ReactJS useDebugValue: Customizing Debug Information for Hooks
-
Introduction
ReactJS is a popular JavaScript library for building user interfaces, and it provides a powerful feature called hooks. Hooks allow developers to add state and other React features to functional components. One such hook is useDebugValue, which provides a way to customize and display debug information for hooks. In this blog post, we will explore the useDebugValue hook and learn how to use it effectively.
1.1 Overview of useDebugValue
The useDebugValue hook is a built-in hook provided by ReactJS that allows developers to provide custom debug information for their hooks. It is primarily used for debugging purposes and provides a way to attach additional information to hooks that can be viewed in development tools.
1.2 Purpose and Benefits of useDebugValue
The main purpose of useDebugValue is to help developers understand and debug hooks more effectively. By providing custom debug information, developers can gain insights into the state and behavior of their hooks during development. This can be particularly useful when working with complex hooks or when debugging issues related to hook dependencies.
Some of the benefits of using useDebugValue include:
Improved debugging experience: By attaching meaningful debug information to hooks, developers can quickly identify the current state and values associated with a hook.
Customized debug output: With useDebugValue, developers have full control over what information is displayed in the development tools, making it easier to track and understand the state of hooks.
Increased code maintainability: By documenting important hook information using useDebugValue, future developers can easily understand the purpose and behavior of the hook, leading to more maintainable code.
1.3 How useDebugValue Works
The useDebugValue hook takes a single parameter, typically a value or a function, which represents the debug information to be displayed. This debug value can be of any type, such as a string, number, object, or even a function. React calls this function during rendering and displays the returned value as the debug information.
To utilize useDebugValue, developers need to invoke it within the body of their custom hook, typically after the initialization of any necessary variables or state. The debug value can be a constant or derived from the hook’s internal state or props. React automatically optimizes the calls to useDebugValue to avoid unnecessary re-evaluations.
-
Usage of useDebugValue
2.1 Syntax and Parameters of useDebugValue
The syntax for using useDebugValue is as follows:
import { useDebugValue } from 'react'; function useCustomHook() { const debugValue = // compute or initialize debug value useDebugValue(debugValue); // Rest of the custom hook's logic }
The only parameter accepted by useDebugValue is the debug value itself. This value can be a constant, a derived value, or a function that returns the debug value. It’s important to note that the debug value is only intended for debugging purposes and should not be relied upon for business logic.
2.2 Example of useDebugValue in a Custom Hook
Let’s consider an example where we create a custom hook called useCounter that provides a counter value along with its debug information. The useCounter hook takes an initial value as a parameter and returns the current counter value along with a custom debug label.
import { useState, useDebugValue } from 'react'; function useCounter(initialValue) { const [count, setCount] = useState(initialValue); const increment = () => setCount(count + 1); const decrement = () => setCount(count - 1); const debugLabel = `Counter: ${count}`; useDebugValue(debugLabel); return [count, increment, decrement]; }
In the example above, the useCounter hook uses useDebugValue to attach the debugLabel to the hook. This label will be displayed in the development tools, providing a clear understanding of the current counter value.
2.3 Best Practices for using useDebugValue
When using useDebugValue, it’s important to follow some best practices to ensure effective debugging and maintainable code. Here are a few tips:
Use descriptive debug values: Make sure to provide informative debug values that accurately describe the state or purpose of the hook. This will make it easier for developers to understand the hook’s behavior.
Avoid complex computations: While you can compute debug values using functions, try to keep the computations simple and efficient. Complex computations might impact performance and increase the overhead of rendering.
Utilize conditional debug values: In certain cases, you might want to conditionally display debug values. You can achieve this by leveraging conditional statements or other logical checks within the debug value computation.
-
Customizing Debug Information
3.1 Creating Custom Debug Values
When creating custom debug values, it’s essential to choose values that accurately represent the state or purpose of the hook. For example, if you have a hook that manages a form’s state, the debug value could be the current form values or an indicator of the form’s validity.
3.2 Providing Descriptive Information
To make the debug values more informative, you can combine multiple values or include additional context. For instance, in the useCounter example, we added the word “Counter” before the actual count value to provide a clear label in the development tools.
3.3 Leveraging useDebugValue in Custom Hooks
Custom hooks often encapsulate complex logic or handle specific concerns. By utilizing useDebugValue, you can provide custom debug information to enhance the debugging experience of the consuming components. This helps developers gain insights into the inner workings of the hook without exposing unnecessary implementation details.
Formatting Debug Values
3.4 Converting Values to Strings
When formatting debug values, it’s common to convert values to strings to ensure proper display. JavaScript provides several built-in methods for converting values to strings, such as toString() or using string interpolation. Choose the appropriate method based on the type and structure of the value.
