Enhance user interaction in your ReactJS application with ‘useClickOutside’
ReactJS provides tools for dynamic, interactive UI components. Detecting clicks outside a component is crucial for functions like closing dropdowns or modals, but ReactJS doesn’t offer this natively. This blog post explores ‘useClickOutside’, a custom React Hook enabling this functionality. We’ll look at its uses, implementation, and best practices, making it a key tool in UI development and site usability improvement.
What is useClickOutside?
`useClickOutside` is a custom hook in ReactJS used to detect clicks outside of a specified component. This functionality is beneficial for many UI/UX scenarios such as dismissing dropdown menus, modal dialogs, popovers, or any other component that should be closed or hidden when a user interacts with the rest of the application.
This hook works by attaching a ‘click’ event listener to the document that triggers a specific callback when a click is detected outside of the component’s boundary. It’s important to note that this hook does not exist in the standard ReactJS library, and it needs to be implemented manually, or through external libraries that offer this functionality.
Basic Use Cases for useClickOutside
`useClickOutside` is a versatile tool in ReactJS that improves user interactions across various scenarios, including:
- Contextual Menus: It enables the automatic closure of contextual menus when a user clicks elsewhere on the screen.
- Modal or Pop-up Windows: It allows pop-up windows or modals to be closed by an outside click, improving usability.
- Autocomplete Suggestions: It can be used to hide autocomplete suggestions when a user clicks outside the field.
- Image/Content Lightboxes: It fulfills the user’s expectation of closing a lightbox by clicking outside of it.
- Collapsible Sidebars/Menus: It assists in retracting collapsible menus or sidebars when a user clicks outside these components.
Leveraging `useClickOutside` can help developers craft more intuitive, user-friendly applications, enhancing their overall user interface.
Prerequisites for Using useClickOutside
To utilize the `useClickOutside` hook in ReactJS effectively, you should have:
- ReactJS knowledge: Understanding the fundamentals of ReactJS is crucial as `useClickOutside` is specific to this library.
- Awareness of React Hooks: Familiarity with the basics of React Hooks, especially `useState` and `useEffect`, is needed as `useClickOutside` is a custom Hook.
- Proficiency in JavaScript: Good command over JavaScript, especially ES6 syntax like arrow functions and destructuring, is essential.
- Node.js and NPM/Yarn: To run a React project, Node.js and a package manager like NPM or Yarn should be installed on your system.
- React Project Setup: Knowledge of setting up a new React project using tools like Create React App (CRA) is required.
- Understanding of DOM Events: As `useClickOutside` works by detecting DOM events, having a basic understanding of them is beneficial.
With these prerequisites fulfilled, you’re well-equipped to implement `useClickOutside` in your ReactJS projects.
Implementing useClickOutside: A Step-by-Step Guide
Let’s go through a step-by-step guide on implementing the `useClickOutside` custom hook in a ReactJS project.
Step 1: Creating a Basic Component
Create a simple functional component. This can be a modal, dropdown, or any other component that you want to apply the `useClickOutside` behavior to.
function MyComponent() { return <div>My Component</div>; }
Step 2: Defining the useClickOutside Hook
Define the `useClickOutside` hook. It should take a `ref` to the component to watch and a `callback` to execute when a click outside is detected.
import { useEffect } from 'react'; function useClickOutside(ref, callback) { useEffect(() => { function handleClickOutside(event) { if (ref.current && !ref.current.contains(event.target)) { callback(); } } document.addEventListener('mousedown', handleClickOutside); return () => { document.removeEventListener('mousedown', handleClickOutside); }; }, [ref, callback]); }
Step 3: Implementing the Hook in Component
Apply the `useClickOutside` hook to your component. Here, we create a `ref` with the `useRef` hook, which is passed to `useClickOutside` along with a `callback`.
import { useRef } from 'react'; function MyComponent() { const ref = useRef(); useClickOutside(ref, () => { console.log('You clicked outside!'); }); return <div ref={ref}>My Component</div>; }
Step 4: Testing the Component Behavior
Now, when you click outside of the “My Component” div, you should see “You clicked outside!” logged in your console.
Remember to test your component thoroughly across different browsers and devices to ensure consistent behavior. This includes making sure the component behaves as expected when nested within other components.
This is a basic implementation of `useClickOutside` and it can be expanded upon to handle more complex cases or to work with other hooks.
Detailed Example: Creating a Modal with useClickOutside
Let’s create a simple modal component using the `useClickOutside` custom hook in React. In this example, we will use the `useState` hook to control the visibility of the modal, and the `useClickOutside` hook to hide the modal when a user clicks outside of it.
