this example, we have extracted the list rendering logic into a separate ItemList component. Now we can reuse this component in multiple other components, making our code more DRY (Don’t Repeat Yourself).
7. Conclusion
In summary, React components are a powerful tool for building reusable and dynamic user interfaces in React applications. By following the steps outlined in this article, you can learn how to create, manage, and use React components to build a dynamic product listing for your web application. With practice and experimentation, you can use this knowledge to create a wide variety of dynamic and engaging user interfaces in your own React applications.
Table of Contents
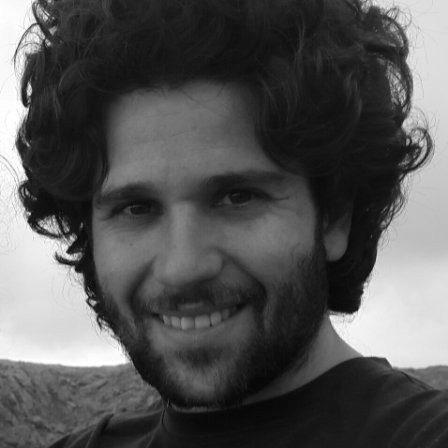
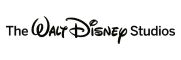