10 Tools for Building React Component Libraries in 2023
React is a popular JavaScript library for building user interfaces. With its component-based architecture, React has become a go-to choice for front-end developers. The library’s popularity has led to the creation of many React component libraries that make it easier to build complex user interfaces.
Table of Contents
If you’re a front-end developer looking to create your own React component library, you’re in luck. There are many tools available that can help you build high-quality and scalable React components. In this blog post, we’ll discuss five tools that you can use to create your own React component library in 2023.
1. Storybook
Storybook is a popular open-source tool for developing UI components in isolation. With Storybook, you can create a living style guide for your React components. The tool enables you to develop and test your components in isolation, without having to worry about the rest of your application.
One of the key benefits of using Storybook is that it helps you document your components. You can add documentation and usage examples for each component, making it easier for other developers to use your library. Storybook also has a built-in testing tool, which makes it easy to test your components and ensure they work as expected.
To get started with Storybook, you need to install it as a dependency in your project:
npm install --save-dev @storybook/react
Once installed, you can create a configuration file for Storybook:
// .storybook/main.js module.exports = { stories: ['../src/**/*.stories.js'], addons: ['@storybook/addon-actions', '@storybook/addon-links'], }; Next, you can create a stories file for each of your components: javascript // src/components/Button/Button.stories.js import React from 'react'; import Button from './Button'; export default { title: 'Button', component: Button, }; export const Default = () => <Button>Click me</Button>; export const Disabled = () => <Button disabled>Disabled</Button>;
In the example above, we’ve created stories for a Button component. We’ve defined the component’s title and imported the Button component from our project. We’ve also defined two stories: Default and Disabled. These stories show the Button component with different props.
Once you’ve created stories for your components, you can run Storybook:
npm run storybook
This will start the Storybook server, and you can view your components in the browser.
2. Bit
Bit is a powerful tool for building and sharing reusable components. With Bit, you can create a component library that can be used across multiple projects. You can also collaborate with other developers and share your components with the wider community.
One of the key benefits of using Bit is that it helps you manage your components’ dependencies. Bit makes it easy to install and manage the dependencies for each component. This helps to keep your components lightweight and reduces the risk of conflicts.
To get started with Bit, you need to install it globally:
npm install bit -g
Next, you can initialize a Bit workspace in your project:
bit init
This will create a new Bit workspace in your project. You can then create a new component using the following command:
bit create react-component my-component
This will create a new component called my-component. You can then add the component to your Bit workspace:
bit add src/components/my-component
This will add the component to your Bit workspace and create a new Bit scope. You can then export the component to your Bit scope:
bit export <your-username>.<your-scope>
This will export the component to your Bit scope, making it available for other developers to use.
To use a Bit component in your project, you can install it as a dependency:
npm install @<your-username>/<your-scope>.my-component
You can then import and use the component in your code:
import React from 'react'; import MyComponent from '@<your-username>/<your-scope>.my-component'; function App() { return <MyComponent />; }
3. Rollup
Rollup is a module bundler that’s designed to create efficient and optimized JavaScript bundles. Rollup is a great choice for building React component libraries because it helps to keep your bundle size small and optimized.
One of the key benefits of using Rollup is that it supports tree shaking. Tree shaking is a process that removes unused code from your bundle, making it smaller and faster to load. This can help to improve the performance of your React components.
To use Rollup in your project, you need to install it as a dependency:
npm install rollup --save-dev
You can then create a configuration file for Rollup:
// rollup.config.js import babel from 'rollup-plugin-babel'; import resolve from '@rollup/plugin-node-resolve'; import commonjs from '@rollup/plugin-commonjs'; export default { input: 'src/index.js', output: [ { file: 'dist/my-component.js', format: 'cjs', }, { file: 'dist/my-component.esm.js', format: 'esm', }, ], plugins: [ babel({ exclude: 'node_modules/**', }), resolve(), commonjs(), ], external: ['react', 'react-dom'], };
In the example above, we’ve created a Rollup configuration file for a component called my-component. We’ve defined two output formats: CommonJS and ECMAScript modules. We’ve also added plugins for Babel, resolving modules, and converting CommonJS modules to ES modules. Finally, we’ve defined the external dependencies of the component: react and react-dom.
Once you’ve created a Rollup configuration file, you can build your component library:
rollup -c
This will build your component library and create two output files:
dist/my-component.js and dist/my-component.esm.js.
