ReactJS useEventListener: Simplifying DOM Event Handling in Functional Components
In the world of web development, ReactJS has gained significant popularity due to its efficient and flexible approach to building user interfaces. One of the key advantages of ReactJS is its ability to handle events seamlessly. While event handling is straightforward in class components, functional components require a different approach. In this blog post, we will explore the use of the useEventListener hook in ReactJS to handle DOM events in functional components.
Overview of ReactJS and Functional Components
ReactJS is a JavaScript library that allows developers to build reusable UI components. It follows a component-based architecture, where each component manages its state and renders a view based on that state. Functional components are a simpler and more concise way of writing React components compared to class components. They are pure functions that take props as input and return JSX (JavaScript XML) as output.
What is useEventListener?
The useEventListener hook is a custom hook that enables functional components to attach event listeners to DOM elements. It abstracts away the complexities of event handling and provides a clean and reusable solution. With useEventListener, you can easily listen for various events, such as clicks, keypresses, scrolls, and more.
Understanding the Concept of useEventListener
Before diving into the implementation details, let’s understand the concept behind the useEventListener hook. In React, the useEffect hook is commonly used to manage side effects, such as event listeners. By combining the useEffect hook with the power of closures, we can create a custom hook that adds event listeners when the component mounts and removes them when the component unmounts.
Here’s an example implementation of the useEventListener hook:
import { useEffect } from 'react'; const useEventListener = (eventName, handler, element = window) => { useEffect(() => { const eventListener = (event) => handler(event); element.addEventListener(eventName, eventListener); return () => { element.removeEventListener(eventName, eventListener); }; }, [eventName, handler, element]); }; export default useEventListener;
Benefits of Using useEventListener in ReactJS
Simplified event handling: By encapsulating event listener logic in a reusable hook, you can declutter your functional components and make event handling more manageable.
Consistent event management: useEventListener ensures that event listeners are added and removed correctly, preventing memory leaks and potential bugs.
Improved reusability: Since useEventListener is a custom hook, you can easily share it across different components and projects, reducing code duplication.
How to use useEventListener in ReactJS?
Using the useEventListener hook is straightforward. Let’s say we want to listen for a ‘click’ event on a button element:
import React from 'react'; import useEventListener from './useEventListener'; const MyComponent = () => { const handleClick = () => { console.log('Button clicked!'); }; useEventListener('click', handleClick); return <button>Click me</button>; }; export default MyComponent;
In the example above, we define a handleClick function that logs a message when the button is clicked. We then use the useEventListener hook to attach the ‘click’ event listener to the button element. Whenever the button is clicked, the handleClick function will be called.
Examples of useEventListener in ReactJS
Let’s explore a few more examples to understand the versatility of the useEventListener hook.
Example 1: Listening for keypress events
import React from 'react'; import useEventListener from './useEventListener'; const MyComponent = () => { const handleKeyPress = (event) => { console.log('Key pressed:', event.key); }; useEventListener('keypress', handleKeyPress); return <div>Press any key</div>; }; export default MyComponent;
In the above example, we listen for ‘keypress’ events and log the pressed key to the console.
Example 2: Listening for scroll events
import React, { useState } from 'react'; import useEventListener from './useEventListener'; const MyComponent = () => { const [scrollPosition, setScrollPosition] = useState(0); const handleScroll = () => { setScrollPosition(window.pageYOffset); }; useEventListener('scroll', handleScroll); return <div>Scroll position: {scrollPosition}px</div>; }; export default MyComponent;
In this example, we track the scroll position of the window and update it whenever a scroll event occurs.
Let’s explore a few more examples to understand the versatility of the useEventListener hook.
Example 3: Handling a custom event
import React from 'react'; import useEventListener from './useEventListener'; const MyComponent = () => { const handleCustomEvent = (event) => { console.log('Custom event triggered:', event.detail); }; useEventListener('customEvent', handleCustomEvent); return ( <div> <button onClick={() => { const customEvent = new CustomEvent('customEvent', { detail: 'Custom event data', }); window.dispatchEvent(customEvent); }}>Trigger Custom Event</button> </div> ); }; export default MyComponent;
In this example, we define a handleCustomEvent function that logs the custom event details when the event is triggered. We then use the useEventListener hook to listen for the ‘customEvent’ event. Inside the component, we have a button that, when clicked, dispatches the custom event with some custom data. The handleCustomEvent function is called when the custom event is triggered, and it logs the event details to the console.
