Using ReactJS for Fast Web Development
ReactJS is a popular JavaScript library used for building user interfaces. With the increasing demand for web applications that provide fast and seamless user experiences, the importance of speed in web development has never been more significant.
Table of Contents
In this article, we will explore the myths and realities of ReactJS speed and provide tips for optimizing performance.
1. ReactJS: A Brief Overview
ReactJS was introduced by Facebook in 2011 as an open-source JavaScript library for building user interfaces. It quickly gained popularity among developers due to its simplicity, flexibility, and reusability of code.
ReactJS uses a virtual DOM (Document Object Model) to optimize rendering performance and minimize the number of updates needed to display changes in the UI.
2. Why is Speed Important in Web Development?
Speed is crucial in web development for several reasons. First, it helps to improve user experience. People are more likely to stay on your website or web application if it loads quickly and functions smoothly. Slow websites and web applications, on the other hand, can lead to frustration and a high bounce rate. Second, speed can help to improve your website or web applications’ search engine optimization (SEO).
Search engines like Google prioritize websites that load quickly and function smoothly, which can help to improve your website’s ranking in search engine result pages (SERPs). Finally, speed can help to reduce your development time and costs. By using a fast and efficient library like ReactJS, you can build web applications and websites in less time, which can help to save on development costs.
3. Why is ReactJS Popular for Fast Web Development?
ReactJS is a popular JavaScript library for building user interfaces, known for its performance and speed. The reason behind its speed is its virtual DOM (Document Object Model) implementation, as explained in the article by Jelvix. ReactJS utilizes a virtual representation of the actual DOM, which allows it to update only the necessary parts of the actual DOM when a change occurs, instead of updating the entire DOM tree.
This approach significantly reduces the amount of time and resources required for updating the UI, making ReactJS faster and more efficient than other traditional web development frameworks. Additionally, ReactJS also utilizes a one-way data flow and a component-based structure, which further contribute to its fast performance by minimizing redundant updates and improving code reusability.
4. Best Practices for Improving ReactJS Speed
Optimizing the performance of a ReactJS application is crucial for providing a smooth user experience. Here are several best practices that developers can use to improve the performance of a ReactJS application:
Use the latest version of ReactJS:
Using the latest version of ReactJS ensures that the application takes advantage of the latest performance improvements and bug fixes.
Optimize rendering:
ReactJS uses a virtual DOM to manage updates to the UI. One of the primary causes of slow ReactJS performance is inefficient rendering. Optimize rendering by using the shouldComponentUpdate lifecycle method to control when a component should update, prevent unnecessary rendering, and improve performance.
Avoid excessive state updates:
ReactJS components rely on state to manage changes to the UI. However, excessive state updates can slow down performance. To avoid this, try to minimize the number of state updates and use the setState method only when necessary.
Use lazy loading:
Lazy loading is a technique that loads only the necessary components and resources when needed. This can significantly improve performance by reducing the initial load time and minimizing the number of network requests.
Optimize images and other assets:
Images and other assets can significantly impact the performance of a ReactJS application. To optimize performance, it’s important to compress and resize images and other assets to reduce their file size. You can also use image placeholders or lazy loading to speed up the initial load time.
Minimize the use of third-party libraries:
Third-party libraries can be a useful addition to a ReactJS project, but they can also negatively impact performance. To avoid this, try to minimize the use of third-party libraries and only use them when necessary. When using third-party libraries, make sure to choose lightweight and well-optimized libraries.
Use server-side rendering:
Server-side rendering is a technique that pre-renders web pages on the server before sending them to the client. This can significantly improve performance by reducing the initial load time and improving SEO. ReactJS provides built-in support for server-side rendering, and several third-party libraries are available for this purpose.
Use memoization:
Memoization is a technique that caches the results of expensive function calls and returns the cached result when the same input occurs again. This can improve performance by reducing the number of function calls and re-renders.
Use the React profiler:
The React Profiler is a built-in tool that helps developers identify performance bottlenecks in their ReactJS applications. Use the React Profiler to identify components that take a long time to render and optimize them for better performance.
Optimize the build process:
Optimizing the build process can significantly improve the performance of a ReactJS application. Use tools like Webpack to minimize the size of the application bundle and reduce the load time.
Use code-splitting:
Code splitting is a technique that allows developers to split their code into smaller, more manageable chunks that can be loaded on demand. This can improve performance by reducing the initial load time and improving the overall load time of the application. ReactJS provides built-in support for code splitting through the dynamic import() syntax.
Minimize the number of re-renders:
Re-rendering components can be a time-consuming process and can slow down the performance of a ReactJS application. To minimize the number of re-renders, use the React.memo() Higher Order Component (HOC) to memoize the component and prevent unnecessary re-renders. Additionally, use the PureComponent class instead of the regular Component class when possible, as PureComponent automatically implements shouldComponentUpdate() to prevent unnecessary re-renders.
Here’s an example of using React.memo() to memoize a component:
import React from 'react'; const MemoizedComponent = React.memo((props) => { return ( // render component ); }); export default MemoizedComponent;
In this example, the React.memo() HOC memorizes the component, preventing unnecessary re-renders when the props haven’t changed.