4. React Styleguidist
React Styleguidist is a tool for building living style guides for React components. With React Styleguidist, you can create a style guide for your components that’s easy to maintain and update. You can also document your components and provide usage examples.
One of the key benefits of using React Styleguidist is that it makes it easy to develop your components in isolation. You can see how your components look and behave without having to worry about the rest of your application. React Styleguidist also has a built-in testing tool, which makes it easy to test your components and ensure they work as expected.
To get started with React Styleguidist, you need to install it as a dependency:
npm install react-styleguidist --save-dev
Next, you can create a configuration file for React Styleguidist:
// styleguide.config.js module.exports = { components: 'src/components/**/*.js', theme: { color: { link: 'tomato', }, }, };
In the example above, we’ve defined the components directory and added a custom theme for the style guide. Once you’ve created a configuration file, you can start the style guide:
styleguidist server
This will start the style guide server, and you can view your components in the browser.
5. React Docgen
React Docgen is a tool for extracting documentation from React components. With React Docgen, you can extract information about your components’ props, methods, and events. You can then use this information to generate documentation for your component library.
One of the key benefits of using React Docgen is that it automates the process of creating documentation. You don’t have to manually create documentation for each component. Instead, React Docgen extracts the necessary information from your components and generates documentation for you.
To use React Docgen, you need to install it as a dependency:
npm install react-docgen --save-dev
Next, you can create a script to generate documentation for your components:
// scripts/generate-docs.js const fs = require('fs'); const reactDocgen = require('react-docgen'); const componentPath = process.argv[2]; fs.readFile(componentPath, (err, content) => { const parsed = reactDocgen.parse(content); console.log(JSON.stringify(parsed, null, 2)); });
In the example above, we’ve created a script that reads a component file and extracts documentation using React Docgen. To run the script, you can pass the path to your component file as an argument:
node scripts/generate-docs.js src/components/Button/Button.js
This will generate documentation for the Button component and output it to the console.
6. Framer Motion
Framer Motion is a powerful animation library for React. With Framer Motion, you can create animations that are easy to use and customize. You can also create complex animations with ease, thanks to Framer Motion’s intuitive API.
One of the key benefits of using Framer Motion is that it integrates well with React. You can use Framer Motion to create animations for your React components, and the animations will work seamlessly with the rest of your React code. Framer Motion also has excellent documentation and a vibrant community, making it a great choice for building animations in React.
Here’s an example of using Framer Motion to create a simple animation for a button component:
import { motion } from "framer-motion"; function Button({ children }) { return ( <motion.button whileHover={{ scale: 1.1 }} whileTap={{ scale: 0.9 }} > {children} </motion.button> ); }
In this example, we’ve imported the motion component from Framer Motion and used it to create an animated button component. We’ve added two animation effects to the button: whileHover and whileTap. These effects make the button scale up when the user hovers over it and scale down when the user clicks it.
7. Emotion
Emotion is a popular CSS-in-JS library for React. With Emotion, you can write CSS styles in JavaScript and use them to style your React components. Emotion has a powerful API and supports many CSS features, making it a great choice for building complex UIs.
One of the key benefits of using Emotion is that it helps you keep your styles organized and reusable. You can define your styles in JavaScript files and use them across multiple components. Emotion also has excellent performance and supports server-side rendering, making it a great choice for building high-performance React applications.
Here’s an example of using Emotion to style a button component:
import { css } from "@emotion/react"; const buttonStyles = css` background-color: blue; color: white; font-size: 1rem; padding: 0.5rem 1rem; border: none; border-radius: 4px; cursor: pointer; &:hover { background-color: darkblue; } `; function Button({ children }) { return <button css={buttonStyles}>{children}</button>; }
In this example, we’ve defined a set of styles for a button component using Emotion’s css function. We’ve then passed these styles to the css prop of our button component. This makes it easy to reuse the styles across multiple components.
8. Docz
Docz is a tool for creating beautiful documentation sites for your React components. With Docz, you can create a documentation site that’s easy to navigate and looks great. You can also document your components and provide usage examples.
One of the key benefits of using Docz is that it’s easy to set up and use. You can create a new Docz project in minutes and start documenting your components right away. Docz also has a powerful theming system and supports many customization options, making it easy to create a documentation site that matches your brand.
