Redefining User Experience: Implementing Lazy Loading and Infinite Scrolling with ReactJS’s useIntersectionObserver Hook
This guide explores the usage of the `useIntersectionObserver` hook in ReactJS for building high-performing web applications. Leveraging Lazy Loading and Infinite Scrolling techniques, it aims to improve user experience by enhancing speed and efficiency. Ideal for both beginners and seasoned developers, this guide provides practical insights on how to optimize app performance by only loading content when necessary.
ReactJS and Intersection Observer API
ReactJS, known for its component-based architecture and efficient handling of state, has gained wide acceptance among developers for building interactive user interfaces. Alongside these powerful features, it allows developers to seamlessly interact with various Web APIs, one of which is the Intersection Observer API.
The Intersection Observer API provides a way to asynchronously observe changes in the intersection of a target element with an ancestor element or with a top-level document’s viewport. This is particularly useful for implementing any form of “lazy loading” mechanism, or to report visibility of elements in order to react accordingly.
In ReactJS, to make the Intersection Observer API more accessible and easy to use, developers often create custom hooks, one of which is `useIntersectionObserver`. This hook abstracts the logic of setting up and tearing down observers, making it a breeze to utilize the API’s capabilities within a React component.
`useIntersectionObserver` in React simplifies dealing with intersections, allowing you to monitor when an element enters or leaves the viewport. With this tool at your disposal, you can create efficient, high-performing applications, implementing features like lazy loading of images or components, infinite scrolling in lists, or triggering animations or fetch requests based on the element’s visibility. It opens up new horizons for efficient loading and handling of page components, enhancing the user experience on your web application.
Lazy Loading in ReactJS
Lazy loading is a design pattern in which content is loaded only when it is needed or when it enters the viewport. This can significantly enhance performance, especially for applications with heavy media content such as images, videos, or large datasets, as it reduces the initial load time of the application.
In the context of ReactJS, lazy loading can be applied to components, images, or any other type of content that might take significant resources to load.
Here is how you can implement lazy loading in ReactJS with the `useIntersectionObserver` hook:
- Initializing useIntersectionObserver hook:
First, you need to set up the `useIntersectionObserver` hook. This hook takes a callback function, which will be invoked whenever the target element intersects with the viewport.
- Setting up the components to be lazy loaded:
Let’s say you want to lazy load an image. You would set up an `img` component with a placeholder image initially. You will replace this placeholder with the actual image once the component enters the viewport.
- Utilizing useIntersectionObserver for lazy loading:
This is where the magic happens. You will use the `useIntersectionObserver` hook on the placeholder image. When the placeholder image comes into the viewport, the callback function passed to `useIntersectionObserver` will be invoked. In this function, you can set the state of the image source to switch from the placeholder to the actual image.
This way, the actual image is only loaded when it is needed, saving valuable resources and reducing the initial loading time of the web application. Lazy loading with `useIntersectionObserver` is a powerful tool to enhance your ReactJS applications’ performance.
Infinite Scrolling in ReactJS
Infinite scrolling is a popular web design pattern used in many applications where continuous content must be loaded smoothly and seamlessly, such as social media feeds, e-commerce product listings, or any long list of data entries. The primary advantage of infinite scrolling is the elimination of traditional pagination and providing users with a frictionless content consumption experience.
In ReactJS, this functionality can be efficiently implemented using the `useIntersectionObserver` hook. Here’s a step-by-step guide on how to do it:
- Initializing useIntersectionObserver hook:
Start by setting up the `useIntersectionObserver` hook. This hook will trigger a callback function whenever a specified “sentinel” element intersects with the viewport.
- Setting up the scrolling component:
Design your component such that data is loaded in batches, with a “Load More” button or a sentinel div at the end. This sentinel serves as the trigger point for loading more data. Initially, it can be hidden from the user’s view.
- Utilizing useIntersectionObserver for infinite scrolling:
Apply the `useIntersectionObserver` hook on the sentinel. When the sentinel enters the viewport, the callback function will be triggered. This function should contain the logic to fetch the next batch of data from your server and append it to your current data list.
Through these steps, you’re able to create an infinite scrolling experience. As the user scrolls down, new data is fetched and displayed, creating a seamless browsing experience. This mechanism, powered by the `useIntersectionObserver` hook in ReactJS, makes handling infinite scrolling a lot more manageable and efficient.
Best Practices and Troubleshooting
While implementing lazy loading and infinite scrolling in ReactJS with `useIntersectionObserver` can greatly enhance your web application’s performance, it’s crucial to follow some best practices and be aware of potential issues. Here are some suggestions for best practices and troubleshooting:
Best practices
- Fallback for Non-Supporting Browsers: Though Intersection Observer is widely supported, some older browsers or versions may not support it. Ensure you have a fallback mechanism in place for these scenarios.
- Unobserve When Not Needed: Be sure to unobserve elements when you no longer need them. This can be done using the disconnect method or unobserve method.
- Prevent Excessive Fetching: For infinite scrolling, add logic to prevent data fetching while a fetch request is already in progress. This prevents unnecessary and redundant requests.
- Provide an Escape Hatch: Though infinite scrolling provides a seamless experience, it can sometimes make navigation difficult. Provide a way for users to jump to specific content or offer a ‘Back to Top’ button.
- Use Placeholder: While implementing lazy loading, use a placeholder of the same dimensions as your content to prevent layout shifts when the actual content loads.
Troubleshooting:
- Callback Not Triggered: If your callback isn’t being called, check to make sure that your target and root elements are set up correctly. Also, verify that your target element is actually intersecting with the viewport or the root element at some point.
- Performance Issues: If you face performance issues, check the number of elements being observed. Observing too many elements might lead to performance degradation.
- Content Not Loading Properly: If your content doesn’t load or display correctly, ensure the logic within your callback function is correctly fetching and rendering your data.
Understanding these best practices and common issues can help you effectively use `useIntersectionObserver` in ReactJS for lazy loading and infinite scrolling, leading to enhanced web application performance.
Conclusion
The utilization of `useIntersectionObserver` in ReactJS is a powerful tool for enhancing web application performance and user experience by implementing Lazy Loading and Infinite Scrolling. Understanding the Intersection Observer API and effectively applying it can transform content delivery on a webpage. However, adhering to best practices and being aware of potential issues is crucial for effective usage. Regardless of the level of expertise, integrating these techniques into applications can lead to significant improvements in performance and user experience.
Happy coding!
Table of Contents
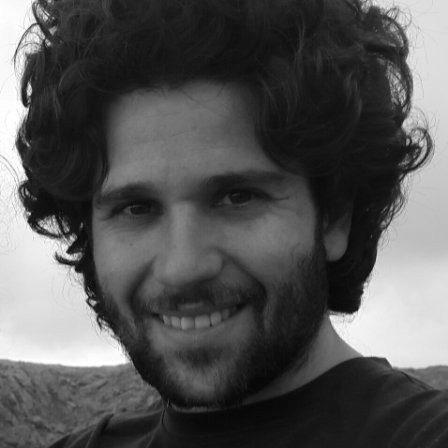
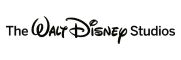