Passing Data between Components in ReactJS using Props
ReactJS is a popular JavaScript library for building user interfaces. One of the most important concepts in ReactJS is component communication, which refers to the process of passing data between components
Table of Contents
In this blog post, we’ll explore how to use ReactJS props to pass data between components in your applications.
1. Introduction to ReactJS Props
ReactJS props, short for properties, are a way to pass data from one component to another in ReactJS. Props are read-only and cannot be modified by the child component that receives them. Instead, the parent component that creates the child component sets the props.
Here’s an example of how to use props to pass data between two components:
javascript
function Welcome(props) { return <h1>Hello, {props.name}!</h1>; } function App() { return <Welcome name="Alice" />; }
In this example, we define a Welcome component that accepts a name prop and uses it to display a greeting. We then use the Welcome component inside an App component and pass the name prop with the value of “Alice”.
2. Passing Props
There are two ways to pass props to a component in ReactJS:
2.1 Inline Props
You can pass props to a component inline by setting them as attributes. For example:
javascript
function App() { return <Welcome name="Alice" />; }
In this example, we pass the name prop with the value of “Alice” to the Welcome component.
2.2 Object Props
You can also pass props as an object. This is useful when you have multiple props to pass or when you want to reuse the same set of props in multiple places. For example:
javascript
function App() { const person = { name: "Alice", age: 25 }; return <Welcome person={person} />; }
In this example, we define a person object with the name and age properties and pass it to the Welcome component as the person prop.
3. Accessing Props
To access props inside a component, you can use the props object that is passed as an argument to the component function. For example:
javascript
function Welcome(props) { return <h1>Hello, {props.name}!</h1>; }
In this example, we access the name prop using props.name and use it to display a greeting.
4. Using Props in Child Components
Props can also be passed down to child components. This allows you to create a tree-like structure of components that each receive data from their parent component. Here’s an example:
javascript
function Welcome(props) { return <h1>Hello, {props.name}!</h1>; } function App() { return ( <div> <Welcome name="Alice" /> <Welcome name="Bob" /> </div> ); }
In this example, we define a Welcome component that displays a greeting using the name prop. We then use the Welcome component twice inside the App component with different values for the name prop.
5. Passing Functions as Props
In addition to passing data as props, you can also pass functions as props. This allows you to create reusable components that can trigger actions in the parent component. Here’s an example:
javascript
function Button(props) { return <button onClick={props.onClick}>{props.label}</button>; } function App() { const handleClick = () => { console.log("Button clicked"); }; return ( <div> <Button onClick={handleClick} label="Click me!" /> </div> ); } ```
In this example, we define a Button component that accepts an onClick prop and a label prop. We then define a handleClick function in the App component and pass it as the onClick prop to the Button component.
6. Using Default Props
In some cases, you may want to provide default values for props in case they are not passed from the parent component. You can do this by defining default values for props using the defaultProps property. Here’s an example:
javascript
function Welcome(props) { return <h1>Hello, {props.name}!</h1>; } Welcome.defaultProps = { name: "Stranger" }; function App() { return ( <div> <Welcome /> <Welcome name="Alice" /> </div> ); }
In this example, we define a Welcome component that accepts a name prop. We then define a default value for the name prop using the defaultProps property. We also use the Welcome component twice inside the App component, once without passing the name prop and once with passing the name prop as “Alice”.
7. Conclusion
ReactJS props are a powerful tool for passing data and functions between components in your applications. By using props, you can create a tree-like structure of components that each receive data from their parent component, as well as trigger actions in the parent component. This can help you build more modular and reusable components and improve the organization of your code.
In this blog post, we explored how to use ReactJS props to pass data and functions between components. We covered the basics of passing and accessing props, as well as how to use props in child components, pass functions as props, and use default props. We hope that this guide has been helpful in understanding how to use ReactJS props effectively in your own applications.
Table of Contents
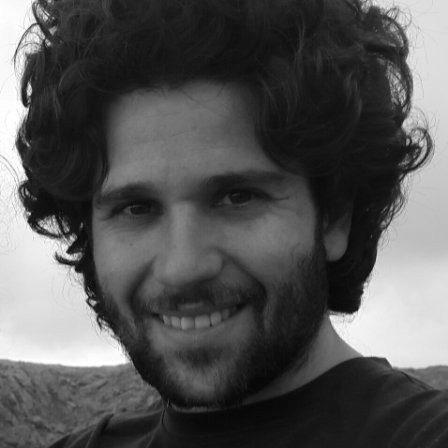
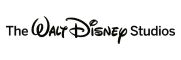