10 Reasons Why React Hooks Are the Future of Web Development
React has been a dominant player in web development since its release in 2013. It’s an open-source JavaScript library that’s maintained by Facebook, and it has a massive following in the web development community.
However, in recent years, alternative frameworks like Next.js and Remix have been gaining popularity. This has led to React’s core team deprecating Create React App (CRA), a popular tool for setting up a new React project. Instead, they’re encouraging developers to use React Hooks, which have become the default way of writing React components.
Table of Contents
In this blog post, we’ll explore the increasing popularity of alternative frameworks, the deprecation of CRA, and the rise of React Hooks.
The Increasing Popularity of Alternative Frameworks like Next.js and Remix
React has always been just a view library, which means that it doesn’t come with built-in support for server-side rendering, routing, or data fetching. However, many web applications require these features, and developers have traditionally relied on third-party libraries and tools to add them to their React projects. This has led to a proliferation of React-based frameworks that provide these features out of the box.
Next.js is one such framework that’s gaining popularity in the React community. It’s a minimalistic framework that provides server-side rendering, automatic code splitting, and static exporting. It also comes with built-in support for popular technologies like Sass, TypeScript, and GraphQL. Next.js has gained a reputation for being easy to use and for providing fast performance out of the box.
Remix is another alternative framework that’s gaining traction. It’s a full-stack framework that provides server-side rendering, routing, and data fetching out-of-the-box. It also comes with a built-in build tool and has a plugin system that allows developers to add additional functionality easily. Remix is gaining a reputation for being easy to use, fast, and flexible.
The popularity of these alternative frameworks has led to many developers questioning the need for React itself. After all, if you can get everything you need out-of-the-box with a framework like Next.js or Remix, why bother with React? However, React still has a lot to offer, especially when it comes to building complex user interfaces.
The Deprecation of Create React App
Create React App (CRA) is a popular tool for setting up a new React project. It provides a pre-configured development environment that includes all the necessary tooling for writing, testing, and building a React application. CRA has been a popular choice for many developers because it saves time and eliminates the need to set up a build system from scratch.
However, the React core team has announced that they’re deprecating CRA in favor of a new tool called npm init react-app. This new tool is based on CRA but provides a more flexible and modular approach to setting up a new React project. It also makes it easier to customize the tooling to meet your specific needs.
The decision to deprecate CRA reflects a broader trend in the React community towards a more flexible and modular approach to building React applications. React itself has always been designed to be highly composable, and this new approach to tooling reflects that philosophy.
How Hooks Are Now the Default
React Hooks were introduced in 2018 as a new way of writing React components. Hooks allow you to use state and other React features in functional components, which means you no longer need to use class components to take advantage of these features.
Hooks have become incredibly popular in the React community and have quickly become the default way of writing React components. In fact, the React core team has announced that they’ll no longer be adding new features to class components and will focus exclusively on improving Hooks.
10 Reasons to Use React Hooks
Hooks provide several benefits over class components, including:
1. Easier to Understand and Maintain
Hooks are easier to understand and maintain than class components. They’re more intuitive, and they don’t require you to remember the intricacies of this keyword or the component lifecycle methods. Hooks make it easier to reason about your code, and they make it easier to reuse code between components.
For example, consider the following class component that uses state:
class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; this.increment = this.increment.bind(this); } increment() { this.setState((prevState) => ({ count: prevState.count + 1 })); } render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={this.increment}>Increment</button> </div> ); } }
Now compare that to the equivalent functional component using the useState Hook:
function Counter() { const [count, setCount] = useState(0); function increment() { setCount((prevCount) => prevCount + 1); } return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> </div> ); }
The functional component using Hooks is much shorter and easier to understand than the class component. The useState Hook is also more intuitive than the this.setState method used in the class component.
2. Better Performance
Hooks can improve the performance of your React application. This is because Hooks are more efficient than class components in several ways. For example, Hooks reduce the amount of boilerplate code you need to write, which can make your code smaller and faster.
Hooks also make it easier to optimize your code using techniques like memoization and useCallback. Memoization is the process of caching the result of a function so that it doesn’t need to be recomputed every time it’s called. useCallback is a Hook that memoizes a function so that it doesn’t need to be recreated every time the component is rendered. These techniques can help reduce unnecessary re-renders and improve the overall performance of your application.
3. More Flexible
Hooks are more flexible than class components because they allow you to use state and other React features in functional components. This means you can use Hooks in conjunction with other features like context, memoization, and asynchronous data fetching.
Hooks also make it easier to compose and reuse code between components. You can create custom Hooks that encapsulate complex logic and reuse them across different components in your application.
4. Encourages Functional Programming
Hooks encourage functional programming principles in your React code. Functional programming is a programming paradigm that emphasizes immutability, purity, and compositionality. Hooks are designed to be composable, which means you can reuse them in different contexts and compose them together to create more complex behavior.
Functional programming can make your code more predictable and easier to reason about. It also makes it easier to test your code, since pure functions are easier to test than impure functions.
5. Future-Proof
Finally, Hooks are future-proof because they’re the default way of writing React components. The React core team has stated that they won’t be adding new features to class components, and they’ll focus exclusively on improving Hooks. This means that if you want to stay up-to-date with the latest features and improvements in React, you need to learn how to use Hooks.
