Developing Multi-Language Applications with ReactJS Localization
In today’s increasingly interconnected world, it’s essential for web applications to cater to users from diverse linguistic and cultural backgrounds. Localization, the process of adapting an application’s interface and content to different languages and regions, plays a crucial role in creating inclusive and accessible web applications.
Table of Contents
In this blog post, we’ll explore localization in ReactJS, discuss popular libraries for building multi-language applications, and provide a step-by-step guide on implementing localization in your React projects.
1. Choosing a Localization Library for ReactJS
There are several libraries available for handling localization in ReactJS applications. Some popular options include:
- i18next: A powerful, feature-rich, and extensible internationalization framework that provides an easy-to-use API for handling translations, pluralization, and more.
- react-intl: A library built on top of the ECMAScript Internationalization API that simplifies handling translations, number formatting, and date and time formatting.
- react-i18nify: A lightweight and straightforward library for implementing localization in your React applications with minimal configuration.
For the purpose of this blog post, we’ll focus on i18next due to its extensive features and flexibility.
2. Setting Up Localization in Your React Application
Follow these steps to set up i18next in your React application:
1. Install the necessary dependencies:
bash
npm install i18next react-i18next
2. Create a folder named locales in your src directory to store your translations.
Inside the locales folder, create subdirectories for each language you want to support, and inside each language folder, create a JSON file named translation.json containing your translations.
For example:
css
src ??? locales ??? en ? ??? translation.json ??? es ??? translation.json
3. In each translation.json file, add your translations as key-value pairs. For example:
json
// en/translation.json { "welcome": "Welcome to our application!", "signIn": "Sign In" } // es/translation.json { "welcome": "¡Bienvenido a nuestra aplicación!", "signIn": "Iniciar sesión" }
4. Create a file named i18n.js in your src folder to configure i18next.
javascript
import i18n from "i18next"; import { initReactI18next } from "react-i18next"; import enTranslations from "./locales/en/translation.json"; import esTranslations from "./locales/es/translation.json"; i18n .use(initReactI18next) .init({ resources: { en: { translation: enTranslations, }, es: { translation: esTranslations, }, }, lng: "en", fallbackLng: "en", interpolation: { escapeValue: false, }, }); export default i18n;
5. Import and initialize i18next in your application’s entry point (index.js).
javascript
import "./i18n"; // ...
6. Now you can use the useTranslation hook and the t function provided by react-i18next to translate your application content.
javascript
import React from "react"; import { useTranslation } from "react-i18next"; const App = () => { const { t } = useTranslation(); return ( <div> <h1>{t("welcome")}</h1> <button>{t("signIn")}</button> </div> ); }; export default App; ``
3. Implementing Language Switching
To allow users to switch between languages, follow these steps:
1. Create a language switcher component that allows users to select their preferred language.
javascript
import React from "react"; import { useTranslation } from "react-i18next"; const LanguageSwitcher = () => { const { i18n } = useTranslation(); const handleChange = (event) => { i18n.changeLanguage(event.target.value); }; return ( <select value={i18n.language} onChange={handleChange}> <option value="en">English</option> <option value="es">Español</option> </select> ); }; export default LanguageSwitcher;
2. Add the LanguageSwitcher component to your application, allowing users to switch between languages.
javascript
import React from "react"; import { useTranslation } from "react-i18next"; import LanguageSwitcher from "./LanguageSwitcher"; const App = () => { const { t } = useTranslation(); return ( <div> <LanguageSwitcher /> <h1>{t("welcome")}</h1> <button>{t("signIn")}</button> </div> ); }; export default App;
4. Handling Plurals, Dates, and Number Formatting
i18next provides built-in support for handling plural forms, date and time formatting, and number formatting. To leverage these features, follow these steps:
1. Update your translation.json files to include plural forms of words or phrases.
json
// en/translation.json { "item": "item", "item_plural": "items" } // es/translation.json { "item": "artículo", "item_plural": "artículos" }
2. Use the t function to handle plurals in your components.
javascript
const { t } = useTranslation(); const itemCount = 5; return ( <div> <p>{t("item", { count: itemCount })}</p> </div> );
3. For date and number formatting, you can use the native Intl JavaScript API or third-party libraries like date-fns or moment.
5. Conclusion
Localization is crucial for creating inclusive, accessible, and user-friendly web applications. By leveraging ReactJS and powerful libraries like i18next, you can easily build multi-language applications that cater to users from diverse linguistic and cultural backgrounds. Following this guide will help you implement localization in your React projects, providing a better user experience for your global audience.
Table of Contents
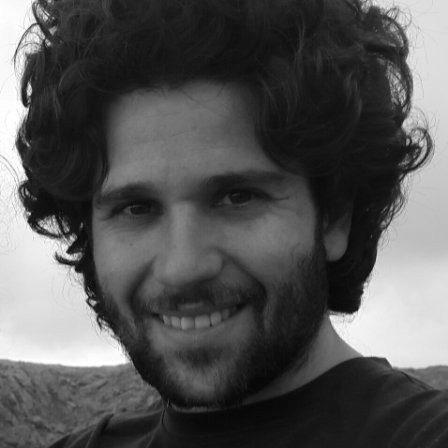
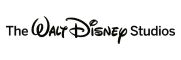