Efficient Event Handling in ReactJS: Debouncing and Throttling with useRef and useCallback
-
Introduction
In today’s fast-paced web applications, optimizing user interactions is crucial for delivering a smooth and responsive user experience. In ReactJS, two powerful techniques for controlling and optimizing the execution of event handlers are debouncing and throttling. By using the useRef and useCallback hooks, developers can easily implement these techniques to improve performance and enhance user interactions.
-
Understanding Debouncing and Throttling
Debouncing and throttling are both techniques used to regulate the frequency of function execution in response to events. While they achieve similar results, they have distinct differences.
Debouncing involves delaying the execution of a function until a specified period of inactivity has occurred. It is often used to handle events that may occur rapidly in succession, such as input field changes or API calls triggered by user input.
Throttling, on the other hand, limits the execution of a function to a specific interval. It ensures that the function is not called more frequently than the specified interval. Throttling is commonly used for events that may fire rapidly, such as scroll or resize events.
The benefits of debouncing and throttling are twofold. Firstly, they improve user experience by preventing excessive or unnecessary function calls. Secondly, they optimize performance by reducing the computational load on the browser.
2.1 Implementing Debouncing with useRef and useCallback
To implement debouncing in ReactJS, we can leverage the useRef and useCallback hooks. Let’s consider an example where we have an input field that triggers a search API call.
First, we create a debounced function using the useRef hook:
import { useRef } from 'react'; const useDebounce = (callback, delay) => { const timeoutRef = useRef(null); const debouncedCallback = useCallback((...args) => { clearTimeout(timeoutRef.current); timeoutRef.current = setTimeout(() => { callback(...args); }, delay); }, [callback, delay]); return debouncedCallback; }; Next, we wrap the original event handler function with the debounced function using the useCallback hook: const SearchInput = () => { const debouncedSearch = useDebounce(search, 300); const handleInputChange = useCallback((event) => { const searchTerm = event.target.value; debouncedSearch(searchTerm); }, [debouncedSearch]); return ( <input type="text" onChange={handleInputChange} /> ); };
In this example, the handleInputChange function will be debounced by 300 milliseconds. The API call will only be triggered once the user has stopped typing or after a 300ms delay.
2.2 Implementing Throttling with useRef and useCallback
Throttling can be implemented using the useRef and useCallback hooks as well. Let’s say we have a button that performs a potentially expensive operation when clicked.
First, we create a throttled function using the useRef hook:
import { useRef } from 'react'; const useThrottle = (callback, delay) => { const timeoutRef = useRef(null); const lastExecTimeRef = useRef(0); const throttledCallback = useCallback((...args) => { const currentTime = Date.now(); if (currentTime - lastExecTimeRef.current > delay) { callback(...args); lastExecTimeRef.current = currentTime; } }, [callback, delay]); return throttledCallback; };
Next, we wrap the original event handler function with the throttled function using the useCallback hook:
const ExpensiveOperationButton = () => { const performExpensiveOperation = () => { // Expensive operation code goes here }; const throttledClick = useThrottle(performExpensiveOperation, 1000); return ( <button onClick={throttledClick}>Perform Operation</button> ); };
In this example, the performExpensiveOperation function will be throttled, allowing it to be called at most once every 1000 milliseconds. This prevents the function from being executed too frequently, optimizing performance.
2.3 Choosing between Debouncing and Throttling
When deciding between debouncing and throttling, it’s important to consider the specific requirements of your application and user interactions.
Debouncing is typically more suitable when handling events that occur rapidly in succession, such as input field changes or autocomplete suggestions. It ensures that the associated function is executed only after a certain period of inactivity, reducing unnecessary function calls.
Throttling is more appropriate for events that may fire rapidly, but still require some level of responsiveness. Examples include scroll or resize events. Throttling limits the function execution to a specific interval, preventing it from being called too frequently.
It’s crucial to assess the impact of delaying or limiting function execution on your application’s user experience. Choosing the right technique will depend on striking a balance between responsiveness and performance.
2.4 Performance Considerations and Best Practices
While debouncing and throttling can significantly improve performance, they may introduce certain trade-offs that need to be considered.
Debouncing can introduce a delay in function execution, which may lead to a perceived lack of responsiveness. Users may experience a slight lag before the expected action takes place. It’s important to fine-tune the delay time to strike the right balance between responsiveness and performance.
Throttling, on the other hand, ensures that the function is executed at regular intervals, potentially delaying updates. For example, if a throttled function is updating a UI component, the updates may not be as frequent as desired. It’s crucial to consider the frequency and urgency of updates in such cases.
To optimize the usage of debouncing and throttling techniques, consider the following best practices:
Determine an appropriate delay or interval time based on the specific use case and user expectations.
Test and fine-tune the timing to achieve the desired balance between responsiveness and performance.
Use appropriate cleanup mechanisms, such as the cleanup function in useEffect, to handle unmounting and clean up any pending timeouts or intervals.
-
Additional Techniques for Optimization
In addition to debouncing and throttling, there are other techniques you can employ to further optimize your ReactJS applications. Let’s explore some of these techniques and how they can contribute to better performance and user experience.
