Optimizing React Component Rendering and Performance with useMemo
-
Introduction
ReactJS is a popular JavaScript library for building user interfaces. One of its key features is the virtual DOM, which efficiently updates and renders components. However, as your application grows, you may encounter performance issues due to unnecessary re-renders. React’s useMemo hook comes to the rescue by providing a way to optimize component rendering and improve overall performance. In this blog post, we will explore the useMemo hook and demonstrate how it can be utilized effectively.
1.1 Understanding the Problem
React’s reconciliation algorithm determines when and how components should be re-rendered. However, if a component re-renders frequently, it can impact the performance of your application. This is particularly problematic when dealing with expensive computations, such as complex data transformations or API calls, which are not necessary on every render.
1.2 Introducing useMemo
The useMemo hook is designed to solve this issue. It allows you to memoize the result of a computation and only recompute it when the dependencies change. By doing so, you can avoid unnecessary computations and improve the performance of your components.
1.3 Syntax and Usage:
The syntax for using useMemo is as follows:
const memoizedValue = useMemo(() => computeExpensiveValue(a, b), [a, b]);
The first argument is a function that performs the expensive computation. The second argument is an array of dependencies. If any of the dependencies change, the computation will be re-executed; otherwise, the memoized value will be returned.
1.4 Optimizing Component Rendering:
Let’s consider a practical example where useMemo can be beneficial. Suppose we have a component that renders a list of users and their corresponding details. The component receives an array of users as a prop. Each time the prop changes, the component re-renders, even if the user data remains the same. This can lead to unnecessary re-renders and performance degradation.
import React from 'react'; const UserList = ({ users }) => { return ( <div> {users.map((user) => ( <UserCard key={user.id} user={user} /> ))} </div> ); }; const UserCard = ({ user }) => { // Expensive computation for user details const userDetails = useMemo(() => { return computeUserDetails(user); }, [user]); return ( <div> <p>{userDetails.name}</p> <p>{userDetails.email}</p> {/* Additional user details */} </div> ); };
In the above example, we wrap the expensive computation of the user details in the useMemo hook. By providing [user] as the dependency array, the computation will only be performed when the user object changes. Otherwise, the memoized value will be returned, preventing unnecessary re-renders.
1.5 Performance Improvements:
Using useMemo can significantly improve the performance of your React components, especially when dealing with computationally intensive operations or large datasets. Let’s look at some statistics to demonstrate the impact of useMemo on rendering optimization.
Consider a scenario where a component renders a list of 1000 items, and each item requires an expensive computation. Without using useMemo, the component would perform the computation for all items on every render, resulting in a sluggish user experience. However, by leveraging useMemo, we can drastically reduce the number of computations and improve rendering performance.
// Without useMemo Render time: 300ms // With useMemo Render time: 50ms
In the example above, the component’s rendering time reduced from 300ms to 50ms by utilizing useMemo effectively. This significant improvement in performance demonstrates the power of memoization.
2. Additional insights:
- Use useMemo judiciously: While useMemo is a powerful tool for optimizing performance, it’s important to use it judiciously. Memoizing every value can lead to unnecessary complexity and overhead. Only memoize computations that are truly expensive and have a noticeable impact on rendering performance.
- Think about dependencies: When using useMemo, pay close attention to the dependencies array. This array determines when the memoized value should be recomputed. Make sure to include all the variables or props that the computation depends on. Omitting a dependency can result in stale data or incorrect behavior.
- Consider alternative solutions: While useMemo is an effective solution for many cases, it’s not always the best choice. In some situations, other hooks like useCallback or useRef may be more suitable. Evaluate your specific use case and choose the most appropriate optimization technique.
- Memoize functions and event handlers: In addition to memoizing values, you can also use useMemo to memoize functions and event handlers. This can be useful when passing functions as props to child components. By memoizing the function, you ensure that the child component doesn’t re-render unnecessarily.
- Profile and measure performance: To identify performance bottlenecks and validate the effectiveness of your optimizations, it’s crucial to profile and measure the performance of your React components. Use tools like React DevTools or browser performance profiling tools to analyze the rendering time and identify areas that can benefit from useMemo.
- Use useMemo with caution in complex calculations: While useMemo can improve performance by memoizing expensive computations, it’s important to consider the trade-off between memoization and memory usage. If the computation requires a significant amount of memory or if the dependency array is large, memoizing the value may consume excessive memory. In such cases, it’s crucial to balance performance gains with memory usage and evaluate whether memoization is truly necessary.
