7 Reasons to Use TypeScript with React
TypeScript has gained a lot of popularity among front-end developers in recent years due to its benefits of static typing, improved code readability, and better tooling support. According to the Stack Overflow Developer Survey 2021, TypeScript was the 4th most loved programming language among developers.
Table of Contents
This popularity has also extended to the React community, where many developers have embraced TypeScript as a way to improve the scalability and maintainability of their applications. In fact, many popular React libraries such as Material-UI and Redux now offer TypeScript support, and the React team itself has provided official support for TypeScript since version 16.8.
Overall, the combination of TypeScript and React has become a powerful tool for developers looking to build scalable, maintainable, and robust front-end applications.
TypeScript is a statically typed superset of JavaScript that provides additional features such as type annotations, interfaces, and classes. It can help catch errors at compile-time rather than at runtime, making it easier to maintain and scale large applications.
Why use TypeScript with React?
React is a popular JavaScript library for building user interfaces. While React itself does not require the use of TypeScript, using TypeScript with React can provide several benefits:
1. Better support for JSX
One of the reasons to use TypeScript with React is its better support for JSX, the syntax used in React for defining and rendering components. TypeScript provides enhanced type checking and error reporting for JSX, which can help catch errors earlier in the development process and improve code quality.
Some of the ways in which using TypeScript with React can benefit you are by better type checking for JSX code, helping reduce runtime errors, improved IntelliSense in your code editor making it easier to write and debug code, catching and reporting JSX-specific syntax errors and by allowing you to define custom JSX elements which allow for better type checking and error reporting.
2. Easily readable components
One of the key benefits of using TypeScript with React is that it provides better code readability and maintainability, which can make components easier to understand and comprehend.
One of the ways TypeScript does this is by making it easy to define prop types for your React components, which can make your code easier to read and use. By defining prop types, you can specify the expected types and shapes of the data that your components receive, helping to ensure that they are used correctly, and reducing the likelihood of errors.
Overall, defining prop types with TypeScript can make your code easier to read and use by providing better documentation, type checking, and IDE support. By specifying the expected types and shapes of the data that your component needs, you can improve code quality and reduce the likelihood of errors.
Here’s an example of how defining prop types with TypeScript can make your code easier to read and use:
import React from 'react'; interface MyComponentProps { name: string; age: number; hobbies: string[]; } const MyComponent = (props: MyComponentProps) => { return ( <div> <h2>{props.name}</h2> <p>Age: {props.age}</p> <p>Hobbies:</p> <ul> {props.hobbies.map((hobby) => ( <li key={hobby}>{hobby}</li> ))} </ul> </div> ); }; export default MyComponent;
In this example, we’re defining an interface called MyComponentProps that describes the prop types for MyComponent. We’re specifying that the name prop is a string, the age prop is a number, and the hobbies prop is an array of strings.
By defining these prop types, we’re providing documentation for the component and making it clear to other developers (and our future selves) what data the component expects. We’re also enabling TypeScript’s type checking to ensure that the component is used correctly and reducing the likelihood of runtime errors.
In the body of the component, we’re using the prop values to render the component’s UI. By accessing the props through their type-checked names (props.name, props.age, and props.hobbies), we’re ensuring that the data is used correctly and reducing the likelihood of errors.
Overall, by defining prop types with TypeScript, we’re improving the readability and maintainability of our code, and reducing the likelihood of errors.
3. TypeScript support present in common libraries
One of the advantages of using TypeScript with React is that many popular React libraries and tools have built-in TypeScript support. This means that you can use TypeScript with these libraries and get type-checking and IntelliSense for their APIs out of the box.
For example, popular state management libraries like Redux and MobX have official TypeScript typings available, allowing you to get type checking and auto-completion for your Redux or MobX code. Similarly, many UI component libraries like Material UI, Ant Design, and Semantic UI React have TypeScript typings available, allowing you to get type checking and auto-completion for their component APIs.
Using TypeScript with these libraries can help catch errors early and make your code more maintainable and readable, as you can take advantage of TypeScript’s strong typing and IDE support to write safer and more efficient code.
Additionally, many popular React tools like Webpack, Babel, and ESLint also have built-in TypeScript support, making it easier to integrate TypeScript into your React project and ensuring that your build and linting processes work seamlessly with TypeScript.
4. Catching errors earlier
Using TypeScript with React can help catch errors earlier by providing static type checking. TypeScript checks the types of variables, function arguments, and function return types at compile-time. This means that any type-related errors are caught before the code is executed, which can save a lot of time and effort in debugging. For example, if a variable is expected to be a string but is assigned a number value instead, TypeScript will throw an error at compile time, allowing the developer to fix the error before the code is executed.
In a React application, using TypeScript can also help catch errors related to component props and state, making it easier to maintain and scale the application. By defining the types of props and state in interfaces, TypeScript can ensure that components are used correctly and that any type-related errors are caught early in the development process. This can help prevent errors from propagating through the application and causing more serious issues later on.
5. Improved code readability and maintainability
Using TypeScript with React can improve code readability and maintainability in several ways. One of the main benefits of TypeScript is its ability to add static typing to JavaScript code, which can make the code more self-documenting. By defining the types of variables, functions, and components, TypeScript can make the code easier to read and understand, especially for developers who are new to the project. Additionally, TypeScript can help enforce consistency in code style and structure, which can make the code easier to maintain over time.
