Potential of animations in ReactJS on building custom animation hooks
ReactJS, developed by Facebook, is a JavaScript library for building user interfaces, primarily for single-page applications. It’s used for handling the view layer for web and mobile apps and allows developers to create reusable UI components. With its efficient and flexible nature, it has become one of the most popular libraries among developers worldwide.

In the context of web applications, animations play a vital role in enhancing the user experience. They add a layer of sophistication and dynamism to web interfaces, making them more interactive and engaging. Moreover, animations can guide users, give feedback, and smooth transitions, improving the overall usability of the app.
In this blog post, we will delve into the world of animations in ReactJS, specifically focusing on a powerful tool at our disposal – custom animation hooks. We’ll kick things off by exploring the basics of animations in ReactJS and the concept of hooks. From there, we’ll venture into the realm of custom hooks, and then we’ll get hands-on by building a ‘useAnimation’ custom hook from scratch. You will also see this hook in action within a ReactJS app, learn best practices for creating custom hooks for animations, troubleshoot common issues, and understand the performance considerations. By the end, you’ll have a clear understanding of custom animation hooks and how they can help bring your ReactJS applications to life.
Basics of Animations in ReactJS
Basics of Animations in ReactJS
In the realm of web development, animations significantly contribute to the overall user experience. They make your applications more engaging, interactive, and user-friendly. By using animations, you can guide users’ attention, provide feedback on interactions, maintain continuity when changing views, and make the application more intuitive.
For instance, animations can be used to show the loading status of a component, transition between routes, confirm form submission, or subtly direct a user’s attention towards a call-to-action.
Different Ways to Implement Animations in ReactJS
Implementing animations in ReactJS can be accomplished in several ways. These methods range from traditional CSS animations to JavaScript libraries, and to the libraries specifically built for ReactJS.
1. Traditional CSS Animations
CSS animations are the most basic way of adding animations to your ReactJS components. You can use standard CSS properties like `transition` or `animation` to animate the changes in CSS properties over time. These animations can be added directly to the className of the ReactJS components.
2. JavaScript Libraries
There are several JavaScript libraries like GSAP (GreenSock Animation Platform), anime.js, and mo.js that offer more control and complex animations compared to traditional CSS. They give you the ability to animate almost any property over time and provide many utilities to make complex animations easier to manage.
3. React-Specific Libraries
React-specific libraries, such as `react-transition-group` or `react-spring`, have been built to work seamlessly within React’s rendering cycle. These libraries utilize the power of React and JavaScript to offer more performant and flexible animation solutions.
“React-transition-group” provides a simple way to animate entering and exiting React components with transition effects. ‘react-spring’, on the other hand, uses spring physics for animations, offering a more natural look and feel.
Each of these methods has its use cases, pros, and cons. The choice depends on your specific needs, complexity of animations, and the performance considerations of your application.
Understanding Hooks in ReactJS
React Hooks were introduced in React 16.8 as a way to let you use state and other React features without writing a class. Before Hooks, stateful logic was confined to class components, making it challenging to reuse or test that logic. Hooks are functions that let you ‘hook into’ React state and lifecycle features from function components, thus improving code reusability and readability.
Here are the basic hooks that come with React:
1. useState
This hook lets you add state to your functional components. The ‘useState’ function returns an array with two elements: the current state and a function to update it.
const [count, setCount] = useState(0);
In the above example, `count` is the state variable that is initially set to `0`, and `setCount` is the function we use to update the state.
2. useEffect
This hook lets you perform side effects in your components. Side effects could be anything from data fetching, subscriptions, or manually changing the DOM.
useEffect(() => {
document.title = `You clicked ${count} times`;
}, [count]);
In the above example, the ‘useEffect’ hook will run after every render where the “count” state has changed. The ‘count’ variable is a dependency of this effect.
These hooks, along with other built-in hooks like ‘useContext’, ‘useReducer’, etc., make it easier to manage state and side effects in React function components. They enable developers to write clean and maintainable code in React, enhancing the overall development process.
Introduction to Custom Hooks
Custom Hooks in React are a powerful feature that allow developers to extract and reuse stateful logic between different components without causing a render or changing the component hierarchy – essentially treating logic as a standalone, reusable piece. Custom Hooks are, essentially, JavaScript functions that start with ‘use’ and can call other Hooks.
Custom Hooks allow you to share complex logic across multiple components. Before Hooks were introduced, patterns like render props and higher-order components were used to share stateful logic between components, but these patterns require restructuring components, which can be cumbersome and make the code harder to follow. With custom Hooks, you avoid the need to add unnecessary components to your tree.
Advantages of Custom Hooks
1. Code Reusability
Custom Hooks help to encapsulate complex logic into a single function that can be reused across multiple components, helping to keep the code DRY (Don’t Repeat Yourself).
2. Separation of Concerns
You can separate the logic from the UI, making your components cleaner, easier to understand, and more focused on rendering.
3. Simplicity
Custom Hooks remove the need to use render props or higher-order components, simplifying your component tree and making your code easier to read.