3.5 Formatting Dates and Times
When working with date or time values, it’s important to format them in a readable way. JavaScript offers various date and time formatting libraries, such as Moment.js or Date-fns, which can be used to convert and format date objects into human-readable strings.
3.6 Displaying Complex Data Structures
If your debug values involve complex data structures, such as arrays or objects, you might need to convert them into a string representation that is more readable. JSON.stringify can be used to serialize JavaScript objects into JSON strings, making it easier to display complex data structures.
Updating Debug Values Dynamically
3.7 Conditionally Displaying Debug Values
Sometimes, you might want to conditionally display debug values based on certain conditions. To achieve this, you can incorporate conditional logic within the debug value computation. For example, you can conditionally show or hide a debug value based on the value of a state variable.
3.8 Handling State Updates
When updating the debug value based on state changes, ensure that the debug value computation depends on the relevant state variables. This ensures that the debug value is updated correctly whenever the state changes. React’s built-in dependency tracking mechanism handles this automatically.
3.9 Controlling Debug Value Frequency
In some cases, you might want to control how frequently the debug value is updated. To achieve this, you can use memoization techniques to avoid unnecessary recomputations of the debug value. Libraries like lodash.memoize or custom memoization techniques can be used to achieve efficient updates.
-
Performance Considerations
4.1 Impact on Performance
While useDebugValue is a powerful debugging tool, it’s important to consider its impact on performance. Debug values are computed during rendering, so if the computation is expensive or involves heavy operations, it can affect the overall performance of your application. It’s recommended to keep the debug value computation lightweight and efficient.
Additionally, keep in mind that using useDebugValue in development environments may have a negligible impact on performance. However, make sure to remove or optimize debug value computations in production builds to avoid any unnecessary overhead.
4.2 Limitations and Trade-offs
Although useDebugValue provides valuable debug information, it does have some limitations. First, it only works in development mode and does not have any effect in production builds. This ensures that the debug values do not impact the performance of the deployed application.
Second, the debug values are only accessible through development tools, such as browser extensions or React DevTools. This means that the debug information is not directly available within the application’s UI, so it’s crucial to ensure that the debug values are meaningful and comprehensive enough for debugging purposes.
4.3 Tips for Optimizing Performance with useDebugValue
To optimize performance while using useDebugValue, consider the following tips:
Keep the debug value computation simple and efficient. Avoid complex computations or heavy operations.
Memoize the debug value computation using memoization techniques to prevent unnecessary re-computations.
Use conditional rendering within the debug value computation to control when the debug value is displayed.
Remove or optimize debug value computations in production builds to minimize performance impact.
-
Troubleshooting and Common Pitfalls
5.1 Debugging Issues with useDebugValue
While using useDebugValue, you may encounter some issues or unexpected behavior. Here are some common debugging techniques to address such issues:
Verify that you are using useDebugValue correctly and that it is placed within the body of your custom hook.
Double-check the dependencies of your debug value computation. Ensure that it correctly depends on the relevant state variables or props to update the debug value as expected.
Use console.log statements or debugging tools to inspect the values of variables used in the debug value computation.
If you are still facing issues, consult the React documentation or seek help from the community to troubleshoot specific problems you may encounter.
5.2 Common Mistakes to Avoid
While using useDebugValue, there are some common mistakes that developers should avoid:
Forgetting to invoke useDebugValue within the custom hook’s body. Ensure that you call useDebugValue with the appropriate debug value to display the desired information.
Overcomplicating the debug value computation. Remember to keep the computation simple and focused on providing.
-
Alternative Approaches
6.1 Other Debugging Techniques in ReactJS
While useDebugValue is a powerful tool for providing custom debug information, ReactJS offers other debugging techniques that can be used in conjunction with or as alternatives to useDebugValue. Some of these techniques include:
Console logging: Using console.log statements strategically throughout your code can help you track the flow of data and debug specific issues. You can log relevant values, state changes, or function calls to gain insights into the behavior of your application.
React DevTools: React DevTools is a browser extension that provides a set of debugging tools specifically designed for React applications. It allows you to inspect components, view props and state, and analyze component hierarchies. Combining useDebugValue with React DevTools can provide a comprehensive debugging experience.
React Profiler: The React Profiler is another tool provided by React DevTools that helps you identify performance bottlenecks and optimize your application. It allows you to record and analyze the rendering time of components, helping you identify areas that require optimization.
6.2 Comparing useDebugValue with DevTools
While useDebugValue focuses on providing custom debug information for individual hooks, React DevTools offers a broader range of debugging capabilities. React DevTools provides a comprehensive view of your entire React component tree, allowing you to inspect and debug at a higher level.
