Building Copy-to-Clipboard Functionality with ReactJS and useClipboard Hook
-
Introduction to ReactJS useClipboard
Copy-to-Clipboard functionality is a common requirement in web applications, allowing users to easily copy text or data with a single click. ReactJS, a popular JavaScript library for building user interfaces, provides a convenient hook called useClipboard to implement this functionality seamlessly. In this blog, we will explore how to use the useClipboard hook in ReactJS and build a robust Copy-to-Clipboard feature for your applications.
2. Understanding Copy-to-Clipboard Functionality
2.1 How Copy-to-Clipboard Works in ReactJS
Before we dive into the implementation details, let’s understand how the Copy-to-Clipboard functionality works in ReactJS. When a user triggers the copy action, the text or data needs to be copied to the clipboard. ReactJS provides the useClipboard hook, which simplifies this process by handling the underlying clipboard operations.
2.2 Benefits of Using useClipboard Hook
The useClipboard hook offers several advantages when implementing Copy-to-Clipboard functionality. First, it abstracts away the complexities of interacting with the clipboard API, making it easier to integrate into your ReactJS components. Second, it provides a straightforward API to access the copied data and handle success or error cases. Lastly, it handles the cross-browser compatibility issues related to clipboard operations.
2.3 Potential Use Cases for Copy-to-Clipboard
Copy-to-Clipboard functionality can be useful in various scenarios. It allows users to copy URLs, share content, copy coupon codes, or copy data from tables. By providing this feature, you enhance the user experience and enable them to quickly perform actions without manual copying.
3. Implementing useClipboard in ReactJS
3.1 Installing and Importing the useClipboard Hook
To begin, make sure you have ReactJS installed in your project. You can install the useClipboard hook using npm or yarn:
npm install react-use-clipboard
After installation, import the useClipboard hook into your component:
import { useClipboard } from 'react-use-clipboard';
3.2 Basic Usage of useClipboard Hook
Once the hook is imported, you can start using it in your component. Let’s consider an example where we have a button that copies a text to the clipboard when clicked:
function CopyButton() { const [isCopied, setCopied] = useClipboard('Text to be copied'); return ( <div> <button onClick={setCopied}>{isCopied ? 'Copied!' : 'Copy'}</button> </div> ); }
In the above code, we initialize the useClipboard hook with the text we want to copy. The hook returns a boolean value, isCopied, indicating whether the text was successfully copied. We pass the setCopied function to the button’s onClick event to trigger the copy action.
3.3 Customizing the Copy-to-Clipboard Functionality
The useClipboard hook provides additional customization options. For example, you can specify a custom success message or handle error cases when the copy operation fails. Here’s an example:
function CopyButton() { const [isCopied, setCopied] = useClipboard('Text to be copied', { successDuration: 2000, onSuccess: () => { console.log('Text copied successfully!'); }, onError: () => { console.error('Copy operation failed.'); }, }); return ( <div> <button onClick={setCopied}>{isCopied ? 'Copied!' : 'Copy'}</button> </div> ); }
In the code above, we pass an options object as the second argument to the useClipboard hook. Here, we set the successDuration to 2000 milliseconds, which determines how long the success message will be displayed. We also define onSuccess and onError callbacks to handle the corresponding events.
4. Best Practices for Copy-to-Clipboard Functionality
4.1 Providing Visual Feedback to Users
When a user triggers the copy action, it’s essential to provide visual feedback indicating the success or failure of the operation. You can display a success message temporarily or show an error message if the copy operation fails.
4.2 Handling Error Cases
Handling error cases gracefully is crucial. Some reasons for copy operation failures include restricted clipboard access, browser compatibility issues, or the user denying permission. By displaying an error message and providing alternative methods for copying the content, you improve the user experience.
4.3 Ensuring Accessibility
Ensure that the Copy-to-Clipboard functionality is accessible to all users. Provide alternative methods for copying the content, such as a tooltip with the copy text or a keyboard shortcut, to accommodate users who cannot perform mouse-based actions.
