Exploring ReactJS Hooks: Understanding the Distinctions between useEffect and useLayoutEffect
ReactJS is a popular JavaScript library used for building user interfaces. It provides developers with powerful tools and hooks to manage state, handle side effects, and optimize the rendering process. Two commonly used hooks in ReactJS are useEffect and useLayoutEffect. While they may seem similar at first glance, there are important differences between the two. In this blog post, we will explore the disparities between useEffect and useLayoutEffect and understand when to use each of them in your React applications.
-
Introduction to useEffect and useLayoutEffect
Before we dive into the differences, let’s briefly explain what useEffect and useLayoutEffect are and what they do.
1.1 useEffect
useEffect is a hook provided by React that allows you to perform side effects in functional components. Side effects can include things like data fetching, subscriptions, or manually changing the DOM.
The useEffect hook takes two arguments: a callback function and a dependencies array. The callback function contains the side effect logic, while the dependencies array specifies which values the effect depends on. When one or more of the dependencies change, the effect will be re-executed.
Here’s an example of using useEffect to fetch data from an API when a component mounts:
import React, { useEffect, useState } from 'react'; const MyComponent = () => { const [data, setData] = useState([]); useEffect(() => { const fetchData = async () => { const response = await fetch('https://api.example.com/data'); const jsonData = await response.json(); setData(jsonData); }; fetchData(); }, []); // empty dependencies array to fetch data only once return ( // render component with fetched data ); };
1.2 useLayoutEffect
useLayoutEffect is similar to useEffect, but it has a different timing during the component lifecycle. While useEffect runs after the browser has painted and the component has rendered, useLayoutEffect runs synchronously after all DOM mutations but before the browser paints the screen.
The useLayoutEffect hook is useful when you need to perform an action that relies on the DOM layout being updated. For example, you might want to measure the dimensions of an element or update a CSS property based on the rendered DOM.
The usage of useLayoutEffect is almost identical to useEffect, with the same callback function and dependencies array. Let’s see an example:
import React, { useLayoutEffect, useState } from 'react'; const MyComponent = () => { const [elementWidth, setElementWidth] = useState(0); useLayoutEffect(() => { const handleResize = () => { const width = document.getElementById('myElement').clientWidth; setElementWidth(width); }; window.addEventListener('resize', handleResize); handleResize(); return () => { window.removeEventListener('resize', handleResize); }; }, []); // empty dependencies array to add and remove event listeners once return ( <div id="myElement" style={{ width: '100%' }}> Element width: {elementWidth}px </div> ); };
In this example, we use useLayoutEffect to measure the width of an element and update the state whenever the window is resized. The effect runs synchronously after the DOM layout changes, ensuring we get the correct width before the screen paints.
1.3 Differences between useEffect and useLayoutEffect
Now that we have a basic understanding of both useEffect and useLayoutEffect, let’s explore the key differences between them.
1.4 Timing and Performance
As mentioned earlier, the primary difference between useEffect and useLayoutEffect lies in their timing and performance characteristics.
useEffect is scheduled to run after the component renders and the browser has painted. This means that it doesn’t block the browser from updating the screen. It’s considered an asynchronous operation, making it more suitable for handling non-critical side effects, such as data fetching or logging. However, since it runs after the paint, the user might experience a slight delay in seeing the updated content.
On the other hand, useLayoutEffect runs synchronously immediately after all DOM mutations but before the browser paints the screen. This means that it can potentially block the browser from updating the UI, leading to a slower rendering process if the logic inside useLayoutEffect is time-consuming. It’s crucial to be mindful of the performance implications when using useLayoutEffect.
In most cases, using useEffect is sufficient for managing side effects in your React components. However, if you need to interact directly with the DOM or perform layout-related calculations, useLayoutEffect might be more appropriate to ensure accurate measurements and prevent layout thrashing.
-
Server-Side Rendering (SSR) Considerations
Another important distinction between useEffect and useLayoutEffect relates to server-side rendering (SSR) and how the hooks behave in that context.
During SSR, React renders components on the server before sending them to the client. The useEffect and useLayoutEffect hooks can both be executed on the server side, but their timing differs.
useEffect doesn’t block the rendering process and is deferred until the browser takes over. This behavior makes it suitable for SSR, as it won’t affect the server’s rendering performance.
However, useLayoutEffect can potentially impact the server’s rendering process because it runs synchronously. If your code relies on the DOM or browser-specific features within useLayoutEffect, it may lead to errors during SSR. In such cases, it’s recommended to conditionally skip or replace useLayoutEffect with useEffect when running on the server to ensure a smooth SSR experience.
To conditionally skip the execution of useLayoutEffect on the server, you can use the useIsomorphicLayoutEffect hook provided by libraries like react-use or use-isomorphic-layout-effect.
2.1 Recommendation for Choosing between useEffect and useLayoutEffect
To summarize, here are some recommendations for choosing between useEffect and useLayoutEffect in your React applications:
Use useEffect for most cases of managing side effects in your components. It provides a good balance between performance and flexibility.
Consider using useLayoutEffect when you need to perform actions that rely on the updated DOM layout, such as measuring elements or updating CSS properties based on the rendered content.
Be cautious when using useLayoutEffect due to its synchronous nature, as it can potentially impact performance. Make sure the logic inside useLayoutEffect is optimized and doesn’t cause delays in rendering.
