Testing ReactJS Applications with Jest and React Testing Library
ReactJS is a popular JavaScript library for building user interfaces, and testing is a crucial part of building high-quality ReactJS applications. Jest and React Testing Library are two of the most popular tools for testing ReactJS applications.
Table of Contents
In this blog post, we’ll explore how to use Jest and React Testing Library to test your ReactJS components and provide best practices for testing ReactJS applications.
1. What is Jest?
Jest is a JavaScript testing framework that is commonly used for testing ReactJS applications. Jest provides several features that make it easy to write and run tests, including:
- A simple and intuitive API for writing tests
- Built-in mocking and assertion capabilities
- Support for code coverage reporting
- Support for running tests in parallel
Jest is designed to be easy to use and provides a familiar interface for developers who are used to working with testing frameworks like Mocha or Jasmine.
2. What is React Testing Library?
React Testing Library is a library that provides utilities for testing ReactJS components. React Testing Library is built on top of DOM Testing Library, which provides a set of tools for testing DOM nodes and events.
React Testing Library provides several features that make it easy to test ReactJS components, including:
- A simple and intuitive API for rendering components
- Built-in support for querying and interacting with rendered components
- Utilities for testing user interactions and component state changes
React Testing Library is designed to be user-friendly and is built with the philosophy that your tests should resemble the way your users interact with your application.
3. Writing Tests with Jest and React Testing Library
Now that we’ve covered the basics of Jest and React Testing Library, let’s look at how to use them to write tests for your ReactJS applications.
Here’s an example of a simple ReactJS component:
javascript
import React from 'react'; function Button(props) { return <button onClick={props.onClick}>{props.label}</button>; } export default Button;
To test this component with Jest and React Testing Library, we can create a test file with the following code:
javascript
import React from 'react'; import { render, fireEvent } from '@testing-library/react'; import Button from './Button'; test('Button component calls onClick handler when clicked', () => { const handleClick = jest.fn(); const { getByText } = render(<Button label="Click me!" onClick={handleClick} />); const button = getByText('Click me!'); fireEvent.click(button); expect(handleClick).toHaveBeenCalledTimes(1); });
This test file imports the necessary functions from React Testing Library and our Button component. The test then creates a mock function using Jest’s jest.fn() method and renders the Button component with some sample props.
The test then uses React Testing Library’s getByText method to get a reference to the button element and fires a click event using fireEvent.click(). Finally, the test checks to make sure that the mock function was called exactly once using Jest’s toHaveBeenCalledTimes() method.
4. Best Practices for Testing ReactJS Applications
In addition to using Jest and React Testing Library, there are several best practices that you should follow when testing ReactJS applications. Here are a few tips to keep in mind:
- Test behavior, not implementation: When writing tests, focus on testing the behavior of your components, rather than their implementation details. This will make your tests more resilient to changes in your codebase.
- Keep your tests small and focused: Break your tests down into small, focused units that test specific behaviors or components. This will make it easier to diagnose and fix problems when they arise.
- Write tests for edge cases: Make sure to test edge cases and error conditions, as these are often where bugs and vulnerabilities can lurk.
- Use test-driven development: Consider using a test-driven development approach, where you write tests before writing the code to ensure that your code meets the expected behavior.
- Use descriptive test names: Use descriptive and meaningful test names to make it clear what each test is testing.
- Use mocking and stubbing sparingly: Use mocking and stubbing only when necessary, as they can make tests more brittle and difficult to maintain.
5. Conclusion
Testing is an essential part of building high-quality ReactJS applications. Jest and React Testing Library are powerful tools that can help you write effective tests for your applications. By following best practices and writing well-designed tests, you can ensure that your ReactJS applications are reliable, maintainable, and bug-free.
In this blog post, we’ve covered the basics of Jest and React Testing Library, provided an example of how to use them to test a simple ReactJS component, and provided some best practices for testing ReactJS applications.
By incorporating testing into your ReactJS development workflow, you can catch bugs and vulnerabilities before they make it into production, and build applications that are more reliable and maintainable in the long run.
Table of Contents
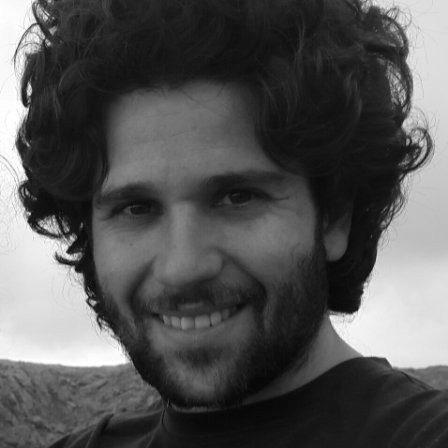
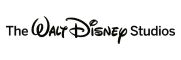