Building Reusable Logic and State Management with ReactJS Custom Hooks
ReactJS has revolutionized front-end development with its component-based architecture and powerful features. One of the key features that makes ReactJS highly flexible and reusable is custom hooks. Custom hooks allow developers to extract reusable logic from components and share it across different parts of an application. In this blog post, we will explore the power of ReactJS custom hooks, their benefits, and how they can be used for building reusable logic and state management. We will also provide code samples and additional insights to illustrate the concepts discussed.
Understanding Custom Hooks
-
Custom hooks encapsulate reusable stateful logic
Custom hooks are JavaScript functions that follow a specific naming convention, starting with the word “use”. These hooks encapsulate reusable logic and provide an abstraction layer that can be easily consumed by React components. Custom hooks can manage state, handle side effects, subscribe to data sources, and perform various other tasks. By creating custom hooks, we can extract complex logic from components and keep them lean, focused, and reusable.
-
Custom hooks promote separation of concerns and code sharing
One of the main advantages of custom hooks is that they promote separation of concerns and code sharing among components. By abstracting reusable logic into custom hooks, we can avoid code duplication and keep our components focused on their primary responsibilities. Custom hooks can be shared across multiple components, enabling code reuse and improving the overall maintainability of the application.
Now that we understand the basics of custom hooks, let’s dive into an example of creating a custom hook for state management.
-
Creating a Custom Hook for State Management
Let’s say we have a component that needs to toggle a boolean value. Instead of managing the state within the component, we can extract this logic into a custom hook.
import { useState } from 'react'; const useToggle = (initialState = false) => { const [state, setState] = useState(initialState); const toggle = () => { setState(!state); }; return [state, toggle]; }; export default useToggle;
In the code above, we define a custom hook called useToggle. It takes an optional initialState parameter with a default value of false. The hook uses the useState hook from React to create a state variable and a setState function for updating the state. The toggle function simply toggles the state value using the setState function.
The custom hook returns an array with two elements: the state value and the toggle function. Now, any component can use this custom hook to manage the toggle state without duplicating the logic.
-
Using the Custom Hook
Let’s see how we can use the useToggle custom hook in a component.
import React from 'react'; import useToggle from './useToggle'; const ToggleComponent = () => { const [isToggled, toggle] = useToggle(false); return ( <div> <button onClick={toggle}>Toggle</button> {isToggled && <p>Toggle is ON</p>} </div> ); }; export default ToggleComponent;
In the code snippet above, we import the useToggle custom hook and use it within the ToggleComponent. We destructure the isToggled state value and the toggle function from the useToggle hook’s return array. The isToggled state value is used to conditionally render the message “Toggle is ON” when the button is clicked.
By using the useToggle custom hook, we keep our component clean and focused on rendering the UI, while the state management logic resides within the custom hook.
-
Additional Insights
5.1. Reusability: Encapsulating logic for reuse across components
One of the significant advantages of custom hooks is their reusability. By encapsulating reusable logic within a custom hook, you can easily share that logic across multiple components. This promotes code reuse and helps in keeping your codebase clean and DRY (Don’t Repeat Yourself).
Custom hooks can encapsulate complex logic, such as form validation, API data fetching, authentication handling, and more. By creating custom hooks for these common tasks, you can centralize the logic and reuse it in different parts of your application. This not only saves development time but also ensures consistent and reliable functionality across your components.
For example, let’s create a custom hook called useFormValidation to handle form validation logic:
import { useState } from 'react'; const useFormValidation = () => { const [values, setValues] = useState({}); const [errors, setErrors] = useState({}); const handleChange = (event) => { setValues({ ...values, [event.target.name]: event.target.value }); }; const handleSubmit = (event) => { event.preventDefault(); // Perform validation logic // Set errors if validation fails }; return { values, errors, handleChange, handleSubmit }; }; export default useFormValidation;
In this example, the useFormValidation custom hook manages the form values and errors state. It provides functions to handle form field changes (handleChange) and form submission (handleSubmit). Other components can import and use this custom hook to handle their form logic without duplicating code.
5.2 Composition: Combining multiple custom hooks for complex behavior
Custom hooks can also be composed together to create more complex behavior. This allows you to leverage the power of multiple custom hooks and build abstractions that encapsulate rich functionality.
