How to optimize React applications?
React applications, by default, offer commendable performance for most use cases. However, as applications grow in complexity or scale, targeted optimizations become essential to maintain responsiveness and efficiency.
Firstly, virtualization can be a game-changer for lists or grids with vast numbers of items. Libraries like `react-window` render only the items currently in view, dramatically reducing the rendering cost for large lists.
Code splitting, facilitated by tools like React’s built-in `React.lazy()` and the dynamic `import()` syntax, ensures that only the necessary code chunks are loaded for a given route or component, speeding up initial load times. Pair this with the `<Suspense>` component to offer fallback content while the component is being loaded.
Memoization can be a powerful technique to avoid unnecessary recalculations. React offers the `useMemo` hook for values and `useCallback` for functions to ensure that they’re only recomputed or recreated when specific dependencies change.
To avoid unnecessary re-renders, you can utilize `React.memo()` for functional components. It’s a higher-order component that ensures your component only re-renders when its props change. For class components, consider implementing `shouldComponentUpdate` or extending `PureComponent` which does a shallow comparison of state and props.
State management solutions, like Redux, can sometimes introduce performance bottlenecks. Using selectors (like those from `reselect`) ensures that components re-render only when the necessary slice of state changes.
Lastly, don’t overlook the basics: keeping packages updated, using production builds when deploying, and utilizing the React DevTools ‘ Profiler to analyze component re-renders and performance bottlenecks.
Optimizing a React application requires a mix of React-specific strategies and general web performance practices. Regular profiling and staying attuned to user feedback can guide your optimization efforts in the right direction, ensuring a responsive and seamless application experience.
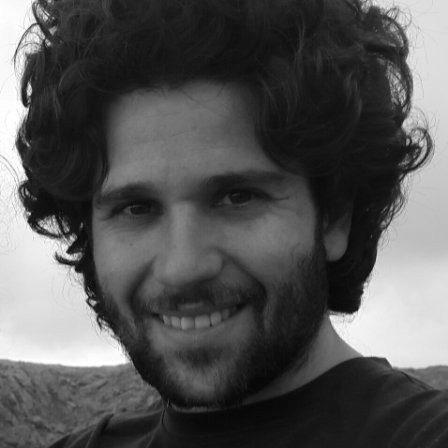
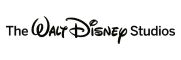