Keeping Pace with Functions: Optimizing Performance with ReactJS’s useDebounce and useThrottle Hooks
In the world of web development, it’s critical to optimize your application for the best user experience. This involves ensuring the app’s smooth running and responsive performance even in the face of numerous, rapid user interactions. For developers using ReactJS, the `useDebounce` and `useThrottle` custom hooks can help optimize application performance when dealing with rapidly changing functions. Let’s dive into their application and understand how they can make our applications more efficient and user-friendly.
Understanding Debouncing and Throttling
Before we delve into their usage in React, let’s get a clear understanding of what debouncing and throttling are.
- Debouncing: Debouncing in JavaScript is a practice used to ensure that time-consuming tasks do not fire so often. This is particularly useful for certain events that can happen faster than we can process them. Debouncing is a technique that bundles rapid successive calls of a function into single call execution after a defined amount of time.
- Throttling: Throttling, on the other hand, is a technique in which, no matter how many times the user fires the event, the attached function will execute only once in a given time interval. In simple words, throttling enforces a maximum number of times a function can be called over time.
Now, let’s dive into their usage within ReactJS.
useDebounce: The What and The How
In React, `useDebounce` is a custom hook that you can use to implement debouncing functionality. This is highly useful when you want to limit the rate at which a function is called.
Let’s imagine a search input field that triggers an API request for every keypress. If you have a high-frequency typist, you might end up with hundreds of API requests per minute. This is inefficient and can cause unnecessary load on your servers. Using the `useDebounce` hook, you can control this by delaying the execution until a certain amount of time has passed without the function being called.
Here’s how a basic implementation of a `useDebounce` hook might look:
import { useState, useEffect } from 'react'; function useDebounce(value, delay) { const [debouncedValue, setDebouncedValue] = useState(value); useEffect(() => { const handler = setTimeout(() => { setDebouncedValue(value); }, delay); return () => { clearTimeout(handler); }; }, [value, delay]); return debouncedValue; } export default useDebounce;
In the above example, the `useDebounce` hook takes in a `value` and a `delay`. It returns the debounced value. The `useEffect` hook sets a timer to update the debounced value after the delay. If the `value` changes before the delay is over, the timer is cleared and restarted.
useThrottle: The What and The How
`useThrottle` is another custom hook in React, employed to apply the throttling technique to function calls. This is beneficial for cases where you want to ensure that an event doesn’t fire more often than you can handle.
For example, a user scrolling on your website triggers scroll events many times per second. However, if you have a function attached to the scroll event (like lazy loading images), it might put heavy computation load on the browser, leading to a non-smooth scrolling experience. Throttling can help here by limiting the rate at which the function is executed.
Here is how you can implement a `useThrottle` hook:
import { useState, useEffect, useRef } from 'react'; function useThrottle(value, limit) { const [throttledValue, setThrottledValue] = useState(value); const lastRan = useRef(Date.now()); useEffect(() => { const handler = setTimeout(function() { if (Date.now() - lastRan.current >= limit) { setThrottledValue(value); lastRan.current = Date.now(); } }, limit - (Date.now() - lastRan.current)); return () => { clearTimeout(handler); }; }, [value, limit]); return throttledValue; } export default useThrottle;
In this example, the `useThrottle` hook accepts a `value` and a `limit`. It returns a throttled value that updates at most every `limit` milliseconds. The `useEffect` hook sets a timer to update the throttled value after the time limit, but only if the `limit` time has passed since it last ran. If the `value` changes before the time limit, the timer is cleared but does not restart until the `limit` time has passed.
Conclusion
Performance optimization is a critical aspect of any application development, and in the case of ReactJS, `useDebounce` and `useThrottle` provide powerful techniques for managing rapidly changing functions. They can significantly enhance the user experience, reduce unnecessary computations, and help prevent potential server overloads.
Mastering these hooks allows developers to create more efficient, optimized web applications that handle real-world user interactions smoothly. Always remember to choose the right technique for your needs. Use debouncing when you want a function to postpone its next execution until after a certain amount of time has passed without it being called, and throttling when you want to ensure that a function is not called more than once per specified period.
Keep exploring and happy coding!
Table of Contents
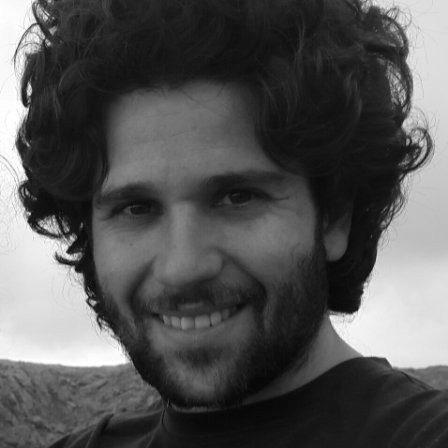
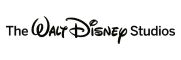