For instance, let’s say you have a custom hook called useDataFetching for fetching data from an API:
import { useState, useEffect } from 'react'; const useDataFetching = (url) => { const [data, setData] = useState(null); const [isLoading, setIsLoading] = useState(false); const [error, setError] = useState(null); useEffect(() => { const fetchData = async () => { setIsLoading(true); try { const response = await fetch(url); const result = await response.json(); setData(result); } catch (error) { setError(error); } setIsLoading(false); }; fetchData(); }, [url]); return { data, isLoading, error }; }; export default useDataFetching;
Now, let’s say you want to create a component that fetches data from an API and toggles a loading state. You can compose the useDataFetching and useToggle custom hooks to achieve this:
import React from 'react'; import useDataFetching from './useDataFetching'; import useToggle from './useToggle'; const DataFetchingComponent = () => { const { data, isLoading, error } = useDataFetching('https://api.example.com/data'); const [isDataVisible, toggleDataVisibility] = useToggle(false); return ( <div> <button onClick={toggleDataVisibility}>Toggle Data</button> {isLoading ? ( <p>Loading...</p> ) : ( <> {error ? ( <p>Error: {error.message}</p> ) : ( <> {isDataVisible && data && <p>Data: {data}</p>} </> )} </> )} </div> ); }; export default DataFetchingComponent;
In this example, the DataFetchingComponent uses the useDataFetching custom hook to fetch data from the specified API URL. It also uses the useToggle custom hook to toggle the visibility of the fetched data. By composing these hooks together, you can achieve a component that handles data fetching, loading state, and data visibility with reusable and composable logic.
The power of composition allows you to create custom hooks that encapsulate specific behavior and combine them to build more complex and feature-rich components.
5.3 Testing: Easier testing due to decoupled hooks
Custom hooks provide a great advantage when it comes to testing. Since hooks are decoupled from the components, they can be tested independently, making it easier to write unit tests for your custom hook logic.
When testing a custom hook, you can focus on the logic and functionality it provides without the need to render an entire component. This level of isolation simplifies the testing process and allows for more targeted and efficient test coverage.
For example, let’s say we want to test the useToggle custom hook:
import { renderHook, act } from '@testing-library/react-hooks'; import useToggle from './useToggle'; test('should toggle the state', () => { const { result } = renderHook(() => useToggle()); expect(result.current[0]).toBe(false); act(() => { result.current[1](); }); expect(result.current[0]).toBe(true); act(() => { result.current[1](); }); expect(result.current[0]).toBe(false); });
In this test, we use the renderHook function from @testing-library/react-hooks to render the useToggle custom hook. We can then access the current state value and toggle function from the result.current object. By simulating user interactions using the act function, we can test the state toggling behavior of the custom hook.
Testing custom hooks in isolation allows you to validate their functionality and behavior without the need for complex component rendering and setup. It simplifies the testing process and provides more confidence in the reliability of your hooks.
5.4. Naming Conventions: Best practices for naming custom hooks
When creating custom hooks, it is recommended to follow certain naming conventions to ensure clarity and adherence to best practices. Custom hooks should always start with the word “use” to indicate that they are React hooks. Following this convention makes it easier for other developers to understand that a function is a custom hook and should be used accordingly.
Additionally, you can leverage the ESLint eslint-plugin-react-hooks plugin, which provides additional linting rules specifically for hooks. Enforcing these rules in your codebase can help catch potential issues and ensure consistent usage of hooks throughout your application.
-
Managing Local Storage with a Custom Hook
Another common use case for custom hooks is managing data in the browser’s local storage. Local storage provides a convenient way to store data persistently on the client-side. Let’s create a custom hook called useLocalStorage that handles the storing and retrieving of data from local storage.
import { useState, useEffect } from 'react'; const useLocalStorage = (key, initialValue) => { const [value, setValue] = useState(() => { const storedValue = localStorage.getItem(key); return storedValue ? JSON.parse(storedValue) : initialValue; }); useEffect(() => { localStorage.setItem(key, JSON.stringify(value)); }, [key, value]); return [value, setValue]; }; export default useLocalStorage;
In the code above, the useLocalStorage custom hook takes two parameters: key and initialValue. It uses the useState hook to manage the value state. On the initial render, it checks if a value is stored in local storage using the provided key. If a value exists, it parses and sets it as the initial state. Otherwise, it uses the provided initialValue. The hook also utilizes the useEffect hook to update the stored value whenever the value state changes.
To use the useLocalStorage custom hook in a component, you can follow this example:
import React from 'react'; import useLocalStorage from './useLocalStorage'; const LocalStorageComponent = () => { const [count, setCount] = useLocalStorage('count', 0); const increment = () => { setCount(count + 1); }; return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> </div> ); }; export default LocalStorageComponent;
In this example, the LocalStorageComponent uses the useLocalStorage custom hook to manage the count state in local storage. The count state is initialized with the value retrieved from local storage using the key ‘count’, or with an initial value of 0 if no value is found. The setCount function updates the count state and automatically updates the stored value in local storage.
By using custom hooks like useLocalStorage, you can easily manage persistent data in local storage without having to write repetitive code in each component. This promotes code reusability and keeps your components focused on their primary responsibilities.
-
Using Custom Hooks for API Data Fetching
Another powerful use case for custom hooks is abstracting the logic for API data fetching. Custom hooks can handle the data fetching process, loading state management, and error handling, making it easier to fetch and manage data from APIs in your components. Let’s create a custom hook called useApiData for handling API data fetching.
import { useState, useEffect } from 'react'; const useApiData = (url) => { const [data, setData] = useState(null); const [isLoading, setIsLoading] = useState(false); const [error, setError] = useState(null); useEffect(() => { const fetchData = async () => { setIsLoading(true); try { const response = await fetch(url); const result = await response.json(); setData(result); } catch (error) { setError(error); } setIsLoading(false); }; fetchData(); }, [url]); return { data, isLoading, error }; }; export default useApiData;
In the code snippet above, the useApiData custom hook takes a URL as a parameter. It uses the useState and useEffect hooks to manage the data, isLoading, and error states. The useEffect hook fetches data from the provided URL and updates the state accordingly. The hook returns an object containing the fetched data, loading state, and error object.
