How to implement server-side rendering?
Server-side rendering involves rendering your React components on the server into an HTML string, which is then sent to the browser. This allows the browser to display a fully-rendered page immediately, even before any JavaScript has been parsed and executed. Once the JavaScript loads and executes, React “hydrates” this pre-rendered content, attaching event listeners and bringing the application to life, making it fully interactive.
To implement SSR with React, you’ll need to utilize the `ReactDOMServer` module, which offers methods to render React components as static HTML strings. The primary method you’ll use is `ReactDOMServer.renderToString()`, which, as the name suggests, renders a React element to its initial HTML.
Here’s a basic outline of the process:
- Server Setup: Utilize a Node.js server framework, such as Express.js.
- Routing: Handle your routes on the server, often using a library like `react-router-dom`. For each route, render the corresponding React component.
- Render & Send: Use `ReactDOMServer.renderToString()` to render your React app to a string. Embed this output inside an HTML structure and send it as the response to the client.
- Hydrate on Client: On the client side, instead of using `ReactDOM.render()`, you’d use `ReactDOM.hydrate()`. This method is used to hydrate a container whose HTML contents were rendered by `ReactDOMServer`.
For data-fetching, you might need to ensure that data-dependent components have their data before rendering them on the server. Libraries like Redux can help manage this with tools like `redux-thunk` for asynchronous actions.
There are also frameworks and tools like Next.js, which provide out-of-the-box support for SSR with React, abstracting away much of the manual setup and providing optimizations.
Server-side rendering with React offers enhanced initial load performance and improved SEO. While implementing SSR can introduce additional complexity into your application, the benefits in terms of user experience and discoverability often make it a worthwhile endeavor.
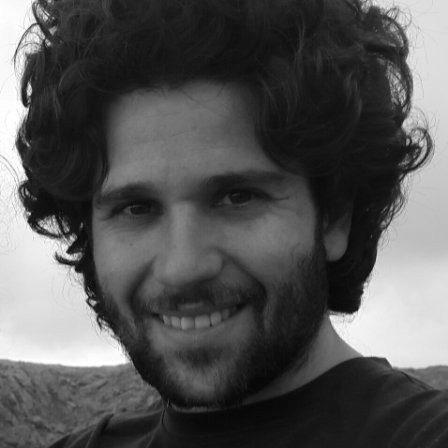
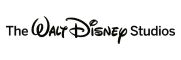