4. Improved Testability
By extracting logic into custom Hooks, you can write tests specifically for that logic without needing to test the component that uses it.
5. State Sharing
Custom Hooks can share stateful logic, not just stateless logic. This is something that wasn’t possible with utility libraries.
In summary, Custom Hooks offer a much-needed level of abstraction, promo code reusability, and lead to cleaner, more maintainable codebases.
Building the useAnimation Custom Hook
Building a custom hook involves abstracting the common logic that can be reused across multiple components. Let’s build a “useAnimation” hook that will trigger CSS animations on demand. We’ll create a hook that adds an animation class to an element for a specified duration and then removes it.
First, we need to import the necessary hooks from React.
import { useState, useEffect } from 'react';
Next, we’ll define the “useAnimation”hook. This hook takes two arguments – “animationName”, the name of the CSS animation to apply, and “duration”, the duration of the animation in milliseconds.
const useAnimation = (animationName, duration) => {
const [animate, setAnimate] = useState(false);
useEffect(() => {
let timeoutId;
if (animate) {
timeoutId = setTimeout(() => setAnimate(false), duration);
}
return () => clearTimeout(timeoutId);
}, [animate, duration]);
return [animate, () => setAnimate(true)];
};
In the `useAnimation` hook, we initialize the `animate` state to `false`. When `animate` is `true`, the `useEffect` hook starts a timer for the specified duration. Once the timer is up, it sets `animate` back to `false`. We return the timer’s cleanup function from `useEffect` to ensure the timer is cleared if the component unmounts before the timer is up.
The hook returns an array containing the `animate` state and a function to set `animate` to `true`, triggering the animation.
Now, you can use the `useAnimation` hook in your components to apply an animation for a specified duration.
import React from 'react';
import useAnimation from './useAnimation';
const AnimatedComponent = () => {
const [animate, triggerAnimation] = useAnimation('bounce', 1000); return ( <div className={animate ? 'bounce' : ''} onClick={triggerAnimation}>
Click me
</div>
);
};
export default AnimatedComponent;
In `AnimatedComponent`, we use the `useAnimation` hook to apply a ‘bounce’ animation for 1 second whenever the `div` is clicked.
Using the useAnimation Custom Hook in a ReactJS App
Using the `useAnimation` hook in a ReactJS app is a simple process. Once you’ve defined the hook, it can be imported into any component that needs to use it.
To illustrate this, let’s create a simple React app that has a button. This button will bounce when it is clicked, using the `useAnimation` hook we’ve just defined.
First, ensure you’ve defined your CSS animation in your styles. Here’s an example of a simple bounce animation in CSS:
@keyframes bounce {
0% {transform: scaleY(1);}
50% {transform: scaleY(0.5);}
100% {transform: scaleY(1);}
}
.bounce {
animation-name: bounce;
animation-duration: 1s;
}
Now, let’s see how to use the `useAnimation` hook in our React component:
import React from 'react';
import useAnimation from './useAnimation';
import './App.css'; // import CSS
const App = () => {
// Use the hook with the 'bounce' animation and a duration of 1000ms (1 second)
const [animate, triggerAnimation] = useAnimation('bounce', 1000);
return (
<div className="App">
<button
className={animate ? 'bounce' : ''}
onClick={triggerAnimation}>
Click me to bounce
</button>
</div>
);
};
export default App;
In this `App` component, we are importing the `useAnimation` custom hook and the CSS file that includes the bounce animation.
We call the `useAnimation` hook with ‘bounce’ as the animation name and 1000 (representing 1000ms or 1s) as the duration. The hook provides us with the `animate` state and `triggerAnimation` function.
The button element uses `animate` to conditionally apply the ‘bounce’ CSS class, which triggers the bounce animation. The `onClick` prop of the button is set to `triggerAnimation`, so the animation starts when the button is clicked.
In this way, the `useAnimation` hook provides a simple, reusable method to apply animations to elements in a ReactJS application.
Conclusion
In essence, animations in ReactJS greatly enhance user experience, and with custom hooks like the `useAnimation` we’ve explored, you can create dynamic and interactive applications. By understanding and leveraging these tools, you’re equipped to construct applications that are not only visually appealing but also maintainable and efficient. Therefore, don’t hesitate to harness the power of ReactJS and its hook system, and venture into creating your unique custom animation hooks. It’s time to bring your web applications to life in a truly engaging way.
Table of Contents
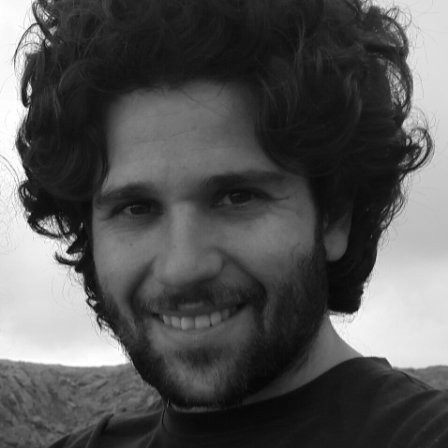
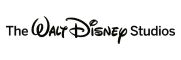