What is lazy loading and how is it implemented?
Lazy loading is a performance optimization technique where specific components or assets are loaded only when they’re required, instead of being loaded upfront during the initial page load. This approach can significantly speed up the initial rendering of web applications by reducing the amount of JavaScript that needs to be fetched and executed at the start.
In the context of React, lazy loading typically refers to deferring the loading of certain React components until they are needed. This can be particularly beneficial for large applications with multiple routes, where users might not visit every page or use every component during a single session.
React has built-in support for component-level lazy loading through the `React.lazy()` function. This function allows you to render a dynamic import of a component as a regular component. When a component is loaded using `React.lazy()`, it is automatically split into a separate bundle by bundlers like Webpack. This new bundle is then fetched only when the component is about to be rendered for the first time.
Here’s a basic outline of implementing lazy loading in React:
- Dynamic Import: Use the `import()` syntax to dynamically import a component.
- React.lazy: Wrap the dynamic import with `React.lazy()`.
- Suspense: Since the component load might be asynchronous, use the `Suspense` component to specify a fallback UI that will be displayed while the component is being loaded.
It’s worth noting that `React.lazy` currently only supports default exports. Also, for server-side rendering scenarios, you might need additional libraries or strategies, as `React.lazy` and `Suspense` are not yet optimized for server-side rendering out-of-the-box.
Lazy loading in React allows developers to improve the perceived performance and efficiency of their applications. By ensuring that users download only the code they need when they need it, you can offer a faster and more responsive user experience.
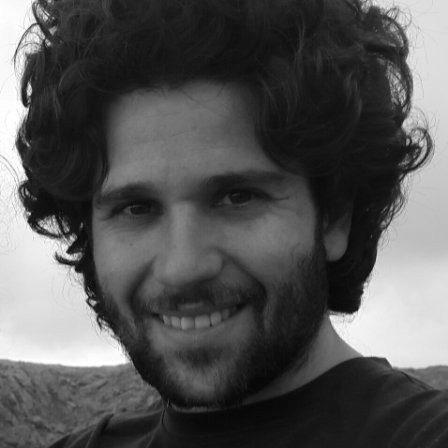
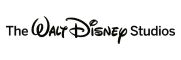