Unleashing Mobile Interactivity with ReactJS: Using the useDeviceOrientation Hook
ReactJS, the popular JavaScript library known for creating interactive user interfaces, is rich with tools, features, and libraries that developers can leverage to augment their web applications. This diversity of tools is one of the many reasons businesses opt to hire ReactJS developers for enhancing their web solutions. A notable feature among these tools is device orientation data – an invaluable resource for crafting immersive and responsive applications.
In this blog post, we’ll explore how to use `useDeviceOrientation` in ReactJS components, a task well-suited for those who hire ReactJS developers to make the most out of their web apps. This tool can be a key player in crafting a more interactive and responsive user experience, especially in applications intended for mobile use. By leveraging the power of device orientation data, businesses who hire ReactJS developers can ensure they’re offering cutting-edge, dynamic user interfaces in their applications.
What is Device Orientation?
Before we jump into the `useDeviceOrientation` hook in React, let’s quickly understand what device orientation is. Simply put, device orientation refers to the physical orientation of a device in terms of its rotation and tilt.
Modern devices, including smartphones and tablets, come equipped with built-in sensors that can track the device’s orientation in three-dimensional space. This data typically includes the following three components:
– Alpha: The device’s rotation around the z-axis. The z-axis runs from the front of the device to the back.
– Beta: The device’s rotation around the x-axis. The x-axis runs from the left side of the device to the right side.
– Gamma: The device’s rotation around the y-axis. The y-axis runs from the bottom of the device to the top.
This image showing the three axes of rotation (alpha, beta, gamma) in device orientation (https://developer.mozilla.org/en-US/docs/Web/API/Detecting_device_orientation)
The Importance of Accessing Device Orientation Data
The ability to access and manipulate a device’s orientation data can significantly enhance the user experience. For example, game developers can utilize orientation data to create immersive experiences where the user can control gameplay by tilting their device. Similarly, virtual reality applications can use this data to adjust the view based on the user’s orientation, creating a more realistic and immersive experience.
Now, let’s see how we can access this valuable data in our React components using the `useDeviceOrientation` hook.
The useDeviceOrientation Hook
React, in its philosophy of declarative programming, uses hooks to allow you to “hook into” React features from your function components. The `useDeviceOrientation` hook is a custom hook that gives us access to the device orientation data in our components.
While React does not provide this hook out of the box, we can easily create it using the native `window.DeviceOrientationEvent` web API.
Let’s see how we can create this hook.
```javascript import { useState, useEffect } from "react"; function useDeviceOrientation() { const [orientation, setOrientation] = useState({ alpha: null, beta: null, gamma: null, }); useEffect(() => { const handleOrientation = (event) => { setOrientation({ alpha: event.alpha, beta: event.beta, gamma: event.gamma, }); }; window.addEventListener("deviceorientation", handleOrientation); return () => { window.removeEventListener("deviceorientation", handleOrientation); }; }, []); return orientation; } ```
In the code above, we define a custom React hook called `useDeviceOrientation`. We start by initializing the state with an object representing the alpha, beta, and gamma values. Then we use the `useEffect` hook to set up an event listener for the `deviceorientation` event. When the device’s orientation changes, our handler function updates the state with the new orientation data.
We then return the orientation data from our hook, which can be used by any component that calls `useDeviceOrientation`.
Using the useDeviceOrientation Hook in Components
Let’s see how we can use the `useDeviceOrientation` hook in a component. In this example, we’ll create a component that displays the current orientation data on the screen.
```javascript import React from 'react'; import useDeviceOrientation from './useDeviceOrientation'; function DeviceOrientationDisplay() { const orientation = useDeviceOrientation(); return ( <div> <h1>Device Orientation:</h1> <p>Alpha: {orientation.alpha}</p> <p>Beta: {orientation.beta}</p> <p>Gamma: {orientation.gamma}</p> </div> ); } export default DeviceOrientationDisplay; ```
Here, we import our `useDeviceOrientation` hook and use it in our `DeviceOrientationDisplay` component. The component displays the current alpha, beta, and gamma values on the screen.
Handling Unsupported Devices
Not all devices support the `deviceorientation` event. In such cases, it’s good practice to handle this gracefully in our hook. Let’s modify our `useDeviceOrientation` hook to handle devices that don’t support device orientation:
```javascript import { useState, useEffect } from "react"; function useDeviceOrientation() { const [orientation, setOrientation] = useState({ alpha: null, beta: null, gamma: null, unsupported: false, }); useEffect(() => { if (!window.DeviceOrientationEvent) { setOrientation((prevOrientation) => ({...prevOrientation, unsupported: true})); return; } const handleOrientation = (event) => { setOrientation({ alpha: event.alpha, beta: event.beta, gamma: event.gamma, unsupported: false, }); }; window.addEventListener("deviceorientation", handleOrientation); return () => { window.removeEventListener("deviceorientation", handleOrientation); }; }, []); return orientation; } ```
We’ve added an `unsupported` field to our state object and checked whether `window.DeviceOrientationEvent` exists before setting up the event listener. If it doesn’t exist, we update the state to mark the device as unsupported.
Conclusion
React’s flexibility and the capabilities of its native APIs make it straightforward to create immersive, interactive experiences in your applications. This ease of use is one reason why many businesses choose to hire ReactJS developers for their projects. Utilizing a custom hook like `useDeviceOrientation`, developers can tap into the power of device orientation data, creating a richer, more dynamic user interface.
Whether you’re building a game, a virtual reality experience, or you just want to add a unique interaction to your application, the `useDeviceOrientation` hook offers a simple method for accessing and using device orientation data. It’s an example of the power you can leverage when you hire ReactJS developers.
Remember, not all devices support this functionality. As responsible developers, it’s essential that we ensure our applications can gracefully handle such instances where this feature is not supported.
So, whether you’re a developer, or you’re looking to hire ReactJS developers, consider the possibilities that device orientation can bring to your React applications! Explore, experiment, and create immersive experiences!
Table of Contents
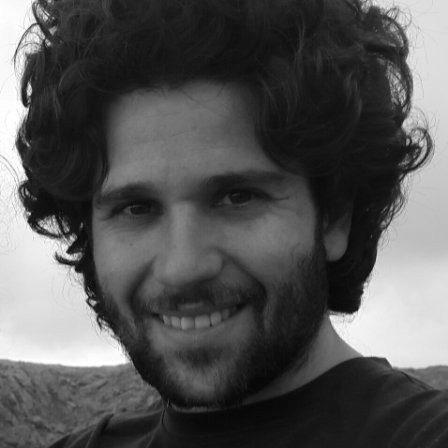
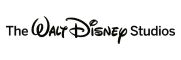