Reacting to Motion: Building Engaging Experiences with useDeviceMotion in ReactJS
We live in an era of technology where user interaction has transcended beyond simple clicks and keyboard inputs. In the modern web development sphere, motion-based interactions are playing a crucial role in providing an immersive user experience. These interactions can include anything from scrolling, hovering to more advanced gyroscope-based motions.
In the realm of ReactJS, the device motion and orientation events enable access to the device’s physical movement in space. This feature enables developers, especially those you hire as ReactJS developers, to create unique and interactive experiences for users. Having skilled ReactJS developers on board ensures these advanced techniques are optimally utilized to enhance user experience.
In this post, we are going to look at how to leverage `useDeviceMotion` in ReactJS to build motion-based interactions. This guide provides an excellent way for both seasoned and newly hired ReactJS developers to grasp the concept with concrete examples.
Introduction to `useDeviceMotion`
`useDeviceMotion` is a hook in ReactJS that allows you to use the DeviceMotionEvent API. The `DeviceMotionEvent` provides web developers with information about the speed of changes for the device’s physical movement around the three axes (X, Y, and Z). It captures the acceleration, rotation rate, and the interval of time for checking these properties.
To get the most from this blog post, it’s essential to have a fundamental understanding of ReactJS. Familiarity with React hooks, in particular, would be beneficial.
Using `useDeviceMotion`
Here is the basic usage of `useDeviceMotion`:
```jsx import { useDeviceMotion } from 'react-use'; function Component() { const state = useDeviceMotion(); return <pre>{JSON.stringify(state, null, 2)}</pre>; } ```
In this code, we are importing and using the `useDeviceMotion` hook from ‘react-use’. The `state` object will hold the values related to the device motion.
Building Motion-Based Interactions
Let’s take a look at how we can use `useDeviceMotion` to build different types of motion-based interactions in ReactJS.
Example 1: Interactive Gallery
In this example, we’ll build an interactive image gallery where the images move based on the device’s orientation. It creates a sense of depth in the web page, providing a 3D-like experience.
```jsx import React from 'react'; import { useDeviceMotion } from 'react-use'; function Gallery() { const { accelerationIncludingGravity } = useDeviceMotion(); return ( <div> {IMAGES_ARRAY.map((image, index) => ( <img key={index} src={image.src} alt={image.alt} style={{ transform: `translate3d(${accelerationIncludingGravity.x}px, ${accelerationIncludingGravity.y}px, 0)` }} /> ))} </div> ); } ```
In this snippet, we are getting the `accelerationIncludingGravity` values from `useDeviceMotion` hook. We then use these values to translate the images based on the device’s motion.
Example 2: Parallax Scrolling
Parallax scrolling is a popular web design effect where the background content moves at a different speed than the foreground content as you scroll. This can be enhanced with device motion, where the background content moves with the device’s orientation, creating an immersive 3D effect.
```jsx import React from 'react'; import { useDeviceMotion } from 'react-use'; function ParallaxScroll() { const { rotationRate } = useDeviceMotion(); return ( <div className="parallax"> <div className="background" style={{ transform: `translate3d(${rotationRate.beta}px, ${rotationRate.gamma}px, 0)` }} > {/* Background Content */} </div> <div className="foreground"> {/* Foreground Content */} </div> </div> ); } ```
In this code, we’re obtaining the `rotationRate` values from the `useDeviceMotion` hook and using these to move the background content.
Example 3: Motion-Based Games
In this example, we’ll use `useDeviceMotion` to control the movement of a character in a game.
```jsx import React from 'react'; import { useDeviceMotion } from 'react-use'; function Game() { const { acceleration } = useDeviceMotion(); return ( <div className="game-area"> <div className="character" style={{ transform: `translate3d(${acceleration.x * 10}px, ${acceleration.y * 10}px, 0)` }} > {/* Character */} </div> </div> ); } ```
Here, the character’s movement is dictated by the device’s acceleration. The `acceleration` values are obtained from `useDeviceMotion` and multiplied by 10 to create a more noticeable effect.
Best Practices and Things to Remember
- Performance: While motion interactions can significantly enhance the user experience, overusing them can negatively impact performance, especially on low-end devices. Always test your applications on various devices to ensure a smooth experience.
- Accessibility: Not all users will have devices capable of detecting motion, or some may have disabled motion at the OS level for accessibility reasons. It’s essential to ensure your app remains functional and accessible without motion interactions.
- Fallbacks: Always provide a fallback for when the DeviceMotionEvent is not supported or if the user has opted out of motion-based interactions.
Conclusion
ReactJS’s `useDeviceMotion` hook provides an easy way to leverage device motion and orientation events in your application. This is why hiring experienced ReactJS developers can be an invaluable decision for your project. They can use such advanced hooks to create a wide range of engaging and immersive experiences for your users.
Remember, however, that while these features can be exciting, they should enhance the user experience, not detract from it. Hence, it’s crucial to have ReactJS developers who keep your users’ needs in mind while innovatively utilizing such powerful features. This approach will ensure that you are on the right path to creating fantastic, interactive experiences with ReactJS and device motion.
We hope you found this guide insightful and it inspires you to explore more about what you can achieve with professional ReactJS developers.
Table of Contents
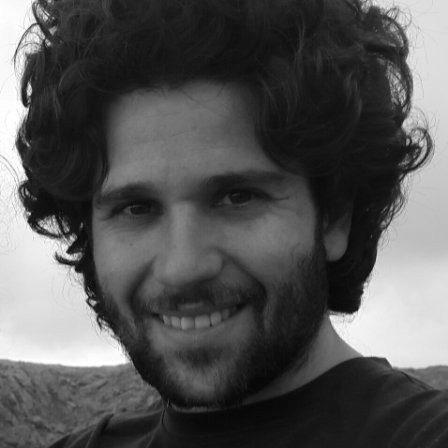
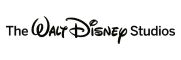