ReactJS Q & A
What are the best practices for performance?
React is known for its efficient reconciliation algorithm, but as applications grow, ensuring optimal performance becomes pivotal. Here are some best practices tailored to enhance React performance:
- Utilize `PureComponent` and `React.memo`: Both these features allow components to prevent unnecessary re-renders by doing a shallow comparison of current and new props or state. If data structures remain unchanged, re-rendering is skipped, conserving computational resources.
- Profile with DevTools: The React DevTools extension provides a Profiler that helps identify bottlenecks and optimize rendering performance. By visualizing component renders, developers can spot and rectify inefficient rendering paths.
- Virtualize Long Lists: When dealing with extensive lists, rendering all items can be wasteful. Libraries like `react-virtualized` or `react-window` enable rendering only the visible items, dramatically improving performance for large lists.
- Optimize Images and Media: Compress and appropriately size media assets. Consider using responsive images or even lazy loading them to ensure that only necessary content is loaded.
- Avoid Inline Functions in Render: Inline functions create a new function instance during every render, potentially causing unnecessary re-renders for child components expecting stable prop references.
- Use Throttling and Debouncing: For events that trigger frequent updates, such as scroll or input events, throttle or debounce to limit the number of times state is updated or effects are run.
- Optimize State Updates: Ensure that state updates are not redundant. Use functional updates with `setState` when the next state relies on the previous one.
- Leverage Code Splitting: Use dynamic imports with React’s lazy and Suspense to split the bundle, delivering only the essential code when a user navigates to a particular route.
- Keep Third-Party Libraries in Check: Periodically audit and profile the impact of third-party libraries. Not all libraries are optimized for performance, and some may become performance bottlenecks.
- Avoid using the Index as a Key: While convenient, using indexes as keys in lists can lead to rendering issues, especially if items get reordered.
While React offers efficient out-of-the-box performance, it’s essential to remain vigilant as applications scale. By adhering to these practices and continuously profiling, developers can ensure a snappy and smooth user experience.
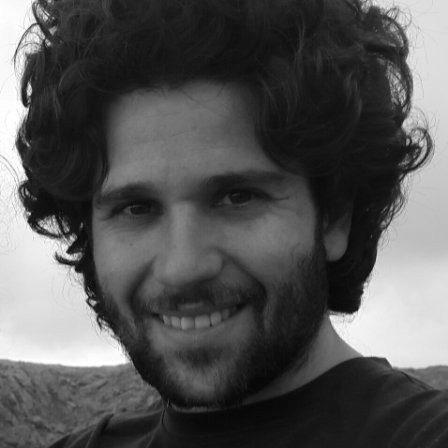
Previously at
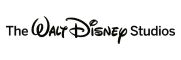
Seasoned Software Engineer specializing in React.js development. Over 5 years of experience crafting dynamic web solutions and collaborating with cross-functional teams.