In summary, code splitting and minimizing the number of re-renders are additional best practices that developers can use to optimize the performance of a ReactJS application. By using these techniques along with the other best practices mentioned above, developers can ensure that their ReactJS application provides a smooth and fast user experience.
5. Benefits of ReactJS for Fast Development
ReactJS offers numerous benefits for fast web development, as outlined in various articles and resources. Firstly, ReactJS’s virtual DOM implementation allows it to update only the necessary parts of the actual DOM, resulting in faster and more efficient performance. This approach significantly reduces the amount of time and resources required for updating the UI, allowing developers to build high-quality web applications quickly. Secondly, ReactJS utilizes a component-based structure, enabling developers to break down complex UI elements into smaller, reusable components.
This approach promotes code reusability and simplifies maintenance, as developers can easily update or replace individual components without affecting the entire application. Additionally, ReactJS has a vast community of developers and offers an extensive range of libraries and tools that can further accelerate the web development process.
Finally, ReactJS’s one-way data flow and strict programming rules help avoid common programming errors, leading to more reliable and efficient code. Overall, ReactJS is an ideal choice for developers seeking to build fast, efficient, and scalable web applications.
5.1 The scalability when using ReactJS
ReactJS is a popular front-end JavaScript library that is known for its performance, speed, and scalability. One of the key benefits of using ReactJS is its ability to scale easily. React’s modular architecture and component-based approach make it simple to break down complex user interfaces into smaller, reusable components.
These components can then be combined and reused throughout the application, making it easy to maintain and scale as the application grows. Additionally, React’s virtual DOM allows for efficient updates and rendering of changes, even in large and complex applications, which further contributes to its scalability. Overall, ReactJS provides developers with a robust and flexible framework that can easily accommodate the needs of any size application.
5.2 The larger developer community when using ReactJS
ReactJS has one of the largest and most active developer communities in the world of front-end web development. As an open-source library, ReactJS is constantly evolving and improving, with new features and updates being released regularly. This is largely thanks to the large community of developers who contribute to the development and maintenance of the library. The React community is highly active, with numerous online forums, blogs, and resources available for developers to learn and share knowledge.
This vibrant community also provides a wealth of pre-built components, libraries, and tools that developers can use to accelerate their development process. With so many talented developers working with ReactJS, there is always a wealth of knowledge and expertise available to help troubleshoot issues and find innovative solutions. Overall, the large and engaged ReactJS community is a key strength of the library and one that continues to drive its growth and popularity.
5.3 React offers easy integration with other tools
ReactJS is a highly flexible and versatile library that can be easily integrated with a wide range of other tools and technologies. The installation and setup of React is straightforward, and it can be easily added to a project using a package manager like npm. React’s component-based architecture is a key feature that enables developers to break down complex UI elements into smaller, reusable components. These components can be easily plugged into different parts of the application, making it easy to maintain and scale the application over time.
React’s use of state and props is another key feature that enables developers to manage and update the application’s data and user interface. ‘State’ represents the data that changes over time, while props are used to pass data between components. This approach enables developers to create highly modular and customizable components that can be reused throughout the application.
Overall, ReactJS’s combination of easy installation, component-based architecture, and use of state and props make it an ideal choice for building scalable and flexible web applications that can be easily integrated with a variety of other tools and technologies.
6. What are the challenges and limitations of ReactJS for fast web development?
While ReactJS is a powerful and flexible library that can help developers build complex web applications quickly and efficiently, there are also some challenges and limitations that should be considered when using it for fast web development.
One of the biggest challenges is the learning curve that comes with using ReactJS. While React is a popular library, it requires a strong understanding of JavaScript and modern front-end development practices. Additionally, React’s component-based architecture can be complex for new developers to understand, which can slow down development time.
Another limitation of ReactJS is its lack of built-in tools for state management and routing. While React provides developers with the flexibility to choose their own state management and routing solutions, this can also require additional setup and configuration time.
Finally, React’s virtual DOM can also have limitations when it comes to performance in large and complex applications. While React’s virtual DOM is designed to improve performance and speed up development, it can become less efficient when dealing with large data sets or frequently changing data.
Overall, while ReactJS is a powerful and popular tool for fast web development, it also has some challenges and limitations that developers should be aware of when choosing to use it.
Table of Contents
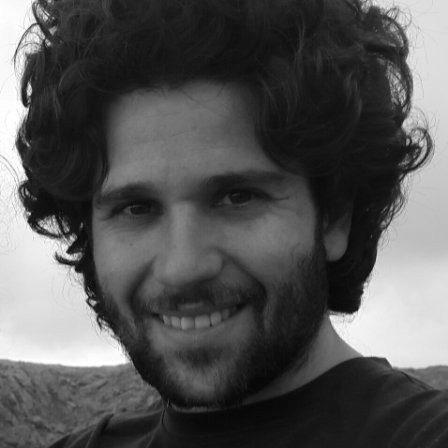
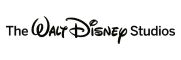