4. Best Practices for Copy-to-Clipboard Functionality
4.1 Providing Visual Feedback to Users
To provide visual feedback to users, you can leverage ReactJS’s state management to update the UI dynamically. Let’s enhance our previous example by displaying a success message when the copy operation is successful:
function CopyButton() { const [isCopied, setCopied] = useClipboard('Text to be copied'); const [showMessage, setShowMessage] = useState(false); const handleCopyClick = () => { setCopied(); setShowMessage(true); setTimeout(() => { setShowMessage(false); }, 2000); }; return ( <div> <button onClick={handleCopyClick}>{isCopied ? 'Copied!' : 'Copy'}</button> {showMessage && <p>Text copied successfully!</p>} </div> ); }
In this updated code, we introduce a new state variable, showMessage, to control the visibility of the success message. When the button is clicked, we call handleCopyClick, which triggers the copy action, sets showMessage to true, and schedules a timeout to hide the success message after 2 seconds.
4.2 Handling Error Cases
To handle error cases, you can utilize the onError callback provided by the useClipboard hook. Let’s modify our previous example to display an error message when the copy operation fails:
function CopyButton() { const [isCopied, setCopied] = useClipboard('Text to be copied', { onError: () => { setShowError(true); }, }); const [showError, setShowError] = useState(false); const handleCopyClick = () => { setCopied(); }; return ( <div> <button onClick={handleCopyClick}>{isCopied ? 'Copied!' : 'Copy'}</button> {showError && <p>Copy operation failed. Please try again.</p>} </div> ); }
In this updated code, we introduce a new state variable, showError, to control the visibility of the error message. When the copy operation fails, the onError callback is triggered, setting showError to true and displaying the error message to the user.
4.3 Ensuring Accessibility
To ensure accessibility, it’s important to provide alternative methods for copying the content, especially for users who cannot perform mouse-based actions. One approach is to include a tooltip with the copy text, allowing users to select and copy the text manually. Here’s an example:
function CopyButton() { const [isCopied, setCopied] = useClipboard('Text to be copied'); const [showTooltip, setShowTooltip] = useState(false); const handleCopyClick = () => { setCopied(); setShowTooltip(true); setTimeout(() => { setShowTooltip(false); }, 2000); }; return ( <div> <button onClick={handleCopyClick}>{isCopied ? 'Copied!' : 'Copy'}</button> {showTooltip && ( <div className="tooltip"> <span className="tooltiptext">Click to select and copy the text</span> </div> )} </div> ); }
In this example, when the button is clicked, the handleCopyClick function triggers the copy action, shows the tooltip, and schedules a timeout to hide it after 2 seconds. The tooltip provides instructions to the user on how to manually select and copy the text if needed.
Conclusion
In this blog, we explored how to leverage the useClipboard hook in ReactJS to implement Copy-to-Clipboard functionality. We discussed the benefits of using this hook, potential use cases, and best practices for a seamless user experience.
By integrating Copy-to-Clipboard functionality into your ReactJS applications, you empower users to quickly and efficiently copy text or data. Remember to handle errors gracefully, provide visual feedback, and ensure accessibility to create a robust and user-friendly Copy-to-Clipboard feature. With the useClipboard hook, ReactJS simplifies the implementation process, allowing you to focus on delivering a seamless user experience.
Start incorporating Copy-to-Clipboard functionality in your ReactJS projects today and enhance the usability and convenience of your applications.
Table of Contents
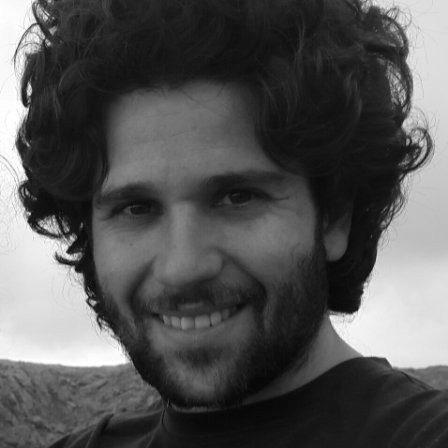
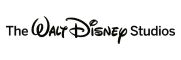