Use useDebugValue when you need to provide specific debug information for a particular hook. However, when you need to inspect the overall state and behavior of your application, React DevTools should be your go-to tool.
6.3 Evaluating the Use of Custom Debug Information
When deciding whether to use custom debug information, consider the following factors:
Complexity of your hooks: If your hooks manage complex state or have intricate dependencies, custom debug information can be invaluable in understanding their behavior.
Development team size: Custom debug information can be particularly helpful in large development teams where multiple developers may need to understand and debug the same hooks.
Debugging needs: Evaluate the specific debugging needs of your application. If you frequently encounter issues related to hooks, custom debug information can significantly improve your debugging workflow.
Ultimately, the decision to use custom debug information depends on the specific requirements and challenges of your project.
-
Future Developments and Updates
7.1 ReactJS Roadmap for useDebugValue
As of now, ReactJS does not have any specific updates or enhancements planned for useDebugValue. However, React’s development team regularly releases updates and improvements to the library, so it’s possible that future versions may include enhancements related to debugging and useDebugValue.
Stay up-to-date with ReactJS releases and documentation to keep track of any future developments or improvements to useDebugValue.
7.2 Community Feedback and Contributions
The ReactJS community plays a vital role in shaping the future of the library and its features. If you have feedback, suggestions, or ideas for improving useDebugValue or any other aspect of React, consider contributing to the React repository on GitHub or participating in discussions on React’s official channels, such as the ReactJS Community Forum or React’s GitHub Discussions.
By actively engaging with the ReactJS community, you can help drive the evolution of debugging tools and contribute to making React even more powerful and developer-friendly.
7.3 Potential Enhancements and Extensions for Custom Debug Information
While useDebugValue already provides a flexible way to customize debug information for hooks, there are potential enhancements and extensions that could further improve the debugging experience. Some ideas for potential enhancements include:
7.3 Potential Enhancements and Extensions for Custom Debug Information
While useDebugValue already provides a flexible way to customize debug information for hooks, there are potential enhancements and extensions that could further improve the debugging experience. Some ideas for potential enhancements include:
Integration with external debugging tools or logging libraries: Allowing useDebugValue to integrate with popular debugging tools or logging libraries could provide developers with even more options for monitoring and debugging their hooks.
Support for additional debugging information types: While useDebugValue currently supports various data types, expanding its capabilities to support more complex data structures or custom formatters could enhance the customization options for debug information.
Standardized debugging patterns or conventions: Establishing best practices and patterns for using useDebugValue and custom debug information could help streamline debugging workflows and make them more consistent across projects.
These are just a few ideas, and the future of useDebugValue will largely depend on the needs and feedback of the ReactJS community.
-
Conclusion
In this blog post, we explored the power of the useDebugValue hook in ReactJS for providing custom debug information for hooks. We discussed the purpose and benefits of useDebugValue, its usage syntax and parameters, and how it works internally. By leveraging useDebugValue, developers can enhance their debugging experience by attaching meaningful debug information to their hooks.
We learned about creating custom debug values and formatting them appropriately, as well as updating debug values dynamically. Additionally, we discussed performance considerations, troubleshooting common issues, and provided tips for effective debugging with useDebugValue.
Furthermore, we compared useDebugValue with other debugging techniques available in ReactJS, such as console logging and React DevTools. We also explored the potential for future developments and updates to useDebugValue, highlighting the importance of community feedback and contributions in shaping the future of React.
To further support your learning journey, we provided resources and references, including the official ReactJS documentation on useDebugValue, tutorials and guides, related articles and blog posts, and additional references for React hooks and debugging.
In conclusion, useDebugValue is a valuable tool for customizing and displaying debug information for hooks in ReactJS. By utilizing useDebugValue effectively, developers can gain insights into the behavior of their hooks, enhance their debugging workflow, and build more maintainable and efficient React applications.
Table of Contents
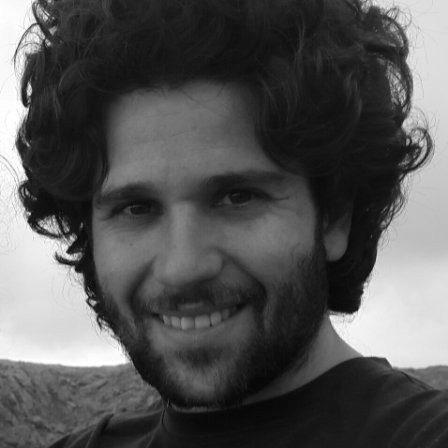
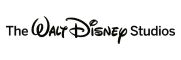