Designing Drag-and-Drop Functionality in ReactJS using Custom Hooks
ReactJS, a popular JavaScript library for building user interfaces, provides developers with a suite of hooks to manage various aspects of the component lifecycle. It’s the tool of choice for many organizations looking to hire ReactJS developers due to its flexibility and efficiency. However, creating a drag-and-drop functionality, a common requirement in modern web applications, isn’t directly addressed within the suite of hooks.
In this article, we’ll explore building a custom ReactJS hook, `useDraggable`, a task often handled by experienced ReactJS developers, to address drag-and-drop behavior. We’ll break down the steps in a beginner-friendly manner and provide concrete examples to guide your understanding. These examples will also serve as an insight into the work of ReactJS developers, demonstrating the complexities they navigate when developing interactive user interfaces.
What are Hooks?
Before we dive in, let’s take a step back and explain what React Hooks are. Introduced in React 16.8, hooks allow developers to use state and other React features without writing a class. They let you use state and lifecycle methods inside functional components, among other things.
A custom hook is a JavaScript function whose name starts with “use”, and it can call other hooks. Custom hooks are a mechanism to reuse stateful logic between different components.
The Problem
Let’s suppose you’re building a Trello-like task management application. You want to allow users to drag and drop tasks between different columns (e.g., Todo, Doing, Done). This is where our `useDraggable` hook will come into play.
Building the `useDraggable` Hook
Let’s start building our custom hook. This hook will keep track of the dragging state and update the position of the dragged item in the DOM.
```javascript import { useState, useEffect } from 'react'; const useDraggable = (id) => { const [dragging, setDragging] = useState(false); useEffect(() => { const handleDragStart = (event) => { event.dataTransfer.setData('text/plain', id); setDragging(true); }; const handleDragEnd = () => { setDragging(false); }; const dragSource = document.querySelector(`#${id}`); dragSource.addEventListener('dragstart', handleDragStart); dragSource.addEventListener('dragend', handleDragEnd); return () => { dragSource.removeEventListener('dragstart', handleDragStart); dragSource.removeEventListener('dragend', handleDragEnd); }; }, [id]); return { dragging }; }; export default useDraggable; ```
In this code, `useDraggable` is a hook that takes an `id` as a parameter, which is the id of the DOM element you want to make draggable. It uses the `useState` hook to manage the dragging state, and the `useEffect` hook to set up event listeners for the `dragstart` and `dragend` events. It uses `setData` to specify the data that will be available to the drop target. `setDragging(true)` is used when dragging starts and `setDragging(false)` when dragging ends.
Using the `useDraggable` Hook
You can use this hook in a component that represents a draggable item. Here’s an example:
```javascript import React from 'react'; import useDraggable from './hooks/useDraggable'; const DraggableItem = ({ id, children }) => { const { dragging } = useDraggable(id); return ( <div id={id} draggable={true} style={{ opacity: dragging ? 0.5 : 1 }}> {children} </div> ); }; export default DraggableItem; ```
Here, the `DraggableItem` component takes an `id` and `children` as props. It uses the `useDraggable` hook, passing the `id` as an argument. The `dragging` state from the `useDraggable` hook is used to adjust the opacity of the item while it’s being dragged.
Building a `useDroppable` Hook
To complement our `useDraggable` hook, we’ll need a `useDroppable` hook to handle drop events.
```javascript import { useState, useEffect } from 'react'; const useDroppable = (id) => { const [over, setOver] = useState(false); useEffect(() => { const handleDragOver = (event) => { event.preventDefault(); setOver(true); }; const handleDragLeave = () => { setOver(false); }; const handleDrop = (event) => { event.preventDefault(); const draggedId = event.dataTransfer.getData('text/plain'); // handle the drop action here setOver(false); }; const dropTarget = document.querySelector(`#${id}`); dropTarget.addEventListener('dragover', handleDragOver); dropTarget.addEventListener('dragleave', handleDragLeave); dropTarget.addEventListener('drop', handleDrop); return () => { dropTarget.removeEventListener('dragover', handleDragOver); dropTarget.removeEventListener('dragleave', handleDragLeave); dropTarget.removeEventListener('drop', handleDrop); }; }, [id]); return { over }; }; export default useDroppable; ```
In this code, the `useDroppable` hook works similarly to `useDraggable`, but it sets up listeners for `dragover`, `dragleave`, and `drop` events instead. It manages an `over` state that’s true when a draggable item is currently over the drop target and false otherwise. In the `handleDrop` function, we retrieve the `id` of the dragged item using `getData`.
Conclusion
In this blog post, we’ve explored how to create a custom hook in ReactJS for managing drag-and-drop functionality. This is a technique commonly used by skilled ReactJS developers. We’ve learned how to create the `useDraggable` and `useDroppable` hooks, and how to effectively use them in components. The power of these hooks lies in their ability to encapsulate the logic related to drag-and-drop, thus promoting code reusability and maintainability.
The examples provided are foundational, and you might need to hire ReactJS developers to enhance them based on your specific requirements. However, they offer a solid understanding of the underlying concepts of working with the drag-and-drop API in conjunction with React hooks, a crucial skill in the repertoire of proficient ReactJS developers.
Table of Contents
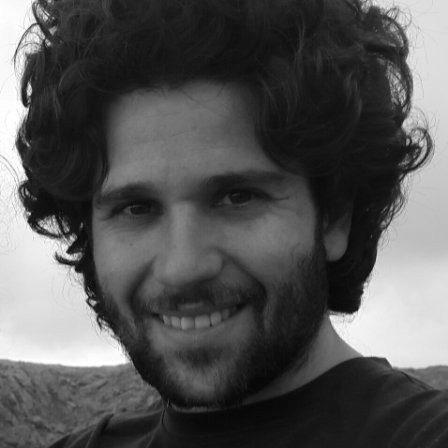
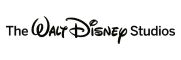