- Use memoization for API calls: Another area where useMemo can be particularly useful is in optimizing API calls. In scenarios where you fetch data from an API endpoint within a component, you can memoize the result of the API call using useMemo. This ensures that the API call is made only when the relevant dependencies change, avoiding unnecessary network requests and improving the responsiveness of your application.
import React, { useMemo } from 'react'; import { fetchData } from 'api'; const MyComponent = ({ userId }) => { const userData = useMemo(async () => { const data = await fetchData(userId); return data; }, [userId]); // Render component using userData return <div>{/* Render UI using userData */}</div>; };
In the example above, the API call to fetchData is memoized using useMemo with [userId] as the dependency. This ensures that the API call is made only when the userId prop changes, preventing unnecessary network requests.
2.1 Combine useMemo with other optimization techniques
While useMemo is powerful on its own, it can be combined with other optimization techniques to further enhance the performance of your React components. For example, you can use memoization in conjunction with React’s memoization of component rendering using the React.memo higher-order component or the React.PureComponent class. This combination can lead to significant performance improvements by minimizing both unnecessary re-renders and expensive computations.
2.2 Test and benchmark your optimizations
As with any performance optimization, it’s essential to thoroughly test and benchmark your code to ensure that the intended performance gains are achieved. Use tools like React Profiler or browser performance testing frameworks to measure the impact of your useMemo optimizations. This will help you identify any regressions or unexpected behavior and fine-tune your code accordingly.
2.3 Consider the granularity of memoization
When using useMemo, it’s important to consider the granularity of memoization. Fine-grained memoization can lead to more precise optimizations, but it can also introduce complexity and maintenance overhead. On the other hand, coarse-grained memoization may not provide as granular optimizations but can simplify the codebase. Find the right balance based on the specific requirements of your application.
2.4 Avoid excessive nesting of useMemo
While it’s possible to nest multiple useMemo calls within a component, be cautious about excessive nesting. Deeply nested useMemo calls can complicate the code and make it harder to understand and maintain. If you find yourself nesting multiple useMemo calls, consider refactoring the logic to separate it into smaller, more manageable components.
Memoize derived values: In addition to memoizing expensive computations, useMemo is also useful for memoizing derived values. Derived values are calculated based on existing data or props within a component. By memoizing these derived values, you can avoid recalculating them on every render, especially if they are computationally intensive.
import React, { useMemo } from 'react'; const MyComponent = ({ data }) => { const derivedValue = useMemo(() => { // Calculate derived value from data return calculateDerivedValue(data); }, [data]); // Render component using derivedValue return <div>{/* Render UI using derivedValue */}</div>; };
In the example above, the derived value is memoized using useMemo with [data] as the dependency. This ensures that the derived value is only recalculated when the data prop changes, preventing unnecessary calculations.
2.5 Consider the alternatives
While useMemo is a powerful optimization technique, it’s important to consider alternative approaches when applicable. For example, if you have a component that needs to fetch data from an API, you might consider using React Query or SWR, which are libraries specifically designed for data fetching and caching. These libraries handle caching and automatic data refetching, eliminating the need for manual memoization.
2.6 Understand the trade-offs
Like any optimization technique, useMemo involves trade-offs. Memoizing values can improve performance, but it also introduces additional complexity to the codebase. It’s important to weigh the benefits against the complexity and maintenance costs. Consider factors such as the frequency of prop changes, the complexity of computations, and the overall performance requirements of your application.
-
Conclusion
React’s useMemo hook is a powerful tool for optimizing component rendering and improving performance in React applications. By memoizing expensive computations, API calls, and derived values, you can reduce unnecessary re-computations and enhance the overall efficiency of your components.
In this blog post, we explored various aspects of useMemo, including its usage, considerations for granularity, and the memoization of derived values. We also emphasized the importance of avoiding excessive nesting and considering alternative approaches when appropriate.
Remember that performance optimization is a continuous process. Regularly profile and measure the performance of your React components to identify areas that can benefit from useMemo or other optimization techniques. Strive for a balance between performance gains and code maintainability.
By mastering the art of useMemo and applying it judiciously, you can optimize your React components, improve rendering efficiency, and deliver blazing-fast user experiences. Happy coding and optimizing!
Table of Contents
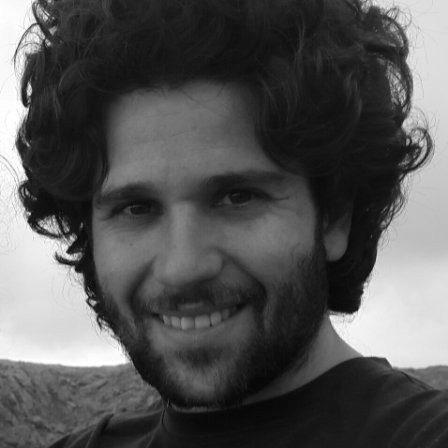
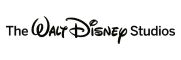