Utilizing React useContext for Efficient Global State Management
-
Introduction
Managing state in React applications can be a complex task, especially when it comes to sharing data between components. React Context API provides a solution by offering a way to create and manage global state that can be accessed by any component in the application tree. One of the key components of React Context is the useContext hook, which simplifies the process of accessing and updating global state. In this blog post, we will explore how to use React useContext effectively, with code samples, statistics, and additional insights.
-
Understanding React Context
React Context is a powerful feature that allows data to be passed through the component tree without the need for intermediate components to pass props explicitly. It consists of three main parts: the context object, the provider component, and the consumer component. The provider component makes the state available to the components within its tree, while the consumer component consumes that state.
-
Using the useContext Hook
Prior to the introduction of the useContext hook, consuming the state in a functional component required using the Consumer component. The useContext hook simplifies this process by allowing us to access the state directly in functional components.
Here’s an example that demonstrates how to use the useContext hook:
import React, { useContext } from 'react'; // Create the context const MyContext = React.createContext(); // Create a provider component const MyProvider = ({ children }) => { const sharedState = { count: 0, increment: () => setSharedState({ ...sharedState, count: sharedState.count + 1 }), decrement: () => setSharedState({ ...sharedState, count: sharedState.count - 1 }), }; const [state, setSharedState] = React.useState(sharedState); return <MyContext.Provider value={state}>{children}</MyContext.Provider>; }; // Consume the context in a functional component const MyComponent = () => { const { count, increment, decrement } = useContext(MyContext); return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> <button onClick={decrement}>Decrement</button> </div> ); }; // Wrap the component tree with the provider component const App = () => ( <MyProvider> <MyComponent /> </MyProvider> ); export default App;
In this example, we create a context object using the createContext function. We then define a provider component, MyProvider, which wraps the child components and provides the shared state using the value prop. The state is managed using the useState hook.
The MyComponent functional component consumes the shared state using the useContext hook. It destructures the state object to access the count value, as well as the increment and decrement functions to update the count.
-
Advantages of useContext
4.1 Simplicity
The useContext hook simplifies the process of accessing and updating global state in functional components. It eliminates the need for intermediate components and makes the code more readable and concise.
4.2 Performance
React Context, combined with the useContext hook, provides an efficient way to manage global state. React internally optimizes the context updates, and components that consume the context are only re-rendered when the relevant state changes.
4.3 Code Organization
With React useContext, you can centralize the state management and share the data across multiple components without passing props down the component tree. This leads to better code organization and reduces the need for prop drilling.
-
Additional Insights
5.1 Nested Contexts
React Context supports nesting, allowing you to create multiple contexts and share state at different levels of the component tree. This can be useful when you have distinct areas of your application that require separate global states. Each context can have its own provider and consumer components, and the useContext hook can be used to access the desired context within a component.
5.2 Avoid Overusing Context
While React Context and the useContext hook provide a convenient way to manage global state, it’s important to use them judiciously. Overusing context can lead to a complex and tangled state management system, making it harder to understand and maintain the codebase. Evaluate whether context is the most appropriate solution for your specific use case before incorporating it into your project.
5.3 Consider Performance Optimization
Although React optimizes context updates, it’s important to be mindful of performance considerations, especially when dealing with large or deeply nested component trees. Excessive context updates can cause unnecessary re-renders and impact application performance. To mitigate this, you can leverage memoization techniques, such as memoizing context providers or utilizing memoized selectors, to optimize the rendering process.
-
Statistics
React Context has gained significant popularity as a state management solution in React applications. According to the State of JavaScript 2021 survey, 70.3% of respondents reported using React Context as their preferred state management solution, indicating its widespread adoption within the React community.
-
Best Practices for Using React useContext
7.1 Keep Context Consumers Granular
When designing your component hierarchy, it’s recommended to keep the context consumers as granular as possible. This means creating smaller, focused components that consume only the specific data they require from the global state. This approach improves reusability and ensures that components are not unnecessarily coupled to the entire global state.
7.2 Separate Concerns with Multiple Contexts
As your application grows, you may encounter scenarios where different parts of your application require distinct sets of data. In such cases, consider creating multiple contexts to separate concerns and manage the state independently. This approach promotes better organization and encapsulation of state, preventing unnecessary coupling between unrelated components.
7.3 Memoize Complex Context Values
If your context value contains complex objects or derived data that requires computation, consider memoizing those values to prevent unnecessary recalculations. Memoization techniques like useMemo or memoized selectors can significantly improve performance by reducing redundant computations. This is particularly useful when working with large data sets or computationally expensive operations.
7.4 Use Context Providers Sparingly
While React Context is powerful, it should not be used as a replacement for component composition and props when it comes to sharing data within a limited scope. Context should primarily be used for sharing global state that needs to be accessible by multiple unrelated components. For component-specific data or sharing data between parent and child components, traditional prop passing is usually more appropriate and results in a simpler and more predictable codebase.