3.1 Memoization with useMemo
Memoization is a technique that involves caching the results of expensive function calls and reusing them when the inputs remain the same. In ReactJS, you can utilize the useMemo hook to memoize values and prevent unnecessary recalculations.
import { useMemo } from 'react'; const ExpensiveComponent = () => { const expensiveValue = useMemo(() => { // Expensive calculation return performExpensiveCalculation(); }, [inputValue]); return <div>{expensiveValue}</div>; };
By wrapping the expensive calculation inside the useMemo hook and providing the relevant dependencies, React will only recompute the value if any of the dependencies change. This optimization technique can be particularly useful when dealing with computationally heavy operations or complex data transformations.
3.2 Lazy Loading with React.lazy and Suspense
Lazy loading is a technique that allows you to load components or assets on-demand, improving initial load times and reducing the amount of resources required upfront. React provides the React.lazy function, along with the Suspense component, to facilitate lazy loading.
import React, { lazy, Suspense } from 'react'; const LazyLoadedComponent = lazy(() => import('./LazyLoadedComponent')); const App = () => { return ( <Suspense fallback={<div>Loading...</div>}> <LazyLoadedComponent /> </Suspense> ); };
In this example, the LazyLoadedComponent is only loaded when it’s actually needed, such as when it’s rendered on the screen. The Suspense component displays a fallback UI while the component is being loaded asynchronously. This technique helps optimize the initial load time of your application and improves the overall perceived performance.
3.3 Virtualization with react-virtualized
Virtualization is a technique used to render large lists or grids efficiently by rendering only the visible portion of the list, instead of rendering every item. The react-virtualized library provides components and utilities to implement virtualization in ReactJS.
import { List } from 'react-virtualized'; const MyVirtualizedList = () => { const rowRenderer = ({ index, key, style }) => { // Render individual list item return ( <div key={key} style={style}> Item {index} </div> ); }; return ( <List width={300} height={500} rowCount={10000} rowHeight={50}rowRenderer={rowRenderer} /> ); };
By using the List component from react-virtualized, you can efficiently render a large number of items by rendering only the visible ones. This approach improves the performance of rendering lists with thousands of items and reduces the memory footprint of your application.
3.4 Code Splitting with React.lazy and React Router
Code splitting is another technique that can significantly improve the performance of your ReactJS applications. It involves splitting your application’s code into smaller, more manageable chunks, which are then loaded on-demand when needed. React provides the React.lazy function in combination with React Router to implement code splitting.
In this example, the components Home, About, and Contact are lazy-loaded using the React.lazy function. When a user navigates to a specific route, the corresponding component is loaded on-demand, resulting in faster initial page loads and improved performance.
3.5 Performance Monitoring and Optimizations
To ensure that your ReactJS application is performing optimally, it’s crucial to monitor its performance and identify potential bottlenecks. Here are some tools and techniques you can use for performance monitoring and optimizations:
- React DevTools: React DevTools is a browser extension that allows you to inspect and debug React component hierarchies. It provides insights into the rendering and update performance of your components, helping you identify any unnecessary re-renders or inefficient rendering patterns.
- Chrome DevTools: Chrome DevTools is a powerful set of developer tools built into the Chrome browser. It provides performance profiling features that allow you to analyze your application’s runtime performance, identify slow functions, and optimize them for better performance.
- Performance Budgeting: Establishing performance budgets helps set constraints on the size and performance impact of your application. By defining limits for factors such as the overall bundle size, network requests, and rendering performance, you can ensure that your application remains performant and delivers a smooth user experience.
- Code Optimization: Analyze your code for potential optimizations. Look for areas where you can reduce unnecessary computations, eliminate redundant code, or optimize algorithms. Minify and compress your code to reduce its size and improve loading times.
- Network Optimization: Optimize network requests by minimizing the number of requests, reducing the size of assets, and leveraging caching techniques. Use tools like webpack to bundle and optimize your assets, and consider using Content Delivery Networks (CDNs) to serve static assets closer to your users.
-
Conclusion
In this blog post, we explored various techniques and strategies for optimizing ReactJS applications. We started with debouncing and throttling, which help control the execution of event handlers and improve performance. We then discussed additional optimization techniques such as memoization, lazy loading, virtualization, code splitting, and performance monitoring.
By implementing these techniques in your ReactJS applications, you can significantly enhance performance, reduce unnecessary computations, and deliver a smooth and responsive user experience. Remember to carefully evaluate the specific requirements of your application and choose the appropriate optimization techniques accordingly.
Continuously monitor and profile your application’s performance, and be proactive in identifying and addressing any potential performance bottlenecks. Regularly optimize and refine your code, network requests, and asset delivery to ensure your application remains performant as it scales.
By combining these optimization techniques with best practices in ReactJS development, you can create high-performing applications that delight users and leave a lasting positive impression. Happy optimizing!
Table of Contents
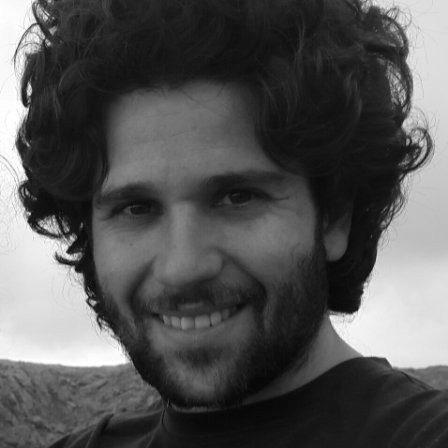
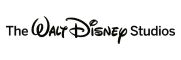