Achieve Smooth and Efficient Animations in ReactJS using requestAnimationFrame
Modern web applications have become increasingly dynamic and interactive, primarily driven by the expertise of ReactJS developers. Users today demand smooth animations, transitions, and immediate response times. One tool that skilled ReactJS developers can leverage to create such an engaging experience is the `requestAnimationFrame()` function. This method, available in the Window API, is key to creating efficient, smooth animations. In the context of ReactJS, it can be employed to optimize user interface updates and animations, thus making it an essential skill for those looking to hire ReactJS developers. Let’s dive into how we can leverage this powerful function.
What is requestAnimationFrame()?
`requestAnimationFrame()` is a method that tells the browser that you wish to perform an animation and requests that the browser calls a specified function to update an animation before the next repaint. This repaint generally happens 60 times per second, which is perceived by the human eye as smooth motion.
When compared to traditional JavaScript timers like `setTimeout` or `setInterval`, `requestAnimationFrame()` has several advantages:
- Rate limiting: Animations are limited to the screen refresh rate (usually 60Hz), which means your animations won’t run at higher than 60 frames per second. This is a sensible default that provides a smooth experience without using unnecessary CPU and GPU resources.
- VSync alignment: The updates align with the device’s screen refresh rate, providing smoother animations.
- Power-efficiency: Browsers can opt to pause animations in separate tabs or windows, or when the user minimizes the browser, contributing to power efficiency.
- Automatic throttling: The browser can intelligently throttle frames when it’s under heavy load.
Using requestAnimationFrame() in ReactJS
Though `requestAnimationFrame()` isn’t a part of ReactJS, it can be used alongside React to create smooth, optimized animations. It fits in naturally with React’s declarative programming model. Let’s see how to implement this with a simple counter component example.
First, let’s create a basic counter component without any animations.
```jsx import React, { useState } from 'react'; const Counter = () => { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; return ( <div> <p>You clicked {count} times</p> <button onClick={increment}> Click me </button> </div> ); }; export default Counter; ```
The above component will update the count each time we click the button. But let’s say we want to animate the counting. We can use `requestAnimationFrame()` to create a smoother transition.
```jsx import React, { useState, useEffect } from 'react'; const Counter = () => { const [count, setCount] = useState(0); useEffect(() => { let animationFrameId; const increment = (currentCount) => { setCount(currentCount); if (currentCount < 100) { animationFrameId = requestAnimationFrame(() => increment(currentCount + 1)); } }; increment(count); return () => { cancelAnimationFrame(animationFrameId); }; }, [count]); return ( <div> <p>You clicked {count} times</p> </div> ); }; export default Counter; ```
In this revised component, the `useEffect` hook kicks off the animation once the component is rendered. The `increment` function updates the state and then schedules the next update using `requestAnimationFrame()`.
To avoid potential memory leaks, we use `cancelAnimationFrame()` in the cleanup function, which gets called when the component is unmounted.
Animating More Complex Scenarios
`requestAnimationFrame()` becomes more vital and apparent when working with complex animations and scenarios, like animating a sequence of images (like in a sprite sheet) or creating complex UI animations. Let’s create a simple sprite animation.
```jsx import React, { useRef, useEffect } from 'react'; const SpriteAnimator = ({ sprites, frameRate }) => { const spriteRef = useRef(null); const frameRef = useRef(0); useEffect(() => { const animate = () => { frameRef.current = (frameRef.current + 1) % sprites.length; spriteRef.current.style.backgroundImage = `url(${sprites[frameRef.current]})`; requestAnimationFrame(animate); }; animate(); return () => { cancelAnimationFrame(animate); }; }, [sprites]); return <div ref={spriteRef} />; }; export default SpriteAnimator; ```
In this example, the `SpriteAnimator` component receives a `sprites` prop that contains an array of image URLs and a `frameRate` prop that specifies how quickly to move through the frames. The `animate` function cycles through the sprites, updating the background image on each frame using `requestAnimationFrame()`.
Performance Considerations and Tips
While `requestAnimationFrame()` can greatly improve the performance of animations, there are certain best practices you should follow to ensure maximum efficiency:
- Avoid heavy computations in each frame: Since the function is called before every frame, any heavy computation will result in skipping frames and a choppy animation. If you have to do heavy computations, try to break them into smaller tasks spread over several frames.
- Cancel your animations: Always remember to cancel your animations in the cleanup function. This not only prevents potential memory leaks but also makes sure unnecessary computations are not being done when the component is not visible.
- Limit DOM updates: Minimizing direct DOM manipulation will greatly boost your performance. Avoid reading or writing to the DOM within the animation loop.
Conclusion
The `requestAnimationFrame()` function is a powerful tool for creating smooth and efficient animations in your ReactJS applications. By understanding and properly using this method, you can greatly enhance the interactivity and responsiveness of your applications, providing a richer user experience.
This technique is often employed by skilled ReactJS developers to ensure optimal performance of their applications. Remember to keep your functions lean, cancel animations when they’re no longer needed, and try to minimize direct DOM manipulation. These are the practices adopted by proficient ReactJS developers to ensure the creation of engaging, animated web applications. If you’re looking to hire ReactJS developers, make sure they have a solid understanding of these concepts for the best results.
Table of Contents
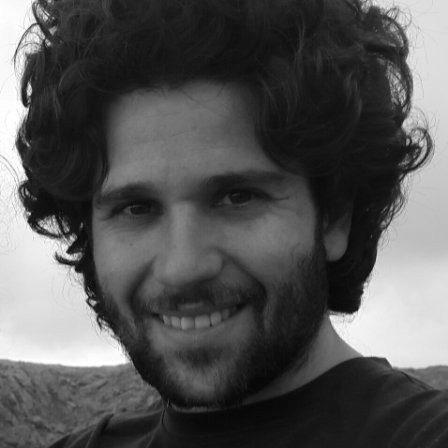
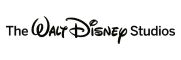