Step 1: Setting up the Project
Assuming that you have a React application set up and ready to go, let’s create a new component named `Modal`.
import React from 'react'; const Modal = ({ isOpen, onClose }) => { if (!isOpen) { return null; } Return ( <div> <div> <h2>Modal Window</h2> <button onClick={onClose}>Close</button> </div> </div> ); }; export default Modal;
Step 2: Building the `useClickOutside` Hook
Next, let’s create the `useClickOutside` hook.
import { useEffect } from 'react'; function useClickOutside(ref, callback) { useEffect(() => { const handleClickOutside = (event) => { if (ref.current && !ref.current.contains(event.target)) { callback(); } }; document.addEventListener('mousedown', handleClickOutside); return () => { document.removeEventListener('mousedown', handleClickOutside); }; }, [ref, callback]); } export default useClickOutside;
Step 3: Implementing the `useClickOutside` Hook in the Modal Component
Now, let’s modify the `Modal` component to use the `useClickOutside` hook.
import React, { useRef } from 'react'; import useClickOutside from './useClickOutside'; const Modal = ({ isOpen, onClose }) => { const ref = useRef(); useClickOutside(ref, onClose); if (!isOpen) { return null; } return ( <div> <div ref={ref}> <h2>Modal Window</h2> <button onClick={onClose}>Close</button> </div> </div> ); }; export default Modal;
Step 4: Testing the Modal Functionality
Finally, let’s test the modal in a parent component. The modal should now close when the user clicks outside of it or clicks the close button.
import React, { useState } from 'react'; import Modal from './Modal'; function App() { const [isModalOpen, setModalOpen] = useState(false); const openModal = () => setModalOpen(true); const closeModal = () => setModalOpen(false); return ( <div> <button onClick={openModal}>Open Modal</button> <Modal isOpen={isModalOpen} onClose={closeModal} /> </div> ); } export default App;
With this setup, you now have a functional modal component that closes when you click outside of it, thanks to the `useClickOutside` hook!
Common Issues and Solutions with useClickOutside
Implementing `useClickOutside` in ReactJS can sometimes pose challenges, including:
- Nested components: Clicks on nested components might be treated as outside clicks due to event bubbling. To avoid this, ensure nested components don’t stop event propagation, or place a separate click handler on the parent component.
- Multiple instances: If multiple components use `useClickOutside`, clicking from one to another can cause undesired behavior. To resolve this, review your component structure and hierarchy, possibly managing the state at a higher level or using context for handling interactions.
- Performance issues: Adding many instances of `useClickOutside` can potentially affect performance due to numerous listeners. To mitigate this, create a global click handler that checks a list of registered components for outside clicks instead of attaching listeners to each component instance.
- Unwanted handler firing: The outside click handler might fire even when it’s not needed, like when the component is not visible. To prevent this, add a condition in your `useEffect` dependency array to check if the component is active or visible before adding the outside click handler.
With careful implementation considering the component structure and behavior, `useClickOutside` can enhance your application’s UI/UX significantly.
Best Practices when Using useClickOutside
When implementing the `useClickOutside` hook, consider the following best practices:
- Conditional useEffect: Conditionally execute your `useEffect` hook based on component visibility to prevent unnecessary function executions.
- Remove Event Listeners: Remove event listeners when the component unmounts, using the `useEffect` hook’s cleanup function.
- Use useRef: Using `useRef` to reference DOM elements in React is more optimal than callbacks, as it doesn’t cause re-renders and stays consistent across renders.
- Nested Components: Be careful with nested components. If they prevent event propagation, the `useClickOutside` hook may not function as expected.
- Avoid Performance Issues: If multiple components use the `useClickOutside` hook, it could potentially lead to performance issues due to multiple event listeners. Implement a global click handler at a higher level instead.
- Use Consistent Naming: Maintain consistent naming for the custom hook across your application, enhancing readability and manageability of your codebase.
- Thorough Testing: Always test across different browsers and devices, ensuring the implementation works well with other components and handles different states correctly.
- Error Handling: Handle errors and edge cases, such as when the ref is not attached or the callback function isn’t provided.
Remember, `useClickOutside` is meant to enhance user experience, so ensure it’s implemented smoothly and correctly.
Conclusion
In this blog post, we delved into `useClickOutside`, a ReactJS custom hook that detects and handles clicks outside a specific component, enhancing user experience by providing an intuitive way to interact with elements such as modals, dropdowns, or contextual menus. We walked through its fundamental use cases, prerequisites, and a detailed guide to implement it. We also discussed potential issues that may arise during its use and shared best practices for efficient implementation. This tool is invaluable for developers aiming to build dynamic, user-friendly interfaces, regardless of the complexity of their projects. Always ensure to thoroughly test your components for consistent behavior across various browsers and devices.
Table of Contents
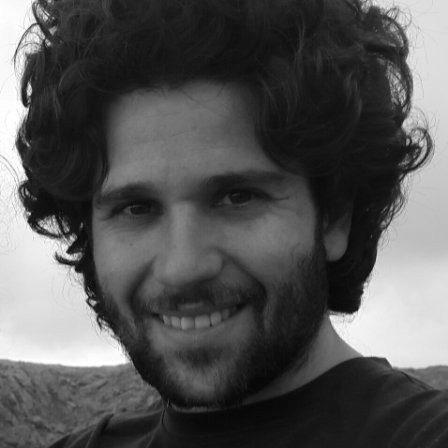
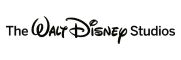