Efficient Resource Management in ReactJS using Document Visibility Detection
In modern web application development, user interactions and the state of the user interface play a pivotal role in offering an engaging and dynamic user experience. At times, it’s not merely about interacting with elements on the page, but also comprehending and detecting whether a user is currently viewing the page or document. This is where the expertise of ReactJS developers can make a significant difference.
Such understanding is crucial for optimizing resources or managing specific events when the user is not active on your page. Thus, when you hire ReactJS developers with such comprehension, it can substantially benefit your application’s performance.
In this blog post, we will explore the `document.visibilityState` property and demonstrate how to create a custom React Hook, `useDocumentVisibility`. This hook, often employed by skilled ReactJS developers, enables easy detection and handling of visibility changes in a React application. This can be incredibly useful for pausing video playback, halting API calls, or curtailing other resource-intensive tasks when a user is not actively engaged with your webpage.
What is the Document Visibility API?
The Page Visibility API is a web standard that provides events you can watch for to know when a document becomes visible or hidden. It also includes the `document.visibilityState` property to check if the page is in the foreground or background.
The `document.visibilityState` property can return one of the following:
– ‘visible’: The page content may be at least partially visible. In practice, this means the page is the foreground tab of a non-minimized window.
– ‘hidden’: The page content is not visible to the user. In practice, this means the document is either a background tab or part of a minimized window, or the OS screen lock is active.
– ‘prerender’: The page is currently being loaded off-screen and has not yet been displayed on screen.
This is an essential API to optimize resource usage and improve the user experience based on whether the user is actively viewing your page or not.
Creating the useDocumentVisibility Hook
Let’s create a custom Hook in React that will provide the current visibility status of the document.
Here’s a basic implementation:
```jsx import { useState, useEffect } from 'react'; function getVisibilityState() { return document.visibilityState; } function useDocumentVisibility() { const [visibility, setVisibility] = useState(getVisibilityState); const handleVisibilityChange = () => { setVisibility(getVisibilityState); }; useEffect(() => { window.addEventListener('visibilitychange', handleVisibilityChange); return () => { window.removeEventListener('visibilitychange', handleVisibilityChange); }; }, []); return visibility; } ```
In the code above, we initially set the visibility state to the current visibility status of the document. We then listen for the `‘visibilitychange’` event and update our state when it fires. Finally, we clean up by removing the event listener when the component unmounts.
Using the useDocumentVisibility Hook
We can now use our `useDocumentVisibility` Hook in our components to adapt their behavior based on the visibility of the document. Here’s a basic example:
```jsx import React from 'react'; import useDocumentVisibility from './useDocumentVisibility'; function VideoPlayer({ src }) { const visibility = useDocumentVisibility(); const videoRef = React.useRef(); React.useEffect(() => { if (visibility === 'hidden' && videoRef.current) { videoRef.current.pause(); } else if (visibility === 'visible' && videoRef.current) { videoRef.current.play(); } }, [visibility]); return <video ref={videoRef} src={src} autoPlay />; } export default VideoPlayer; ```
In the example above, we have a `VideoPlayer` component. It uses the `useDocumentVisibility` Hook to pause video playback when the user is not currently viewing the document and resumes playback when the user comes back.
A More Complex Example: Fetching Data
We can also use the `useDocumentVisibility` Hook to optimize API calls. Suppose you have a component that regularly polls an API to fetch data. If the user is not currently viewing the document, there is no need to continue polling, so we can stop it. When the user comes back, we can resume polling.
Here’s an example of how you can do that:
```jsx import React from 'react'; import useDocumentVisibility from './useDocumentVisibility'; import useInterval from './useInterval'; function DataFetcher({ api }) { const visibility = useDocumentVisibility(); const [data, setData] = React.useState(null); const fetchData = async () => { const response = await fetch(api); const data = await response.json(); setData(data); }; useInterval(fetchData, visibility === 'visible' ? 5000 : null); return ( <div> {data ? ( <pre>{JSON.stringify(data, null, 2)}</pre> ) : ( 'Loading data...' )} </div> ); } export default DataFetcher; ```
In this component, we use the `useInterval` Hook to fetch data from an API every 5 seconds. The `useInterval` Hook runs a function at specified intervals, and we stop it by passing `null`. We only run the interval when the visibility is `’visible’`, effectively stopping and resuming the interval based on the visibility of the document.
Conclusion
The Page Visibility API is a powerful tool for optimizing resources and adapting your application based on whether the user is actively viewing your document. With the skills of proficient ReactJS developers, it becomes even more straightforward to leverage this API in your components using the `useDocumentVisibility` Hook.
You can adapt video playback, API calls, animations, or any other resource-intensive tasks based on the document visibility, thereby improving user experience and optimizing resource usage. Hiring ReactJS developers who understand these concepts can be a game-changer in developing efficient applications.
Remember, an application that respects user interaction and application state is one that keeps users engaged and delivers a fantastic user experience. This is why it’s crucial to hire ReactJS developers who consider aspects like document visibility when building modern and intuitive applications.
Table of Contents
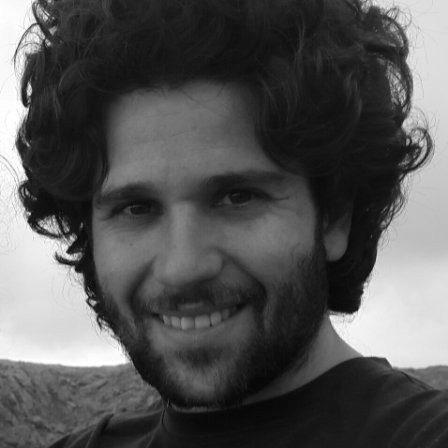
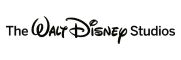