Simplifies data fetching using ReactJS useFetch hook
ReactJS has gained immense popularity among web developers due to its efficient and intuitive approach to building user interfaces. One crucial aspect of web development is data fetching, where applications retrieve data from external sources such as APIs. In this blog post, we will explore how to improve data fetching in ReactJS by building a custom data fetching hook called useFetch.
Importance of data fetching in web applications
Data fetching plays a crucial role in web applications, serving as the foundation for dynamic and interactive user experiences. It involves retrieving data from various sources such as APIs, databases, or third-party services to populate the application with relevant information. The importance of data fetching can be understood through the following key points:
- Real-time Data Updates: Web applications often rely on real-time or near real-time data updates to provide users with the latest information. Whether it’s social media feeds, live chat conversations, or stock market prices, data fetching enables applications to retrieve and display fresh data, keeping users engaged and informed.
- Seamless User Interactions: Many web applications require data fetching to enable seamless user interactions. For instance, when a user submits a form, the application may need to fetch data from a server to validate the input or update related information. Without efficient data fetching, the application’s responsiveness and interactivity can be compromised.
- Dynamic Content Rendering: Web applications often display dynamic content that changes based on user actions or external events. Data fetching allows applications to retrieve specific data subsets, filtered results, or paginated data to render dynamic content efficiently. By fetching only the necessary data, applications can optimize performance and enhance the user experience.
- Integration with External Services: Web applications frequently integrate with external services or APIs to access additional functionality or data sources. Data fetching enables applications to interact with these services, retrieve relevant data, and incorporate it seamlessly into the application’s workflow. This integration can range from fetching weather information to processing payments or integrating with social media platforms.
- Progressive Loading and Performance Optimization: Efficient data fetching techniques, such as lazy loading or pagination, can significantly improve application performance. Instead of loading all data upfront, applications can fetch data incrementally, reducing initial load times and improving perceived performance. This approach is particularly beneficial when dealing with large datasets or media files.
- Data Synchronization and Offline Support: In scenarios where web applications have offline capabilities or operate in unstable network conditions, data fetching becomes crucial for data synchronization. Applications can periodically fetch updates when the network connection is available, ensuring that the user’s data is up to date and consistent across different devices.
- Analytics and Business Intelligence: Data fetching is fundamental for collecting and analyzing data to derive meaningful insights. Web applications often fetch data from analytics platforms, tracking user behavior, and measuring key performance indicators. This data fuels business intelligence, allowing organizations to make informed decisions, improve products, and optimize their strategies.
In summary, data fetching is essential for web applications to provide real-time updates, seamless user interactions, dynamic content rendering, integration with external services, progressive loading, data synchronization, and analytics. By implementing efficient data fetching mechanisms, developers can create robust and user-friendly web applications that deliver a rich and responsive user experience.
UseFetch custom data fetching hook for ReactJS
The useFetch custom data fetching hook for ReactJS provides a streamlined and reusable solution for handling data fetching in React applications. Let’s explore its importance and benefits in-depth, along with some examples:
Simplified Data Fetching Logic:
The useFetch hook simplifies the process of fetching data by encapsulating the necessary logic within a reusable function. It abstracts away the complexities of making HTTP requests, handling loading states, errors, and parsing responses. This allows developers to focus on the core functionality of their components without getting lost in the details of data fetching.
For example, consider a scenario where you need to fetch a list of user data from an API endpoint:
import React from 'react'; import useFetch from './useFetch'; function UserList() { const { data, loading, error } useFetch('https://api.example.com/users'); if (loading) { return <p>Loading...</p>; } if (error) { return <p>Error: {error.message}</p>; } return ( <ul> {data.map((user) => ( <li key={user.id}>{user.name}</li> ))} </ul> ); }
With useFetch, the component is concise and focuses on rendering the user list based on the fetched data. The hook handles the data fetching process, loading states, and error handling, making the code more maintainable and readable.
Reusability and Code Organization:
By encapsulating data fetching logic in a custom hook, like useFetch, it becomes highly reusable across different components in the application. Instead of duplicating the same fetching logic in multiple places, you can simply import and use the useFetch hook wherever data fetching is required.
For instance, consider a scenario where you need to fetch data from different endpoints in different components:
import React from 'react'; import useFetch from './useFetch'; function ComponentA() { const { data, loading, error } = useFetch('https://api.example.com/dataA'); // ... } function ComponentB() { const { data, loading, error } = useFetch('https://api.example.com/dataB'); // ... }
In this example, both ComponentA and ComponentB utilize the same useFetch hook but fetch data from different endpoints. This promotes code organization, reduces duplication, and ensures consistency in data fetching across the application.
