Managing State and Side Effects with a Modern Approach: Hooks in ReactJS
ReactJS is a popular JavaScript library for building user interfaces, and Hooks are a new addition to ReactJS that provide a simpler way to manage state and side effects in your applications.
Table of Contents
In this blog post, we’ll explore Hooks in ReactJS and how they can be used to manage state and side effects.
1. Introduction to Hooks
Hooks are functions that let you “hook into” ReactJS state and lifecycle features from function components. They are a new addition to ReactJS as of version 16.8 and provide a simpler way to manage state and side effects in your applications.
There are several built-in Hooks in ReactJS, including:
- useState: Lets you use state in a function component.
- useEffect: Lets you perform side effects in a function component.
- useContext: Lets you use context in a function component.
- useReducer: Lets you use the reducer pattern to manage state in a function component.
- useCallback: Lets you memoize functions in a function component.
- useMemo: Lets you memoize values in a function component.
- useRef: Lets you create a mutable value that persists across renders in a function component.
1.1 Using useState Hook
The useState Hook lets you use state in a function component. It takes an initial value as an argument and returns an array with two elements: the current state and a function to update the state. Here’s an example:
javascript
import React, { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); const handleClick = () => { setCount(count + 1); }; return ( <div> <p>Count: {count}</p> <button onClick={handleClick}>Increment</button> </div> ); }
In this example, we define a Counter component that uses the useState Hook to manage a count state. We define a handleClick function that updates the count state when the button is clicked, and we render the count state and a button that triggers the handleClick function.
1.2 Using useEffect Hook
The useEffect Hook lets you perform side effects in a function component. It takes a function as an argument that will be executed after every render of the component. Here’s an example:
javascript
import React, { useState, useEffect } from 'react'; function Counter() { const [count, setCount] = useState(0); useEffect(() => { document.title = `Count: ${count}`; }); const handleClick = () => { setCount(count + 1); }; return ( <div> <p>Count: {count}</p> <button onClick={handleClick}>Increment</button> </div> ); }
In this example, we define a Counter component that uses the useEffect Hook to update the document title with the current count state after every render of the component. We define a handleClick function that updates the count state when the button is clicked, and we render the count state and a button that triggers the handleClick function.
1.3 Using other Hooks
The other built-in Hooks in ReactJS can also be useful for managing state and side effects in your applications. useContext lets you consume context in a function component, useReducer lets you use the reducer pattern to manage state, useCallback lets you memoize functions, useMemo lets you memoize values, and useRef lets you create a mutable value that persists across renders.
Here’s an example of using the useReducer Hook:
javascript
import React, { useReducer } from 'react'; const initialState = { count: 0 }; function reducer(state, action) { switch (action.type) { case 'increment': return { count: state.count + 1 }; case 'decrement': return { count: state.count - 1 }; default: throw new Error(); } } function Counter() { const [state, dispatch] = useReducer(reducer, initialState); return ( <div> <p>Count: {state.count}</p> <button onClick={() => dispatch({ type: 'increment' })}>Increment</button> <button onClick={() => dispatch({ type: 'decrement' })}>Decrement</button> </div> ); }
In this example, we define a Counter component that uses the useReducer Hook to manage a count state using the reducer pattern. We define an initialState object and a reducer function that handles the state updates based on the dispatched action. We render the count state and two buttons that dispatch the increment and decrement actions.
2. Benefits of Hooks
Using Hooks in your applications can provide many benefits, such as improved code readability, reusability, and performance. Hooks also allow for better separation of concerns, making it easier to maintain and test your code.
When using Hooks, it’s important to follow the rules of Hooks. Some of the main rules include:
- Only call Hooks at the top level of your function component or custom Hooks.
- Only call Hooks from React function components or custom Hooks.
- Only call Hooks in the same order on every render of your component.
By following these rules, you can ensure that your code is safe and predictable and that your Hooks work correctly.
3. Conclusion
Hooks in ReactJS provide a simpler way to manage state and side effects in your applications. By using Hooks, you can avoid class components and make your code more concise and readable.
In this blog post, we explored Hooks in ReactJS and how they can be used to manage state and side effects. We covered the basics of the useState and useEffect Hooks, and briefly mentioned some of the other built-in Hooks in ReactJS. We also showed an example of using the useReducer Hook.
If you haven’t already, give Hooks a try in your next ReactJS project and see how they can improve your development experience.
Table of Contents
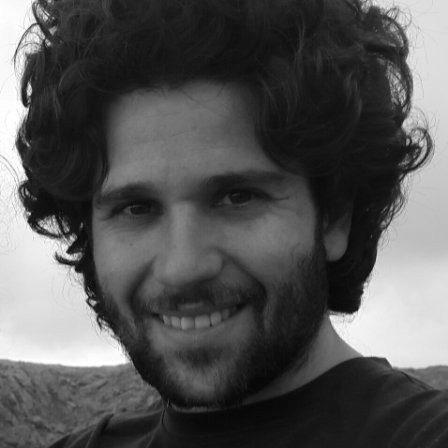
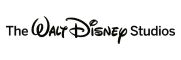