Building Single Page Applications with ReactJS using React Router
React Router is a powerful library that allows developers to create single-page applications with ReactJS. Single-page applications (SPAs) are web applications that load a single HTML page and dynamically update their content as the user interacts with the application.
Table of Contents
React Router provides a way to map URLs to different components in your application, making it easy to create a navigable SPA.
1. Getting Started with React Router
To get started with React Router, you first need to install it using npm or yarn:
npm install react-router-dom
or
csharp
yarn add react-router-dom
Once you have installed React Router, you can import the necessary components from the react-router-dom package:
javascript
import { BrowserRouter, Route, Switch, Link, Redirect } from 'react-router-dom';
2. Defining Routes with React Router
To define routes in your application, you need to use the Route component. The Route component takes two props: path and component. The path prop defines the URL path that the component should be rendered for, and the component prop is the component that should be rendered.
Here’s an example of how to define routes using the Route component:
php
<BrowserRouter> <Switch> <Route path="/" exact component={Home} /> <Route path="/about" exact component={About} /> </Switch> </BrowserRouter>
In this example, we define two routes: one for the root path (/) and another for the /about path. The exact prop is used to ensure that the route only matches the exact path and not any sub-paths.
3. Navigating Between Pages
Once you have defined your routes, you can create links to navigate between pages using the Link component provided by React Router. The Link component works just like an <a> tag, but instead of navigating to a new page, it updates the URL and renders the appropriate component.
Here’s an example of how to use the Link component to create a navigation menu:
php
<nav> <ul> <li> <Link to="/">Home</Link> </li> <li> <Link to="/about">About</Link> </li> </ul> </nav>
In this example, we use the Link component to create two links: one that navigates to the root path (/) and another that navigates to the /about path. When the user clicks on one of these links, the URL is updated and the appropriate component is rendered.
4. Handling URL Parameters
Sometimes you may need to pass parameters to a component via the URL. For example, you may want to display a specific post based on its ID. React Router makes it easy to handle URL parameters using the Route component’s path prop.
Here’s an example of how to define a route with a URL parameter:
php
<Route path="/post/:id" component={Post} />
In this example, we define a route that matches the pattern /post/:id. The : before id indicates that id is a URL parameter. When this route is matched, React Router will pass the id parameter to the Post component as a prop.
Here’s an example of how to use the URL parameter in the Post component:
csharp
function Post(props) { const { id } = props.match.params; // fetch post data using id return ( <div> <h1>Post {id}</h1> <p>This is the content of post {id}.</p> </div> ); } ```
In this example, we use destructuring to extract the id parameter from the match.params object. We can then use this parameter to fetch the post data or perform any other actions needed in the component
.
5. Redirecting with React Router
Finally, React Router also provides a way to redirect the user to a different URL using the Redirect component. The Redirect component takes a to prop that specifies the URL to redirect to.
Here’s an example of how to use the Redirect component to redirect the user to the /about path:
php
<BrowserRouter> <Switch> <Route path="/" exact> <Redirect to="/about" /> </Route> <Route path="/about" exact component={About} /> </Switch> </BrowserRouter>
In this example, we define a route for the root path (/) that uses the Redirect component to redirect the user to the /about path. The exact prop is used to ensure that the Redirect component is only rendered for the root path and not any sub-paths.
6. Additional Features
React Router provides several additional features that can enhance your SPA, such as nested routes, code splitting, and authentication. Here are some examples of how to use these features:
6.1 Nested Routes:
php
<Route path="/posts" component={Posts}> <Route path="/posts/:id" component={Post} /> </Route>
In this example, we define a nested route for the /posts path. When this route is matched, React Router will render the Posts component, which can then render the Post component for a specific post using the id parameter.
6.2 Code Splitting:
javascript
import { lazy, Suspense } from 'react'; const Home = lazy(() => import('./Home')); function App() { return ( <BrowserRouter> <Suspense fallback={<div>Loading...</div>}> <Switch> <Route path="/" exact component={Home} /> <Route path="/about" exact component={About} /> </Switch> </Suspense> </BrowserRouter> ); }
In this example, we use the lazy and Suspense components provided by React to dynamically load the Home component only when it is needed. This can improve the performance of your SPA by reducing the initial load time.
6.3 Authentication:
javascript
import { Redirect, Route } from 'react-router-dom'; function PrivateRoute({ component: Component, ...rest }) { const isAuthenticated = // check if user is authenticated return ( <Route {...rest} render={(props) => ( isAuthenticated ? <Component {...props} /> : <Redirect to="/login" /> )} /> ); }
In this example, we define a PrivateRoute component that checks if the user is authenticated before rendering the component. If the user is not authenticated, the component is redirected to the /login path.
7. Conclusion
React Router is a powerful library that allows developers to create single-page applications with ReactJS. It provides a way to map URLs to different components in your application, making it easy to create a navigable SPA. In this post, we covered the basics of React Router, including routing components, navigating between pages, handling URL parameters, and redirecting. We also covered some additional features, such as nested routes, code splitting, and authentication, that can enhance your SPA. With these tools in hand, you can create powerful and dynamic single-page applications that provide a seamless user experience. However, this is just the tip of the iceberg, and there is much more to explore in React Router.
If you’re interested in learning more about React Router, be sure to check out the official documentation, which provides detailed information on all of the available components and features. Additionally, there are many tutorials and resources available online that can help you get started with React Router and build your own SPAs.
In conclusion, React Router is an essential tool for building single-page applications with ReactJS. With its powerful routing capabilities, React Router makes it easy to create a navigable SPA that provides a seamless user experience. Whether you’re building a simple website or a complex web application, React Router is a must-have tool in your development arsenal. So if you haven’t already, be sure to give it a try and see how it can help you build better SPAs with ReactJS.
Table of Contents
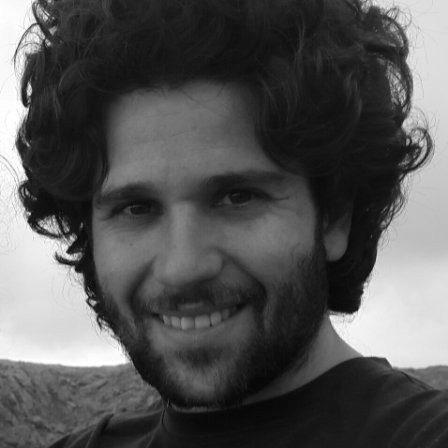
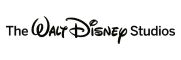