Handling Loading States and Errors:
Fetching data asynchronously often involves dealing with loading states and potential errors. The useFetch hook simplifies this process by automatically managing loading and error states, enabling you to provide appropriate feedback to the user.
import React from 'react'; import useFetch from './useFetch'; function UserProfile({userId }) { const { data, loading, error } = useFetch(`https://api.example.com/users/${userId}`); if (loading) { return <p>Loading...</p>; } if (error) { return <p>Error: {error.message}</p>; } return <p>Name: {data.name}</p>; }
In this example, the UserProfile component fetches user data based on a given userId. During the loading state, a “Loading…” message is displayed, and if an error occurs, an appropriate error message is rendered. Once the data is fetched successfully, the user’s name is displayed.
Customization and Flexibility:
The useFetch hook can be customized to suit different data fetching requirements. You can pass additional parameters such as headers, query parameters, or request methods to enhance the functionality of the hook.
import React from 'react'; import useFetch from './useFetch'; function Component() { const { data, loading, error } = useFetch('https://api.example.com/data', { headers: { Authorization: 'Bearer token' }, method: 'POST', body: JSON.stringify({ query: 'example' }), }); // ... }
In this example, the useFetch hook is customized to include headers for authorization, specify the request method as POST, and send a request body with a JSON payload. This flexibility allows you to handle various authentication mechanisms, send specific data to the server, or customize the request based on your application’s needs.
In conclusion, the useFetch custom data fetching hook for ReactJS simplifies data fetching, promotes code reuse, handles loading states and errors, and provides flexibility for customization. By leveraging the useFetch hook, you can enhance the efficiency and maintainability of your React applications when dealing with data fetching operations.
Understanding Data Fetching in ReactJS
Data fetching is a fundamental requirement for modern web applications. Traditionally, developers have used methods like the fetch API or libraries like Axios to fetch data in ReactJS. However, these approaches often involve boilerplate code and can lead to scattered logic throughout the application. Additionally, managing loading states, error handling, and reusability can become challenging with traditional methods.
Explaining the concept of Data Fetching
Data fetching is a fundamental concept in web development that involves retrieving data from external sources to populate and update the content of a web application. It enables applications to access and display information from various data providers such as APIs, databases, or third-party services.
In web applications, data fetching is essential for creating dynamic, interactive, and up-to-date user experiences. It allows applications to retrieve data in real-time, handle user interactions, and ensure that the displayed information remains accurate and relevant.
The process of data fetching typically involves sending requests to a data source, waiting for a response, and processing the received data. This can be achieved through various protocols and technologies, such as HTTP, GraphQL, or WebSocket.
Data fetching can be categorized into different types based on the purpose and characteristics of the data retrieval:
- Initial Data Fetching: When a web application loads for the first time, it often requires an initial fetch of data to populate the user interface with content. This can include fetching user profiles, product listings, or any other data needed to render the initial view of the application.
- Real-Time Updates: Many web applications rely on real-time updates to provide users with the latest information. This involves fetching data at regular intervals or establishing persistent connections with server-side technologies like WebSockets. Real-time data updates enable features like chat applications, live notifications, or stock market tickers.
- User-Initiated Data Fetching: Web applications often allow users to trigger data fetching operations by performing certain actions, such as submitting a search query, applying filters, or navigating to different sections. These user-initiated requests fetch data based on specific criteria or user input to provide personalized and relevant results.
- Pagination and Infinite Scrolling: When dealing with large datasets, it’s common to fetch data in smaller chunks using techniques like pagination or infinite scrolling. Pagination involves fetching a limited number of items at a time, allowing users to navigate through pages of data. Infinite scrolling loads additional data as the user scrolls down, dynamically expanding the content.
Data fetching is a critical aspect of modern web development, enabling applications to connect with external data sources and provide users with dynamic and up-to-date content. It involves handling request and response cycles, managing loading states, and processing the retrieved data to update the application’s state and user interface. With the right strategies and tools, data fetching can be optimized for performance, scalability, and a seamless user experience.
Challenges and limitations of traditional data fetching methods
Traditional data fetching methods in web development, such as using the fetch API or libraries like Axios, have certain challenges and limitations. Let’s explore some of these challenges:
- Boilerplate Code: Traditional data fetching methods often require writing repetitive boilerplate code for making HTTP requests, handling response parsing, and managing loading states and error handling. This can lead to code duplication and decreased code readability.
- Scattered Logic: Without a centralized approach, data fetching logic can be scattered throughout the application, making it harder to maintain and debug. The lack of a unified mechanism for data fetching can result in inconsistent error handling, loading indicators, and data update strategies.
- Lack of Abstraction: Traditional methods lack a convenient abstraction for encapsulating data fetching logic. As a result, developers often need to implement similar data fetching code in multiple components, leading to reduced reusability and increased development effort.