By using Hooks, you’ll also be better positioned to take advantage of future features and improvements in React. Hooks are designed to be composable and flexible, which means they can be used to build more complex and powerful applications.
6. Better Debugging
Hooks make it easier to debug your React code. For example, suppose you have a component that fetches data from an API and displays it. If the component isn’t working correctly, you can isolate the useEffect Hook responsible for fetching the data to see if it’s causing the problem. This can help you quickly identify and fix the issue.
function MyComponent() { const [data, setData] = useState(null); useEffect(() => { fetch("https://api.example.com/data") .then((response) => response.json()) .then((data) => setData(data)) .catch((error) => console.log(error)); }, []); if (!data) { return <div>Loading...</div>; } return <div>{data}</div>; }
7. Better Code Reusability
Hooks make it easier to reuse code between components. For example, suppose you have two components that both need to fetch data from an API. Instead of duplicating the data fetching logic in both components, you can create a custom Hook that encapsulates the data fetching logic and reuse it across both components.
function useDataFetching(url) { const [data, setData] = useState(null); useEffect(() => { fetch(url) .then((response) => response.json()) .then((data) => setData(data)) .catch((error) => console.log(error)); }, [url]); return data; } function ComponentA() { const data = useDataFetching("https://api.example.com/data"); if (!data) { return <div>Loading...</div>; } return <div>{data}</div>; } function ComponentB() { const data = useDataFetching("https://api.example.com/other-data"); if (!data) { return <div>Loading...</div>; } return <div>{data}</div>; }
8. Improved Readability
Hooks can improve the readability of your React code. For example, suppose you have a class component that manages state and performs an effect. The state and effect methods may be scattered throughout the component, making it difficult to understand the overall behavior of the component.
class MyComponent extends React.Component { constructor(props) { super(props); this.state = { data: null }; this.getData = this.getData.bind(this); } componentDidMount() { this.getData(); } async getData() { const response = await fetch("https://api.example.com/data"); const data = await response.json(); this.setState({ data }); } render() { if (!this.state.data) { return <div>Loading...</div>; } return <div>{this.state.data}</div>; } }
Now, compare that to the equivalent functional component using the useState and useEffect Hooks:
function MyComponent() { const [data, setData] = useState(null); useEffect(() => { async function fetchData() { const response = await fetch("https://api.example.com/data"); const data = await response.json(); setData(data); } fetchData(); }, []); if (!data) { return <div>Loading...</div>; } return <div>{data}</div>; }
The Hooks version is shorter and easier to understand, and the state and effect are clearly visible in one place.
9. Improved Type Safety
Hooks can improve the type safety of your React code. For example, suppose you have a component that takes props of a specific type. With class components, you would need to use PropTypes to validate the type of the props. However, with Hooks and TypeScript, you can use interfaces to define the shape of your props and ensure that they are of the correct type.
interface Props { name: string; age: number; } function MyComponent(props: Props) { return ( <div> <p>Name: {props.name}</p> <p>Age: {props.age}</p> </div> ); }
In this example, Props is an interface that defines the shape of the props that MyComponent expects. By using TypeScript, you can ensure that the props are of the correct type and catch errors early.
10. Improved Accessibility
Hooks can improve the accessibility of your React applications. For example, suppose you have a component that needs to be accessible to users with disabilities, including users who rely on screen readers. You can use the useLayoutEffect Hook to ensure that your component is accessible to users who rely on screen readers.
import { useLayoutEffect } from "react"; function MyComponent(props) { const ref = useRef(null); useLayoutEffect(() => { const node = ref.current; if (node) { node.setAttribute("role", "button"); node.setAttribute("tabIndex", "0"); } return () => { if (node) { node.removeAttribute("role"); node.removeAttribute("tabIndex"); } }; }, []); return <div ref={ref}>{props.children}</div>; }
In this example, MyComponent uses the useLayoutEffect Hook to set the role and tabIndex attributes on the component’s div element. These attributes are important for making the component accessible to users who rely on screen readers. The useLayoutEffect Hook ensures that the attributes are set after the component has rendered and are cleaned up when the component is unmounted. This helps ensure that your React applications are accessible to all users.
Conclusion
React Hooks have become the default way of writing React components and for good reason. They’re easier to understand and maintain than class components, they improve performance, they’re more flexible, they encourage functional programming principles, and they’re future-proof.
While alternative frameworks like Next.js and Remix are gaining popularity, React still has a lot to offer when it comes to building complex user interfaces. And with the deprecation of Create React App, the React community is moving towards a more flexible and modular approach to building React applications.
If you’re new to React, or if you’re still using class components, now is the time to start learning and using Hooks. They’ll make your code easier to understand and maintain, and they’ll position you well for the future of React development.
Table of Contents
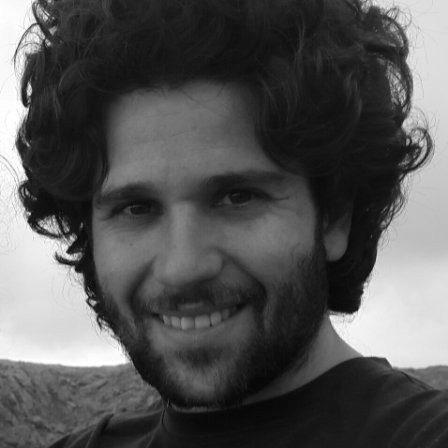
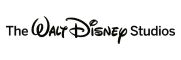