Example 4: Listening for window resize events
import React, { useState } from 'react'; import useEventListener from './useEventListener'; const MyComponent = () => { const [windowSize, setWindowSize] = useState({ width: window.innerWidth, height: window.innerHeight, }); const handleResize = () => { setWindowSize({ width: window.innerWidth, height: window.innerHeight, }); }; useEventListener('resize', handleResize, window); return ( <div> <div>Window Width: {windowSize.width}px</div> <div>Window Height: {windowSize.height}px</div> </div> ); }; export default MyComponent;
In this example, we utilize the useEventListener hook to listen for ‘resize’ events on the window object. We update the windowSize state whenever a resize event occurs, capturing the updated width and height of the window. The component then renders the current window width and height.
Example 5: Handling mouse hover events
import React, { useState } from 'react'; import useEventListener from './useEventListener'; const MyComponent = () => { const [isHovered, setIsHovered] = useState(false); const handleMouseEnter = () => { setIsHovered(true); }; const handleMouseLeave = () => { setIsHovered(false); }; useEventListener('mouseenter', handleMouseEnter); useEventListener('mouseleave', handleMouseLeave); return ( <div> <div>{isHovered ? 'Mouse entered' : 'Mouse left'}</div> </div> ); }; export default MyComponent;
In this example, we use the useEventListener hook to listen for ‘mouseenter’ and ‘mouseleave’ events. We update the isHovered state when the mouse enters or leaves the component. The component renders a message based on the isHovered state, indicating whether the mouse has entered or left the component.
Example 6: Handling touch events
import React, { useState } from 'react'; import useEventListener from './useEventListener'; const MyComponent = () => { const [touchPosition, setTouchPosition] = useState({ x: 0, y: 0 }); const handleTouchMove = (event) => { const touch = event.touches[0]; setTouchPosition({ x: touch.clientX, y: touch.clientY }); }; useEventListener('touchmove', handleTouchMove); return ( <div> <div>Touch X: {touchPosition.x}px</div> <div>Touch Y: {touchPosition.y}px</div> </div> ); }; export default MyComponent;
In this example, we utilize the useEventListener hook to listen for ‘touchmove’ events. We extract the touch position from the event and update the touchPosition state accordingly. The component renders the current touch coordinates.
Conclusion
The useEventListener hook provides an elegant solution for handling DOM events in functional components in ReactJS. It simplifies event management, promotes reusability, and ensures consistent behavior across different components. By incorporating the useEventListener hook into your ReactJS projects, you can create interactive and responsive user interfaces with ease.
In this blog post, we explored the concept of useEventListener in ReactJS, its benefits, and how to use it in functional components. We provided code examples showcasing its usage in various scenarios, such as handling keypress events, scroll events, custom events, window resize events, mouse hover events, and touch events.
Remember to import the useEventListener hook into your project or create a custom implementation based on the provided code sample. Experiment with different events and explore its capabilities to create delightful user experiences.
Harness the power of useEventListener and unlock the full potential of event handling in your ReactJS applications. Happy coding!
Table of Contents
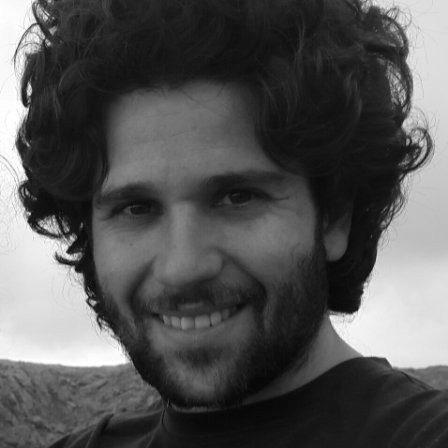
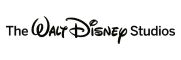