Remember to consider SSR implications. useEffect is generally safe for SSR, while useLayoutEffect should be used with caution and possibly conditionally skipped during server-side rendering.
If you’re unsure whether to use useEffect or useLayoutEffect, start with useEffect and switch to useLayoutEffect only when you encounter specific layout-related requirements.
By understanding the differences between useEffect and useLayoutEffect and considering their characteristics, you can make informed decisions when choosing the appropriate hook for managing side effects and layout-related actions in your React components.
Both useEffect and useLayoutEffect are powerful tools in ReactJS, with subtle differences that make them suitable for different scenarios. While useEffect is asynchronous and runs after the component renders, useLayoutEffect is synchronous and runs before the browser paints the screen. useEffect is commonly used for general side effects, such as data fetching and subscriptions, while useLayoutEffect is useful for tasks that require the updated DOM layout, such as measuring elements or updating CSS properties.
When it comes to performance, useEffect is generally more performant as it doesn’t block the rendering process. However, useLayoutEffect can potentially impact performance, especially if the logic inside it is time-consuming. Therefore, it’s crucial to optimize the code inside useLayoutEffect and be mindful of performance implications.
When considering server-side rendering (SSR), useEffect is safe to use as it doesn’t block rendering. On the other hand, useLayoutEffect can cause issues during SSR and should be conditionally skipped or replaced with useEffect when running on the server.
2.2 Timing Differences
One important distinction is the timing at which useEffect and useLayoutEffect are executed. Here’s an example to illustrate this:
import React, { useEffect, useLayoutEffect } from 'react'; const MyComponent = () => { useEffect(() => { console.log('useEffect'); }); useLayoutEffect(() => { console.log('useLayoutEffect'); }); console.log('Component rendering'); return ( // JSX to render the component ); };
In this example, when the component renders, the console output will be as follows:
Component rendering useEffect useLayoutEffect
As you can see, useEffect runs after the component renders, while useLayoutEffect runs before the component is painted on the screen.
-
DOM Manipulation and Layout Calculations
useLayoutEffect is especially useful when you need to perform actions that rely on the updated DOM layout. Let’s take a look at an example where we measure the height of an element using useLayoutEffect:
import React, { useLayoutEffect, useRef } from 'react'; const MyComponent = () => { const elementRef = useRef(null); let elementHeight = 0; useLayoutEffect(() => { elementHeight = elementRef.current.clientHeight; }); return ( <div ref={elementRef}> <p>Element Height: {elementHeight}px</p> </div> ); };
In this example, we use the useRef hook to create a reference to the element we want to measure. Inside the useLayoutEffect hook, we access the clientHeight property of the element to retrieve its height. This ensures that we get the accurate height after the DOM layout is updated but before the screen is painted.
3.1 SSR Considerations
As mentioned earlier, it’s important to consider server-side rendering (SSR) when using useEffect and useLayoutEffect. Here’s an example demonstrating how to conditionally skip useLayoutEffect during SSR:
import React, { useLayoutEffect, useEffect, useState } from 'react'; const MyComponent = () => { const [data, setData] = useState([]); useLayoutEffect(() => { // DOM-related operations // This code won't be executed during SSR }, []); useEffect(() => { // Side effect code // This code will be executed during SSR }, []); return ( // JSX to render the component ); };
In this example, we use both useEffect and useLayoutEffect. However, since useLayoutEffect may cause issues during SSR, we can rely on useEffect to handle the server-side rendering case. The code inside useEffect will execute on the server and in the browser, while the code inside useLayoutEffect will only execute in the browser.
4. To summarize, here are some key points to remember
Use useEffect for most cases of managing side effects in your components, as it provides a good balance of performance and flexibility.
Reserve useLayoutEffect for tasks that require the updated DOM layout or interactions with the browser-specific features.
Optimize the code inside useLayoutEffect to ensure it doesn’t cause delays in rendering.
Consider SSR implications and conditionally skip or replace useLayoutEffect when running on the server.
Start with useEffect and switch to useLayoutEffect only when specific layout-related requirements arise.
By understanding the differences and appropriate use cases for useEffect and useLayoutEffect, you can leverage these hooks effectively to manage side effects and handle layout-related tasks in your React applications.
-
Conclusion
Understanding the differences between useEffect and useLayoutEffect is crucial for effectively managing side effects and layout-related tasks in React applications. useEffect is asynchronous and runs after the component renders, making it suitable for most side effects. useLayoutEffect is synchronous and runs before the component is painted, making it useful for DOM manipulation and layout calculations.
By utilizing these hooks appropriately, you can ensure optimal performance and handle specific requirements in your React components.
Table of Contents
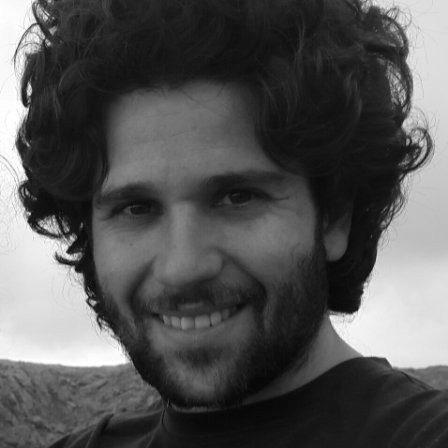
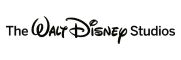