React Native for Mobile Development with ReactJS
Mobile applications are becoming increasingly important for businesses and developers alike. React Native is a popular framework that allows developers to build mobile applications using the same principles and tools as ReactJS.
In this blog post, we’ll explore what React Native is, the benefits of using React Native for mobile development, and how to get started building a React Native application.
1. Understanding React Native
React Native is an open-source framework for building mobile applications using JavaScript and ReactJS. It allows developers to write mobile applications that can run on both iOS and Android devices, using a single codebase. React Native accomplishes this by translating ReactJS components into native UI components, providing a fast and responsive user experience.
2. Benefits of Using React Native for Mobile Development
There are several benefits to using React Native for mobile development, including:
- Cross-platform compatibility: React Native allows you to build applications that can run on both iOS and Android devices, reducing development time and costs.
- Reusability: React Native components can be reused across different applications and platforms, providing a more efficient development process.
- Performance: React Native provides fast and responsive UI, thanks to its ability to translate ReactJS components into native UI components.
- Community support: React Native has a large and active community, with many resources and tools available for developers.
3. Getting Started with React Native
To get started with React Native, you’ll need to set up your development environment and create a new React Native project. Here’s how:
- Install the React Native CLI:
npm install -g react-native-cli
- Create a new React Native project:
react-native init MyReactNativeApp
- Start the development server:
cd MyReactNativeApp react-native start
- Run your application on an iOS or Android emulator or device:
react-native run-ios react-native run-android
4. Building a React Native Application
Once you’ve set up your React Native project, you can start building your mobile application. Here’s an example of how to create a basic React Native component:
import React from "react"; import { StyleSheet, Text, View } from "react-native"; const App = () => { return ( <View style={styles.container}> <Text>Hello, React Native!</Text> </View> ); }; const styles = StyleSheet.create({ container: { flex: 1, justifyContent: "center", alignItems: "center", }, }); export default App;
5. Adding Functionality to Your React Native Application
To add functionality to your React Native application, you can use third-party libraries and APIs, just like you would in a traditional ReactJS application. Here’s an example of how to use the fetch API to fetch data from an API:
import React, { useEffect, useState } from "react"; import { StyleSheet, Text, View } from "react-native"; const App = () => { const [data, setData] = useState([]); useEffect(() => { fetch("https://jsonplaceholder.typicode.com/todos") .then((response) => response.json()) .then((json) => setData(json)) .catch((error) => console.error(error)); }, []); return ( <View style={styles.container}> <Text>Number of todos: {data.length}</Text> </View> ); }; const styles = StyleSheet.create({ container: { flex: 1, justifyContent: "center", alignItems: "center", }, }); export default App;
6. Conclusion
React Native allows developers to build mobile applications using the same principles and tools as ReactJS, providing cross-platform compatibility, reusability, and fast performance. By following the key concepts and tools outlined in this blog post, you’ll be well on your way to building your own mobile application with React Native. With its large and active community, the possibilities for creating a seamless and powerful user experience are endless.
Table of Contents
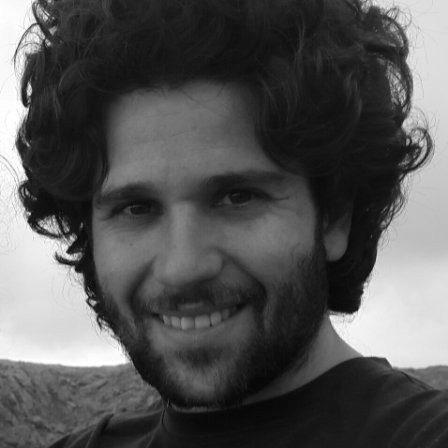
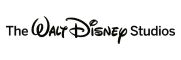