Another way that using TypeScript with React can improve code maintainability is through its support for interfaces. Interfaces provide a way to define the shape of objects, which can be particularly useful in a React application where components often receive complex data structures as props. By defining interfaces for these data structures, TypeScript can ensure that components are used correctly and can provide helpful error messages when a component is not used as intended.
Finally, using TypeScript with React can improve code maintainability by catching errors earlier in the development process. By catching errors at compile-time, developers can avoid introducing bugs into the codebase and can spend less time debugging issues that could have been caught earlier.
6. Better IDE support
Using TypeScript with React can provide better IDE support, which can make it easier to write and debug code. IDEs such as Visual Studio Code have built-in support for TypeScript, which provides features such as autocompletion, type checking, and error highlighting. These features can make it easier to write code by providing helpful suggestions and reducing the number of syntax errors that can be introduced.
In a React application, using TypeScript can also provide better IDE support for components. By defining the types of component props and state, TypeScript can provide helpful suggestions when working with these components in the code editor. This can help avoid common errors and can make it easier to work with complex component hierarchies.
Another way that using TypeScript with React can improve IDE support is through its support for interfaces. Interfaces can provide a way to define the shape of objects, which can be particularly useful when working with large and complex data structures. By defining interfaces for these data structures, TypeScript can provide autocomplete suggestions and type checking, which can reduce the chance of errors and make it easier to work with these data structures in the code editor.
7. Enhanced collaboration:
Using TypeScript with React can enhance collaboration between developers by providing a clear and consistent type system that can be easily understood by all team members. When using TypeScript, developers can define the types of variables, functions, and components in a way that is self-documenting and easy to understand. This can reduce the need for extensive documentation and can make it easier for developers to understand each other’s code.
In a React application, using TypeScript can also enhance collaboration by providing a way to define interfaces for components and data structures. These interfaces can provide a clear contract for how components should be used and how data should be structured, making it easier for different developers to work on different parts of the codebase without introducing errors.
Another way that using TypeScript with React can enhance collaboration is through its support for tools such as linters and code formatters. These tools can help ensure that the codebase is consistent and follows best practices, which can make it easier for developers to work together and understand each other’s code.
Finally, using TypeScript with React can enhance collaboration by catching errors earlier in the development process. By catching errors at compile-time, developers can avoid introducing bugs into the codebase that could cause issues for other team members. This can help reduce the need for extensive debugging and can make it easier for developers to work together on complex features.
Challenges to Overcome When Using TypeScript with React
While using TypeScript with React can provide many benefits, there are also some challenges that developers may need to overcome. Some of these challenges include:
1. Learning curve
Developers who are new to TypeScript may need to spend some time learning the syntax and structure of TypeScript, which can be different from traditional JavaScript.
2. Integration with the existing codebase
If a project already has a large codebase written in JavaScript, integrating TypeScript can be challenging. It may require rewriting parts of the codebase or using tools such as conversion scripts to gradually transition to TypeScript.
3. Limited third-party library support
While TypeScript has been gaining popularity in recent years, not all third-party libraries have TypeScript definitions available. This can make it challenging to integrate these libraries into a TypeScript project and may require writing custom type definitions.
4. Type annotation overhead
Adding type annotations to every variable, function, and component can add overhead to the development process. While this overhead can pay off in improved code readability and maintainability, it can also slow down the development process.
5. Increased build time
TypeScript requires a build step to compile TypeScript code into JavaScript. This can increase build times, especially for larger projects.
While these challenges may be significant, they can be overcome with proper planning and attention to detail. With the right tools and resources, using TypeScript with React can provide many benefits and improve the overall quality of the codebase.
Overall, using TypeScript with React can help you write better code, catch errors earlier, and improve collaboration and maintainability.
Conclusion
There are many compelling reasons to use TypeScript with React. TypeScript can help catch errors earlier, improve code readability and maintainability, enhance collaboration, and provide better IDE support. It can also make it easier to gradually adopt TypeScript into existing projects and take advantage of the built-in TypeScript support present in common React libraries and tools.
By leveraging TypeScript’s static type-checking and IntelliSense capabilities, developers can write safer, more efficient, and more scalable code. TypeScript can also help teams work more efficiently and collaboratively, reducing the time and effort required to debug and maintain code.
Overall, whether you’re building a small React application or a large-scale enterprise project, TypeScript can help you write better code faster, with fewer errors and a more maintainable codebase. With its growing popularity and widespread adoption, TypeScript is an increasingly essential tool for modern React developers looking to build high-quality, scalable applications.
Table of Contents
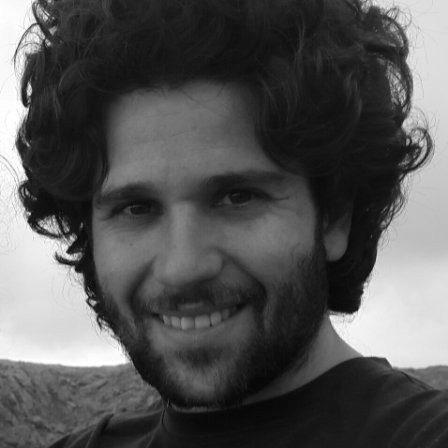
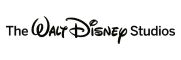