- State Management Challenges: Integrating traditional data fetching methods with state management libraries, like Redux, can introduce additional complexity. Managing loading states, error states, and data updates within the application state can be challenging, requiring extra code and coordination between different parts of the application.
- Error Handling: Traditional methods may require manual error handling and parsing of error responses from the server. This can introduce inconsistencies in error handling across different data fetching operations and make error handling more prone to human error.
- Lack of Built-in Features: Traditional data fetching methods often lack built-in features for common scenarios like handling request cancellation, debouncing or throttling requests, or managing caching. Developers need to implement these features manually, resulting in increased development effort and potential performance issues.
- Limited Support for Advanced Data Fetching Patterns: Some advanced data fetching patterns, such as parallel requests, pagination, or handling web socket connections, may require additional complexity and custom code when using traditional data fetching methods. These patterns may not be as easily achievable with basic fetch or Axios-based approaches.
- Testing Complexity: Testing components that rely on traditional data fetching methods can be challenging. Mocking network requests and responses or simulating different data scenarios may require additional setup and custom testing logic.
In summary, traditional data fetching methods often suffer from challenges such as boilerplate code, scattered logic, lack of abstraction, state management complexity, error handling issues, limited built-in features, difficulties in implementing advanced patterns, and testing complexity. These challenges can hinder development productivity, code maintainability, and the overall user experience.
Introducing useFetch: A custom data fetching hook
To overcome the limitations of traditional data fetching approaches, we can create a custom hook called useFetch. Custom hooks in ReactJS are reusable functions that encapsulate logic and can be shared across different components. The useFetch hook simplifies data fetching and provides a clean and concise way to handle loading states, errors, and data retrieval.
What is a custom hook in ReactJS and useFetch?
In ReactJS, a custom hook is a JavaScript function that follows a specific naming convention and leverages built-in React hooks to provide reusable functionality. Custom hooks allow developers to extract common stateful logic from components, making it easier to share and reuse that logic across multiple parts of an application.
Custom hooks are created by prefixing the function name with the word “use” and using one or more of the existing React hooks within the implementation. By adhering to this naming convention, React recognizes the function as a custom hook and allows it to leverage the power of hooks.
The useFetch custom hook is a specific example of a custom hook that is designed to simplify data fetching in ReactJS applications. It encapsulates the logic required for making HTTP requests, handling loading states, error handling, and parsing responses.
By using the useFetch hook, developers can abstract away the complexities of data fetching and provide a clean and reusable interface for fetching data in their React components. The useFetch hook typically returns an object containing the fetched data, loading state, and error state, which can then be used within the component to render the appropriate UI based on the current state of the data fetching process.
Here’s an example implementation of the useFetch custom hook:
import { useState, useEffect } from 'react'; const useFetch = (url) => { const [data, setData] = useState(null); const [loading, setLoading] = useState(true); const [error, setError] = useState(null); useEffect(() => { const fetchData = async () => { try { const response = await fetch(url); if (!response.ok) { throw new Error('Failed to fetch data'); } const json = await response.json(); setData(json); setLoading(false); } catch (error) { setError(error); setLoading(false); } }; fetchData(); }, [url]); return { data, loading, error }; }; export default useFetch;
In this example, the useFetch hook accepts a URL as a parameter and uses the fetch API to make the asynchronous request. It then updates the component’s state with the fetched data, loading state, and error state. The component using the useFetch hook can handle these states to render the appropriate UI elements.
By utilizing the useFetch custom hook in React components, developers can achieve cleaner and more modular code, improve reusability, and enhance the overall maintainability and efficiency of their data fetching operations.
Benefits of using useFetch for data fetching
Using the useFetch custom data fetching hook in your ReactJS applications provides several benefits. Here are five key advantages:
- Code Reusability: The useFetch hook promotes code reusability by encapsulating data fetching logic into a standalone function. Once created, the hook can be easily imported and used in multiple components across your application. This saves development time and effort, as you don’t need to duplicate the same data fetching code in different places.
- Simplified Data Fetching: With useFetch, the process of fetching data is simplified and abstracted away. The hook handles the underlying complexities of making HTTP requests, managing loading states, handling errors, and parsing responses. This allows you to focus on utilizing the fetched data within your components without getting bogged down in the details of the fetching process.
- Consistent Loading and Error Handling: The useFetch hook provides a consistent approach to handling loading states and errors across different components. It automatically manages the loading state, enabling you to display loading indicators or placeholders while the data is being fetched. It also handles error states, allowing you to show appropriate error messages or fallback UI in case of failed fetches. This consistency enhances the user experience by providing predictable feedback during the data fetching process.
