A Comprehensive Guide to State Management Using ReactJS and Redux
ReactJS is a popular JavaScript library for building user interfaces, and Redux is a powerful state management library that works seamlessly with ReactJS. In this blog post, we’ll explore how to use Redux with ReactJS for state management in your applications.
1. Introduction to Redux
Redux is a predictable state container for JavaScript apps. It helps you write applications that behave consistently, run in different environments (client, server, and native), and are easy to test. Redux is commonly used with ReactJS, but it can be used with any other JavaScript library or framework.
Three Main Concepts of Redux:
- Store: The store is a JavaScript object that holds the state of your application.
- Actions: Actions are plain JavaScript objects that describe what happened in your application.
- Reducers: Reducers are functions that take the current state of your application and an action, and return a new state.
Simple example of how Redux works:
arduino
// Define an action const increment = { type: 'INCREMENT' }; // Define a reducer function counter(state = 0, action) { switch (action.type) { case 'INCREMENT': return state + 1; default: return state; } } // Create a store const store = Redux.createStore(counter); // Dispatch an action store.dispatch(increment); // Get the current state console.log(store.getState()); // Output: 1
In this example, we define an increment action that has a type property of “INCREMENT”. We then define a counter reducer that takes the current state and the increment action and returns a new state with the count incremented by one. We create a store with the counter reducer and dispatch the increment action to update the state. Finally, we log the current state of the store to the console.
2. Using Redux with ReactJS
To use Redux with ReactJS, you need to install the react-redux library, which provides bindings between ReactJS and Redux. You can then create a store and wrap your ReactJS components with a Provider component to make the store available to all child components.
Example of how to use Redux with ReactJS:
javascript
// Define an action const increment = { type: 'INCREMENT' }; // Define a reducer function counter(state = 0, action) { switch (action.type) { case 'INCREMENT': return state + 1; default: return state; } } // Create a store const store = Redux.createStore(counter); // Define a component function Counter() { const count = ReactRedux.useSelector(state => state); const dispatch = ReactRedux.useDispatch(); const handleIncrement = () => { dispatch(increment); }; return ( <div> <p>Count: {count}</p> <button onClick={handleIncrement}>Increment</button> </div> ); } // Wrap the component with a Provider ReactDOM.render( <ReactRedux.Provider store={store}> <Counter /> </ReactRedux.Provider>, document.getElementById('root') );
In this example, we define an increment action and a counter reducer, create a store with the counter reducer, and wrap a Counter component with a Provider component to make the store available to the component. The Counter component uses the useSelector hook to get the current state of the store, and the useDispatch hook to dispatch the increment action when the button is clicked
3. State Management with Redux
Redux provides a centralized store for managing the state of your application. This makes it easy to share data between components and keep your application’s state consistent. Here are the main steps to manage state with Redux:
- Define your state: You define your application state in the Redux store.
- Define your actions: You define the actions that can be performed on your state. These actions are plain JavaScript objects with a type property.
- Define your reducers: You define how your state should change in response to your actions. A reducer is a pure function that takes the current state and an action, and returns a new state.
- Dispatch your actions: You dispatch your actions to the store to update the state.
Example of how to manage state with Redux:
go // Define the initial state const initialState = { count: 0 }; // Define the actions const increment = { type: 'INCREMENT' }; const decrement = { type: 'DECREMENT' }; // Define the reducer function counter(state = initialState, action) { switch (action.type) { case 'INCREMENT': return { ...state, count: state.count + 1 }; case 'DECREMENT': return { ...state, count: state.count - 1 }; default: return state; } } // Create a store const store = Redux.createStore(counter); // Dispatch the actions store.dispatch(increment); store.dispatch(decrement); // Get the current state console.log(store.getState()); // Output: { count: 0 }
In this example, we define the initial state of the application as an object with a count property of 0. We then define two actions, increment and decrement, and a reducer that takes the current state and the actions and returns a new state with the count incremented or decremented. We create a store with the counter reducer and dispatch the increment and decrement actions to update the state. Finally, we log the current state of the store to the console.
4. Conclusion
ReactJS and Redux are a powerful combination for building complex applications with predictable state management. By using Redux with ReactJS, you can centralize your application’s state, simplify the management of your application’s data flow, and improve the performance of your components.
In this blog post, we explored how to use Redux with ReactJS for state management in your applications. We covered the basics of Redux, how to use Redux with ReactJS, and how to manage state with Redux. We hope that this guide has been helpful in understanding how to use Redux effectively in your own applications.
Table of Contents
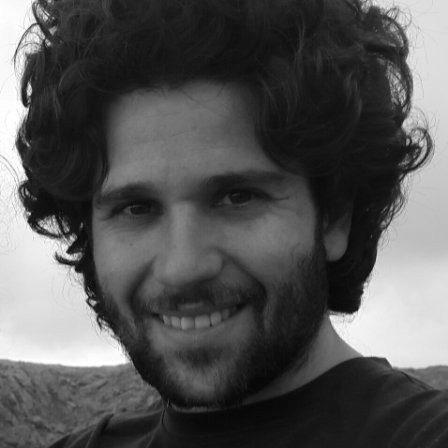
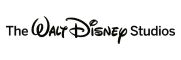