ReactJS Lifecycle Methods for Managing Component State
ReactJS is a popular JavaScript library for building user interfaces. One of the most powerful features of ReactJS is its component lifecycle methods, which allow you to manage component state throughout the lifecycle of your application.
Table of Contents
In this blog post, we’ll provide a complete guide to ReactJS lifecycle methods and how they can be used to manage component state in your ReactJS applications.
1. Introduction to ReactJS Lifecycle Methods
ReactJS components have a lifecycle that can be divided into three phases: Mounting, Updating, and Unmounting. During these phases, a series of methods are called that allows you to manage the state of your components. These methods are called ReactJS lifecycle methods.
The following is a list of the lifecycle methods in ReactJS, grouped by the phase in which they are called:
Mounting:
- constructor()
- static getDerivedStateFromProps()
- render()
- componentDidMount()
Updating:
- static getDerivedStateFromProps()
- shouldComponentUpdate()
- render()
- getSnapshotBeforeUpdate()
- componentDidUpdate()
Unmounting:
- componentWillUnmount()
1.1 Mounting Phase
The Mounting phase is the first phase of a component’s lifecycle. During this phase, the component is created and inserted into the DOM.
1.1.1 constructor()
The constructor() method is called first when a component is created. This method is used to initialize the state of the component and bind event handlers.
1.1.2 static getDerivedStateFromProps()
The static getDerivedStateFromProps() method is called after the constructor() method. It is used to update the state of the component based on changes to the props.
1.1.3 render()
The render() method is called after the getDerivedStateFromProps() method. It returns the HTML markup that should be displayed on the screen.
1.1.3 componentDidMount()
The componentDidMount() method is called after the component is rendered to the DOM. This method is used to perform any necessary setup, such as fetching data from an API or setting up event listeners.
1.2 Updating Phase
The Updating phase is the second phase of a component’s lifecycle. During this phase, the component is updated based on changes to its props or state.
1.2.1 static getDerivedStateFromProps()
The static getDerivedStateFromProps() method is also called during the Updating phase. It is used to update the state of the component based on changes to the props.
1.2.2 shouldComponentUpdate()
The shouldComponentUpdate() method is called before the component is re-rendered. It is used to determine whether the component should be re-rendered or not.
1.2.3 render()
The render() method is called if the shouldComponentUpdate() method returns true. It returns the HTML markup that should be displayed on the screen.
1.2.4 getSnapshotBeforeUpdate()
The getSnapshotBeforeUpdate() method is called after the render() method but before the component is updated. It is used to capture information about the current state of the component, such as the scroll position.
1.2.5 componentDidUpdate()
The componentDidUpdate() method is called after the component is updated. This method is used to perform any necessary cleanup or additional updates, such as updating the DOM or making additional API calls.
1.3 Unmounting Phase
The Unmounting phase is the final phase of a component’s lifecycle. During this phase, the component is removed from the DOM.
1.3.1 componentWillUnmount()
The componentWillUnmount() method is called before the component is removed from the DOM. It is used to perform any necessary cleanup, such as removing event listeners or canceling API calls.
2. Managing Component State with Lifecycle Methods
ReactJS lifecycle methods can be used to manage the state of your components throughout the lifecycle of your application. Here are a few examples of how lifecycle methods can be used to manage component state:
2.1 Using constructor()
You can use the constructor() method to initialize the state of your component. For example:
javascript
class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={() => this.setState({ count: this.state.count + 1 })}> Increment </button> </div> ); } }
In this example, we use the constructor() method to initialize the count state variable to 0.
2.2 Using componentDidMount()
You can use the componentDidMount() method to perform any necessary setup, such as fetching data from an API or setting up event listeners. For example:
javascript
class TodoList extends React.Component { constructor(props) { super(props); this.state = { todos: [] }; } componentDidMount() { fetch('/api/todos') .then(response => response.json()) .then(data => this.setState({ todos: data })); } render() { return ( <ul> {this.state.todos.map(todo => <li key={todo.id}>{todo.text}</li>)} </ul> ); } }
In this example, we use the componentDidMount() method to fetch data from an API and update the todos state variable.
2.3 Using shouldComponentUpdate()
You can use the shouldComponentUpdate() method to determine whether the component should be re-rendered or not. For example:
kotlin
class Checkbox extends React.Component { constructor(props) { super(props); this.state = { isChecked: false }; } shouldComponentUpdate(nextProps, nextState) { return nextState.isChecked !== this.state.isChecked; } handleClick = () => { this.setState({ isChecked: !this.state.isChecked }); }; render() { return ( <label> <input type="checkbox" checked={this.state.isChecked} onChange={this.handleClick} /> {this.props.label} </label> ); } }
In this example, we use the shouldComponentUpdate() method to prevent unnecessary re-renders of the component if the isChecked state variable hasn’t changed.
3. Conclusion
ReactJS lifecycle methods are a powerful tool for managing component state in your ReactJS applications. By understanding how these methods work and how to use them effectively, you can build more robust and scalable applications that provide a seamless user experience.
In this blog post, we provided a complete guide to ReactJS lifecycle methods, including examples of how to use them to manage component state. We hope that this guide has been helpful in understanding how to use ReactJS lifecycle methods effectively in your own applications.
Table of Contents
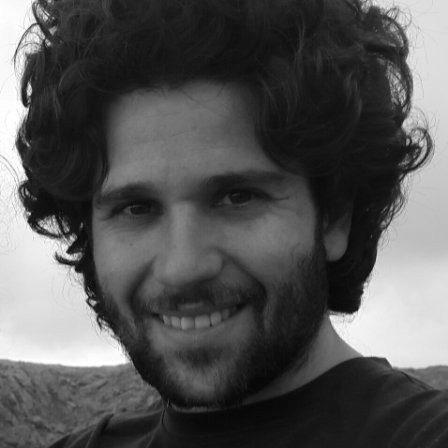
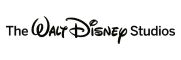