Here’s an example of using Docz to create documentation for a button component:
import React from "react"; /** * A simple button component. */ export default function Button({ children }) { return <button>{children}</button>; }
In this example, we’ve documented our button component using JSDoc comments. We’ve added a description of the component and its props, making it easy for other developers to use. We can then use Docz to generate documentation for our component library:
npx docz build
This will generate a static documentation site for our component library, which we can then deploy to a hosting service.
9. React Testing Library
React Testing Library is a tool for testing React components. With React Testing Library, you can write tests that simulate user interactions with your components. You can also test your components’ behavior and ensure they work as expected.
One of the key benefits of using React Testing Library is that it encourages testing your components from the user’s perspective. Instead of testing implementation details, you write tests that check if your components behave correctly when the user interacts with them. This makes your tests more reliable and helps you catch bugs before they make it to production.
Here’s an example of using React Testing Library to test a button component:
import { render, fireEvent } from "@testing-library/react"; import Button from "./Button"; test("renders a button with the given text", () => { const { getByText } = render(<Button>Click me</Button>); const button = getByText("Click me"); expect(button).toBeInTheDocument(); }); test("calls the onClick handler when the button is clicked", () => { const handleClick = jest.fn(); const { getByText } = render(<Button onClick={handleClick}>Click me</Button>); const button = getByText("Click me"); fireEvent.click(button); expect(handleClick).toHaveBeenCalledTimes(1); });
In this example, we’ve written two tests for our button component. The first test checks if the button renders correctly with the given text. The second test checks if the onClick handler is called when the button is clicked. We use the render function from React Testing Library to render our component, and the fireEvent function to simulate a click on the button.
10. React Sketch.app
React Sketch.app is a tool for designing and prototyping React components in Sketch. With React Sketch.app, you can create designs for your React components and see how they look in the context of your application.
One of the key benefits of using React Sketch.app is that it helps you design your components in a familiar environment. Sketch is a popular design tool for UI designers, and React Sketch.app makes it easy to use Sketch to design your React components. You can also use React Sketch.app to create interactive prototypes of your components, making it easier to get feedback from stakeholders.
Here’s an example of using React Sketch.app to create a design for a button component:
import React from "react"; import { render, Artboard, Text, View } from "react-sketchapp"; import Button from "./Button"; function ButtonDesign() { return ( <Artboard name="Button"> <View style={{ padding: 20 }}> <Button>Click me</Button> </View> <Text style={{ marginTop: 20 }}>Button design</Text> </Artboard> ); } render(<ButtonDesign />);
In this example, we’ve created a design for our button component using React Sketch.app. We’ve defined an Artboard component that represents our design canvas. We’ve also added a View component that contains our button component, and a Text component that describes our design. We can then use the render function from React Sketch.app to render our design and export it to a Sketch file.
Conclusion
In this blog post, we’ve covered 10 tools that can help you build React component libraries in 2023. These tools range from documentation and testing tools to animation and design tools. With these tools, you can create high-quality and scalable React components that can be used across multiple projects.
When building a React component library, it’s important to choose the right tools for your needs. Storybook and React Styleguidist are great choices for documenting your components and developing them in isolation. Bit and Rollup can help you create optimized and shareable components. Emotion can help you keep your styles organized and reusable, while Framer Motion can help you create powerful animations for your components. Finally, React Docgen, React Testing Library, and React Sketch.app can help you test and design your components with ease.
No matter what tools you choose, building a React component library is a great way to improve your front-end development skills and contribute to the React community. We hope this blog post has given you some ideas for tools to use in your next component library project!
Table of Contents
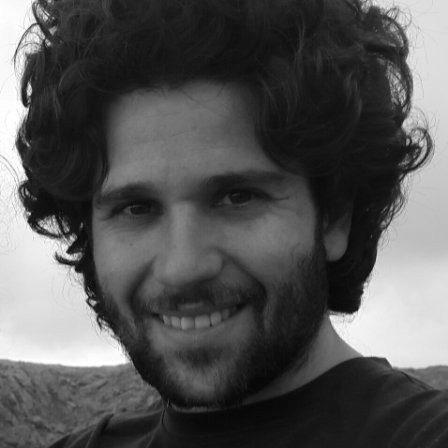
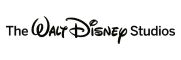