- Enhanced Maintainability: By abstracting data fetching logic into a custom hook, useFetch improves the maintainability of your codebase. With a centralized data fetching mechanism, it becomes easier to manage and update the fetching logic in one place. This reduces the risk of inconsistencies or bugs that may arise from scattered or duplicated data fetching code throughout the application.
- Customization and Flexibility: The useFetch hook provides flexibility to customize the data fetching process based on your application’s specific requirements. You can pass additional parameters such as headers, query parameters, or request methods to the hook, enabling you to handle various authentication mechanisms, send specific data to the server, or customize the request based on your needs. This customization capability ensures that the useFetch hook can adapt to a wide range of data fetching scenarios.
In summary, using the useFetch custom data fetching hook offers benefits such as code reusability, simplified data fetching, consistent loading and error handling, enhanced maintainability, and customization flexibility. By leveraging these advantages, you can streamline your data fetching process, improve code quality, and deliver a more efficient and user-friendly ReactJS application.
Implementing useFetch in a ReactJS application
Let’s dive into the implementation of the useFetch hook in a ReactJS application. First, we set up a new ReactJS project and install the necessary dependencies. Then, we import the useFetch hook and use it within a component. We can pass the URL of the data source as a parameter to the useFetch hook, which will handle the fetching process and return the data.
Here’s a simplified example:
import React from 'react'; import useFetch from './useFetch'; function MyComponent() { const { data, loading, error } = useFetch('https://api.example.com/data'); if (loading) { return <p>Loading...</p>; } if (error) { return <p>Error: {error.message}</p>; } return <p>Data: {data}</p>; }
Setting up a new ReactJS project:
To create a new ReactJS project, you’ll need Node.js and npm (Node Package Manager) installed on your machine. Here’s how you can set up a new ReactJS project:
- Open your terminal or command prompt and navigate to the desired directory where you want to create your project.
- Run the following command to create a new ReactJS project using Create React App:
npx create-react-app my-app
Replace “my-app” with the desired name of your project.
- Once the command finishes executing, navigate into the newly created project directory.
cd my-app
Your ReactJS project is now set up and ready for use.
Installing the useFetch Hook:
The useFetch hook is a custom hook that needs to be installed in your ReactJS project as a separate dependency. Here’s how you can install it:
- In your terminal or command prompt, ensure you’re inside the root directory of your ReactJS project.
- Run the following command to install the useFetch hook from the package registry:
npm install use-http
The use-http package is a popular library that provides the useFetch hook for data fetching in ReactJS. It offers additional features and flexibility beyond basic data fetching.
Importing and Using the useFetch Hook:
After installing the useFetch hook, you can import and use it within your React components. Here’s an example of how to import and use the useFetch hook:
- Open the component file where you want to use the useFetch hook.
- At the top of the file, import the useFetch hook:
import useFetch from 'use-http';
c. Within your component function, use the useFetch hook to fetch data. Here’s an example:
import React from 'react'; import useFetch from 'use-http'; function MyComponent() { const { data, loading, error } = useFetch('https://api.example.com/data'); if (loading) { return <p>Loading...</p>; } if (error) { return <p>Error: {error.message}</p>; } return <p>Data: {data}</p>; } export default MyComponent;
In this example, the useFetch hook is used to fetch data from the ‘https://api.example.com/data‘ endpoint. The hook handles loading and error states, and the fetched data is rendered accordingly.
That’s it! You have successfully set up a new ReactJS project, installed the useFetch hook, and imported it into your component for data fetching. You can now continue building your ReactJS application and utilize the useFetch hook for efficient data retrieval.
Conclusion
In conclusion, the useFetch custom data fetching hook provides a powerful and efficient solution for handling data fetching in ReactJS applications. By encapsulating fetching logic into a reusable hook, we can reduce code duplication, improve maintainability, and simplify error handling and loading states. We encourage you to explore the useFetch hook and experiment with custom hooks to enhance your ReactJS development. Remember to refer to the provided resources for further learning and inspiration.
Happy coding!
Table of Contents
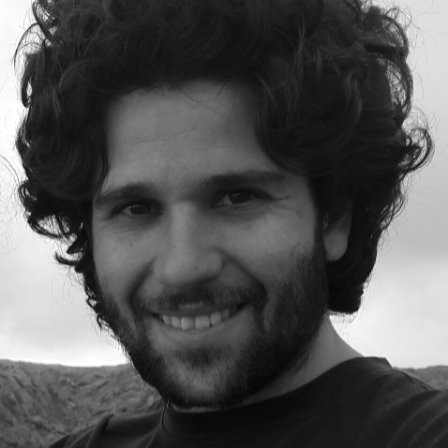
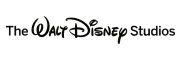