To utilize the useApiData custom hook in a component, you can follow this example:
import React from 'react'; import useApiData from './useApiData'; const APIComponent = () => { const { data, isLoading, error } = useApiData('https://api.example.com/data'); if (isLoading) { return <p>Loading...</p>; } if (error) { return <p>Error: {error.message}</p>; } return ( <div> {data && <p>Data: {data}</p>} </div> ); }; export default APIComponent;
In this example, the APIComponent uses the useApiData custom hook to fetch data from the specified API URL. The hook manages the loading state (isLoading), fetched data (data), and error state (error). The component conditionally renders different content based on the loading and error states.
If the data is still being fetched (isLoading is true), the component displays a “Loading…” message. If an error occurs during the API call, the component shows an error message with the error details. Finally, if the data is successfully fetched, the component renders the data.
By utilizing custom hooks for API data fetching, you can abstract the common logic of fetching and handling API data. This promotes code reusability and makes it easier to manage API integration across multiple components in your application.
-
Sharing Custom Hooks
One of the significant advantages of custom hooks is the ability to share them across different components and projects. By extracting reusable logic into custom hooks, you can create a library of hooks that can be easily imported and utilized in various parts of your application.
For example, let’s say you have a custom hook called useFormValidation that handles form validation logic. You can package this custom hook into a separate module and share it with other developers or use it in different projects.
Here’s an example of how the useFormValidation custom hook can be implemented:
import { useState } from 'react'; const useFormValidation = () => { const [values, setValues] = useState({}); const [errors, setErrors] = useState({}); const handleChange = (event) => { setValues({ ...values, [event.target.name]: event.target.value }); }; const handleSubmit = (event) => { event.preventDefault(); // Perform validation logic // Set errors if validation fails }; return { values, errors, handleChange, handleSubmit }; }; export default useFormValidation;
In this example, the useFormValidation custom hook manages form values, errors, and provides functions to handle form field changes and form submission. Other components can import and utilize this custom hook to handle their form logic without duplicating code.
import React from 'react'; import useFormValidation from './useFormValidation'; const FormComponent = () => { const { values, errors, handleChange, handleSubmit } = useFormValidation(); return ( <form onSubmit={handleSubmit}> <input type="text" name="name" value={values.name || ''} onChange={handleChange} /> {errors.name && <p>{errors.name}</p>} <button type="submit">Submit</button> </form> ); }; export default FormComponent;
In this example, the FormComponent utilizes the useFormValidation custom hook to handle form logic. It destructures the required values and functions from the custom hook and uses them within the component to manage form values, errors, and field changes.
By sharing custom hooks, you can create a library of reusable logic that can be easily consumed and integrated into different parts of your application. This promotes code modularity, maintainability, and collaboration among developers.
-
Conclusion
ReactJS custom hooks provide a powerful mechanism for building reusable logic and state management in your applications. By encapsulating complex functionality into custom hooks, you can promote code reuse, separation of concerns, and maintainable code. Custom hooks can be shared across components and projects, making them valuable assets for improving development efficiency.
In this blog post, we explored the concept of ReactJS custom hooks and their benefits. We discussed how custom hooks encapsulate reusable stateful logic and promote separation of concerns and code sharing. We also provided code samples for creating custom hooks related to state management, local storage, API data fetching, and form validation.
We also touched upon additional insights such as the reusability and composition of custom hooks, their testing advantages, naming conventions, and the ability to share custom hooks with other developers.
By leveraging custom hooks, you can create modular and maintainable code, improve development productivity, and enhance the overall quality of your ReactJS applications. Custom hooks offer a way to abstract common logic, reduce code duplication, and make your components more focused and reusable.
So, take advantage of ReactJS custom hooks in your projects, explore different use cases, and build a library of reusable hooks that can boost your development workflow. With the power of custom hooks, you can create more efficient, scalable, and delightful ReactJS applications.
Happy coding!
Table of Contents
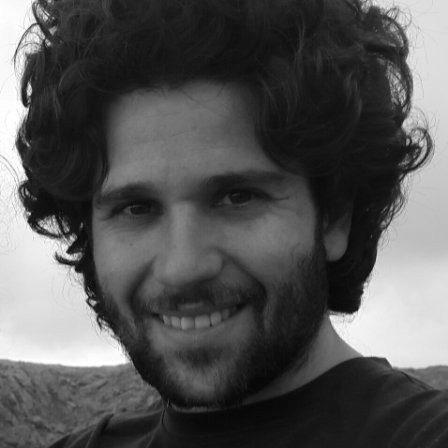
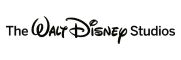