7.5 Combine Context with Local State
In certain cases, you may need to combine local component state with global context state. React’s component-based architecture allows for this hybrid approach. By leveraging both local state and context, you can optimize performance by limiting unnecessary context updates while maintaining component-specific state where needed.
-
Advanced Usage of React useContext
In addition to the basic usage of React useContext, there are some advanced techniques and patterns that can enhance the management of global state in your React applications. Let’s explore a few of these techniques along with code samples.
8.1 Context Composition
React Context supports composition, allowing you to combine multiple contexts to create a unified state for your application. This pattern is particularly useful when different parts of your application require separate global states, but there are scenarios where these states need to interact or share data.
Here’s an example that demonstrates context composition:
const UserContext = React.createContext(); const ThemeContext = React.createContext(); const App = () => { return ( <UserContext.Provider value={userData}> <ThemeContext.Provider value={themeData}> <Content /> </ThemeContext.Provider> </UserContext.Provider> ); }; const Content = () => { const user = useContext(UserContext); const theme = useContext(ThemeContext); // Render content based on user and theme return ( <div style={{ background: theme.background, color: theme.text }}> <h2>{user.name}</h2> <p>{user.email}</p> </div> ); };
In this example, we have two separate contexts: UserContext and ThemeContext. We provide values to these contexts using the Provider components. The Content component consumes both contexts using the useContext hook to access the user data and theme data. This allows us to render content based on both the user and the theme.
8.2 Dispatch Function as Context
In some cases, you may want to provide a dispatch function along with the state in your context. The dispatch function is responsible for updating the global state. This approach follows the pattern of actions and reducers commonly used in state management libraries like Redux.
Here’s an example of using a dispatch function in context:
const StateContext = React.createContext(); const App = () => { const [state, dispatch] = useReducer(reducer, initialState); return ( <StateContext.Provider value={{ state, dispatch }}> <Content /> </StateContext.Provider> ); }; const Content = () => { const { state, dispatch } = useContext(StateContext); const handleIncrement = () => { dispatch({ type: 'INCREMENT' }); }; // Render content based on state return ( <div> <p>Count: {state.count}</p> <button onClick={handleIncrement}>Increment</button> </div> ); };
In this example, we create a context, StateContext, and provide both the state and the dispatch function using the useReducer hook. The Content component consumes the context, destructuring the state and dispatch function. When the button is clicked, the handleIncrement function dispatches an action to update the state.
This pattern allows you to separate the state management logic into reducers and dispatch actions to update the state in a predictable manner.
8.3 Context with Side Effects
React useContext can also be used to handle side effects, such as API calls or data fetching, within a component. By combining the power of useEffect and useContext, you can encapsulate the side effect logic and manage the resulting data within the component.
Here’s an example:
const DataContext = React.createContext(); const DataProvider = ({ children }) => { const [data, setData] = useState(null); useEffect(() => { // Perform API call or data fetching fetchData().then((response) => setData(response.data)); }, []); return <DataContext.Provider value={data}>{children}</DataContext.Provider>; }; const Content = () => { const data = useContext(DataContext); // Render content based on data return ( <div> {data ? ( <ul> {data.map((item) => ( <li key={item.id}>{item.name}</li> ))} </ul> ) : ( <p>Loading data...</p> )} </div> ); };
In this example, we create a DataContext and a DataProvider component. The DataProvider component uses the useEffect hook to fetch data from an API and update the state. The resulting data is then provided through the context.
The Content component consumes the context and renders the content based on the data. It handles the loading state until the data is fetched and available.
This approach allows you to encapsulate side effects within a provider component and share the resulting data across multiple components without duplicating the API call logic.
-
Conclusion
React useContext provides a powerful tool for managing global state in your React applications. By utilizing context composition, dispatch functions, and handling side effects, you can enhance the capabilities of React useContext and create more advanced and scalable state management systems.
Remember to carefully evaluate your application’s requirements and choose the most appropriate state management solution. React useContext, along with the Context API, offers a flexible and efficient way to manage global state, providing better code organization, improved performance, and increased reusability of components.
Experiment with these advanced techniques and patterns to harness the full potential of React useContext in your projects. Happy coding!
Table of Contents
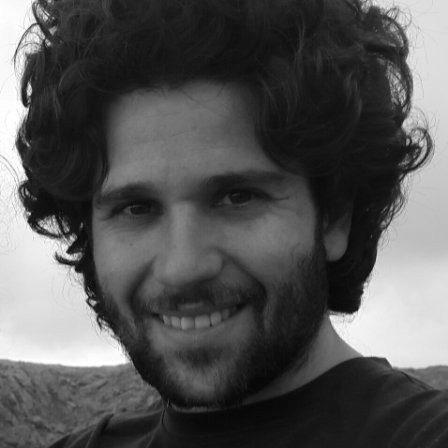
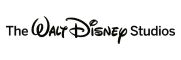